Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial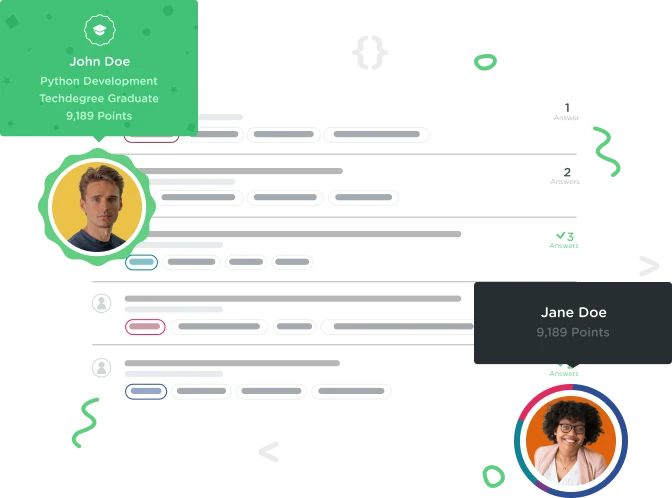

Michal Sverak
3,797 PointsOverriding Methods - code challenge
Hi can somebody please help me solve this? I am stuck on this code challenge and I don't really see what is wrong but I really want to solve it :)
2 Answers
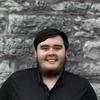
Michael Hulet
47,913 PointsTo override a method in Swift, you just redefine the method again, but you add the override
keyword in front of it, kind of like this:
//This can be done with structs or classes (and maybe enums, too?)
struct Parent{
func someMethod() -> Void{
print("This is logged from the parent class!")
}
}
struct Child: Parent{
//This is an example of overriding a method
override func someMethod() -> Void{
print("You successfully overrode someMethod!")
}
}

Allison Larson
3,177 PointsNeither of these work
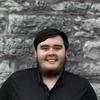
Michael Hulet
47,913 PointsI just noticed, but it's probably because I'm trying to not directly give the answer, so you can figure it out for yourself, but I overlooked one thing. Look at the constant name that it wants you to assign to. Are you using that properly?
Michal Sverak
3,797 PointsMichal Sverak
3,797 PointsThat is exactly what I have done but It doesn't seems to work for the challenge.
Michael Hulet
47,913 PointsMichael Hulet
47,913 PointsI think your problem is that the challenge asks for there to be a space between "Dr." and "Smith", but you didn't include that. Try this: