Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial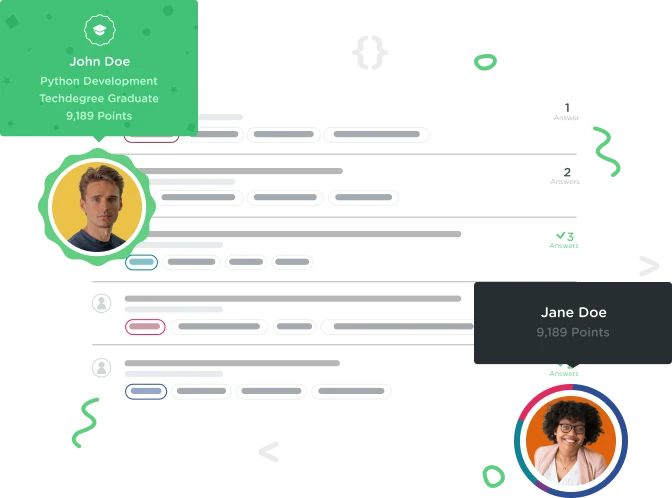

Shubham Batra
1,960 Pointsoverriding methods challenge
I don't know where did i go wrong. please tell me the correct answer to this challenge
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double){
width += points
height += points
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
override func incrementBy(points: Double = 7.0){
width += points
height += points
}
}
3 Answers

Omar Nunez
4,101 PointsYou Should init that RoundButton class, because you have a new var "cornerRadius"
init(width:Double,height:Double,cornerRadius:Double){
self.cornerRadius = cornerRadius
super.init(width: width, height: height)
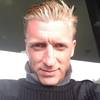
Rasmus Rønholt
Courses Plus Student 17,358 PointsHi Shubham
You can either omit the override keyword (called overloading) or insert an underscore before the argument name. Also you can take advantage of the base class function with the super keyword.
class RoundButton: Button {
var cornerRadius: Double = 5.0
override func incrementBy(_ points: Double = 7.0){
super.incrementBy(points)
}
// Or, with overloading...
func incrementBy(points: Double = 7.0){
super.incrementBy(points)
}
}

Omar Nunez
4,101 PointsAgree... It will be something like this
// Subclasses and Override
class RoundButton: Button {
var cornerRadius = 5.0
init(width:Double,height:Double,cornerRadius:Double){
self.cornerRadius = cornerRadius
super.init(width: width, height: height)
}
override func incrementBy(_ Points: Double = 7.0) {
height = height + Points
width = width + Points
}
}
Rasmus Rønholt
Courses Plus Student 17,358 PointsRasmus Rønholt
Courses Plus Student 17,358 PointsActually a subclass will automatically use its super's designated initialiser if none is provided - so in this case it's not necessary. It's true that if you wanted the cornerRadius to be an argument when instantiating the RoundButton then a new designated initialiser is in order...