Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial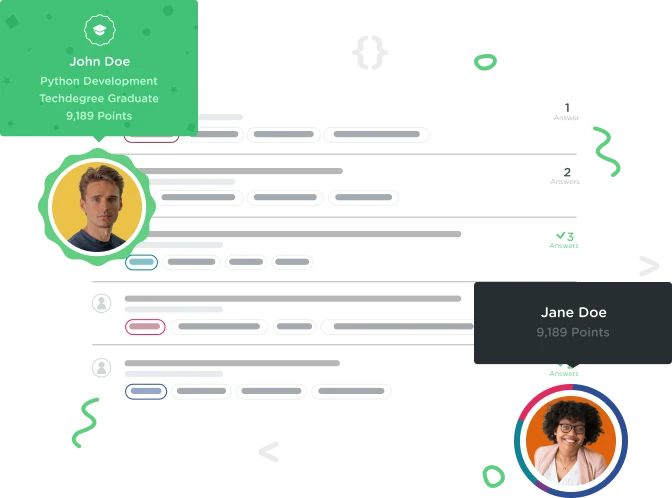

Simon Fitch
1,442 PointsOverriding Methods challenge - the code below works perfectly for me in Xcode 9.2 but keep getting 'code won't compile'
Any help on this one I would be most grateful - thanks :) Typical, just when I thought I was beginning to understand all this OOP as well!
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override init(firstName: String, lastName: String) {
super.init(firstName: firstName, lastName: lastName)
self.firstName = "Dr."
}
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
//someDoctor.fullName()
1 Answer

andren
28,558 PointsThe reason why it complains about the code not compiling is that firstName
and lastName
are declared with let
, which makes them constants. That means that it's invalid to change their contents after they are assigned a value, which they are when you call the super.init
method.
Are you sure you tried that exact code snipped in Xcode? In theory it should not work in any Swift compiler. If you declared firstName
and lastName
with var
like this:
class Person {
var firstName: String
var lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override init(firstName: String, lastName: String) {
super.init(firstName: firstName, lastName: lastName)
self.firstName = "Dr."
}
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
Then your code should compile, and in fact that code passes the challenge fine.
But one thing you should note for future reference is that challenges, as a general rule, are pretty strict about their instructions. If they ask you to do a specific thing in your code, then doing anything besides that thing will usually fail, even if the result of your code ends up being what the challenge asks for.
So while the code above (which is not really what the challenge asked you to write) does pass this particular challenge, it is rarely the case that challenges are so lenient. In most cases you have to follow the instructions to the letter. Practically any deviation from the template answer the challenge was programmed with tends to get your code invalidated.

Simon Fitch
1,442 PointsThanks very much for your answer Andren. Yes, I'm realising that now about the answer templates having to be pretty much in line with our answer code despite getting the correct result of the challenge. I still however got the code working fine in Xcode using let declarations instead of var ones which is odd? Anyway, thanks again for your reply.
Simon Fitch
1,442 PointsSimon Fitch
1,442 PointsOk, I get it (at last!) - you have to override the function rather than override the firstname property - still doesn't explain why it worked perfectly in Xcode though? Unless, the answer has to match exactly the template answer?