Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial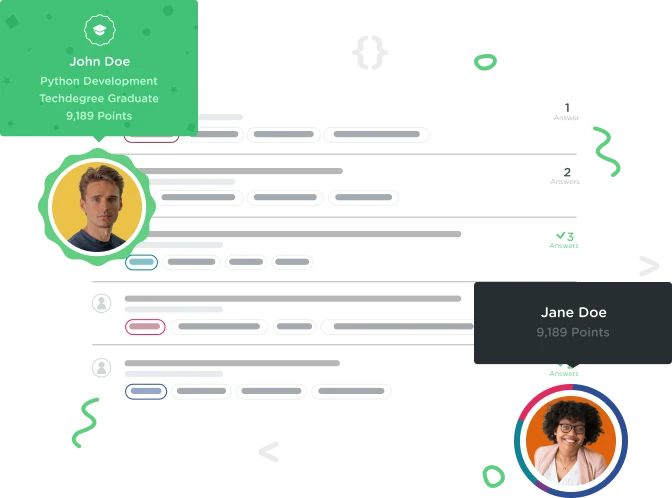

David Petrin
3,384 PointsOverriding Method Challenge Task Problem with Code
Not sure what I am doing wrong with this task here. Anyone have any suggestions?
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double) -> (Double, Double) {
return(width + points, height + points)
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
override func incrementBy(_ points: Double = 7.0) -> (Double, Double) {
return super.incrementBy(points)
}
4 Answers
William Li
Courses Plus Student 26,868 PointsHi, David Petrin
Aside from the missing closing bracket pointed out by Michael Hulet , there's another fatal problem with your code.
You modified the return type of the original incrementBy
method, which you shouldn't do; also, it's incorrect to add return type for method with no return value. The challenge is expecting the incrementBy
method be intact, and only override its implementation (slightly) in the RoundButton class.
Our RoundButton class should have its own implementation of the incrementBy method. Override the incrementBy method in the RoundButton class and default the points parameter to 7.0.
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double){
width += points
height += points
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
override func incrementBy(_ points: Double = 7.0) {
return super.incrementBy(points)
}
}
This should do it.
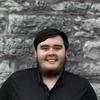
Michael Hulet
47,912 PointsJust glancing at it, I can see you're missing the closing bracket of the override
function. Try this:
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double) -> (Double, Double) {
return(width + points, height + points)
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
override func incrementBy(_ points: Double = 7.0) -> (Double, Double) {
return super.incrementBy(points)
//The following closing bracket didn't exist in your original code
}
}

David Petrin
3,384 PointsThanks, I did correct that. However, it gives me an error stating "You didn't add the 'incrementBy' method to the class 'RoundBotton' or click preview for compile errors. There are no compile errors. Is the code correct, but just not in the form (e.g. the function was modified to return a tuple) the challenge wanted?
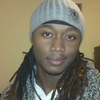
Kieran Tross
8,266 PointsSee my code below. It doesn't work
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double){
width += points
height += points
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
override func incrementBy(_ points: Double = 7.0) -> (Double) {
return super.incrementBy(points)
}
}
anyone know the answer ?
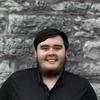
Michael Hulet
47,912 PointsIn the future, you should open your own question.
You're modifying the
return
type of yourincrementBy
function in yourRoundButton
subclass. Everyoverride
function needs to have the samereturn
type as the function it's overriding. Try this:
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double){
width += points
height += points
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
//Notice how this function declares no return type, and also never returns anything
override func incrementBy(_ points: Double = 7.0){
super.incrementBy(points)
}
}