Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial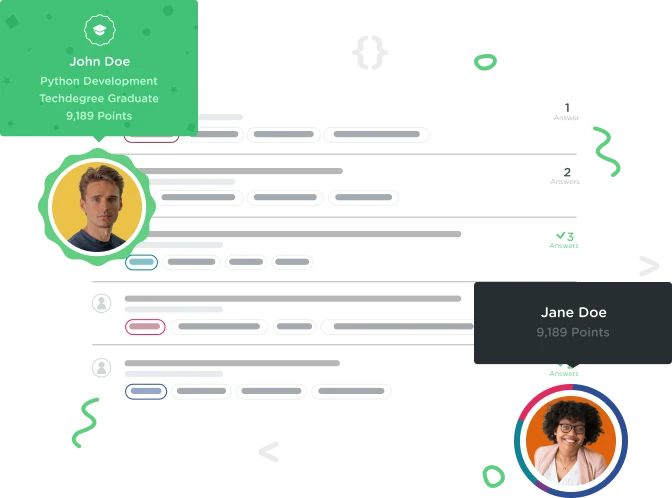

awake
6,126 PointsOverriding perform_create() and get_object() did not prevent me from changing the course on the api views.
from django.shortcuts import get_object_or_404
from rest_framework import generics
from . import models
from . import serializers
class ListCreateCourseView(generics.ListCreateAPIView):
queryset = models.Course.objects.all()
serializer_class = serializers.CourseSerializer
class RetrieveUpdateDestroyCourseView(generics.RetrieveUpdateDestroyAPIView):
queryset = models.Course.objects.all()
serializer_class = serializers.CourseSerializer
class ListCreateReviewView(generics.ListCreateAPIView):
queryset = models.Review.objects.all()
serializer_class = serializers.ReviewSerializer
def get_queryset(self):
return self.queryset.filter(course_id=self.kwargs.get('course_pk'))
def perform_create(self, serializer): # prevent changing the course that is being reviewed
course = get_object_or_404(
models.Course, pk=self.kwargs.get('course_pk'))
serializer.save(course=course)
class RetrieveUpdateDestroyReviewView(generics.RetrieveUpdateDestroyAPIView):
queryset = models.Review.objects.all()
serializer_class = serializers.ReviewSerializer
def get_object(self):
return get_object_or_404(
self.get_queryset(),
course_id=self.kwargs.get('course_pk'),
pk=self.kwargs.get('pk')
)
1 Answer
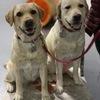
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi awake,
Can you verify that you're having the problem with both the courses/x/reviews/
AND the courses/x/reviews/y/
endpoints?
In the former case, you should be able to POST and the saved object should get a course id that corresponds with the URL regardless of what is chosen in the form. I was able to verify this behaviour.
In the latter case, we haven't written any code in the RetrieveUpdateDestroy view to replicate the perform_create
functionality that we have in the ListCreate view. Note that the get_object
method only provides the equivalent behaviour to the get_queryset
method from ListCreate.
If you want to implement this functionality, look at the perform_update
and perform_destroy()
methods.
Cheers,
Alex