Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial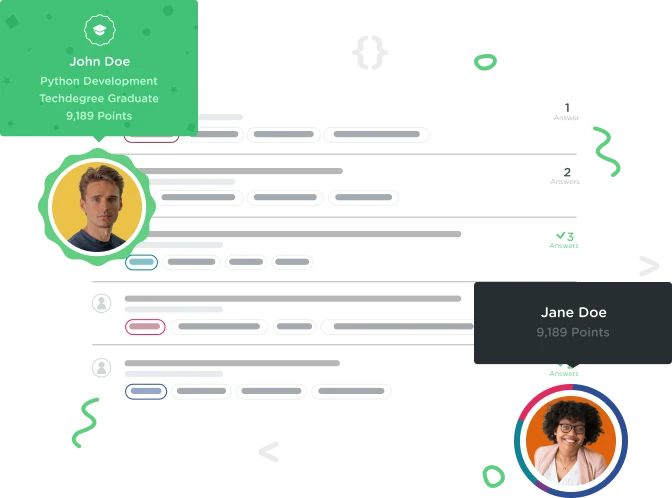

Kaiyang Lin
5,573 PointsOverwriting to_s function in a class
So what's interesting, I noticed when we are trying to overwrite the to_s method, is that we don't actually need to use instance variables, but can use local variables instead. Why is this the case, and where is the to_s getting these local variables from? Will there actually be an error if we had called the instance variables in the overwritten to_s method instead?
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Kaiyang,
I assume your questions are referring to name
and balance
within the to_s
method.
def to_s
"Name: #{name}, Balance: #{sprintf("%0.2f", balance)}"
end
You're correct that they are not instance variables because they do not have the @
in front of them. They could be local variables but then the program would produce an error since they're undefined. They're being accessed without having given them a value first.
The other thing that they could be are method calls. And in fact, the class does have a balance method which was written in the first part of this video. So balance
is actually calling the balance method. The returned balance is then what is inserted into that part of the string.
What about name
though? Looking through the class there isn't a name
method. However, at the top you have attr_reader :name
That code automatically creates the following method:
def name
@name
end
This is simply a getter method which returns the instance variable @name
So in the to_s method, name
is actually a call to that method. The returned name is what gets inserted into the string.
Your last question, "Will there actually be an error if we had called the instance variables in the overwritten to_s method instead?"
No error. You could still access the name instance variable directly and not go through the getter method.
This works too:
def to_s
"Name: #{@name}, Balance: #{sprintf("%0.2f", balance)}"
end
We can't do it with balance though because there is no instance variable @balance
. That one has to remain a method call.
So to summarize, you could always access instance variables directly from within the class but attr_reader
makes it convenient to access them through the getter methods by leaving off the @
symbol.
Kaiyang Lin
5,573 PointsKaiyang Lin
5,573 PointsI see. So its the method being called within the class.
Thanks for the clear explanation, Jason!
PoJung Chen
5,856 PointsPoJung Chen
5,856 PointsVery clearly explanation! Thanks!