Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial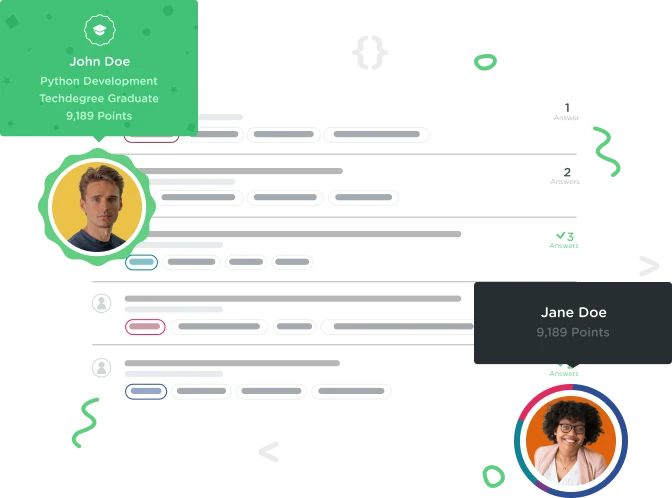

SC3 Rocks
33,474 PointsPacking Challenge...
Hi, I'm having trouble figuring out what this challenge wants me to do - I thought I had a better idea as to what it was asking, but I guess I don't - I would appreciate any help that someone could provide - Below is the code that I tried to use for this challenge - Thank you in advance...
"Let's play with the *args pattern. Create a function named multiply that takes any number of arguments. Return the product (multiplied value) of all of the supplied arguments. The type of argument shouldn't matter."
def multiply("Melanie", "Amil", "Jackllin", "Kelvar"):
for m in multiply:
total = total * multiply
return total
5 Answers

Umesh Ravji
42,386 PointsOkay, this problem has two aspects that you need to understand. The first is being able to deal with any number of arguments and then being able to iterate over the arguments in order to do something with them. I'm going to use a different, but similar, function to demonstrate these two points.
1. Accepting any number of arguments.
def add_all(*numbers):
pass
print(add_all(1, 2, 3, 4, 5)) # None
print(add_all(1, 2, 3, 4, 5, 6, 7, 8,9)) # None
The add_all
function is able to accept any number of arguments. The asterisk in front of the numbers
parameter packs up all of the arguments into a single tuple named numbers
that can be iterated over using a for loop.
2. Iterating over the arguments.
def add_all(*numbers):
total = 0
for number in numbers:
total += number
return total
print(add_all(1, 2, 3, 4, 5)) # 15
print(add_all(1, 2, 3, 4, 5, 6, 7, 8,9)) # 45
Since you have all of the arguments packed together into a tuple named numbers
, iterating over them using a for loop and doing something with each number is just like any other for loop.

SC3 Rocks
33,474 PointsOkay, so, I tried it again, and this is what I came up with:
def multiply(18, 34, 28, 37):
total = 1
for total = total * m
return total

SC3 Rocks
33,474 PointsOkay, does anyone know of any free tutoring in Python that I can look into, because I'm really not getting this - Maybe it's my dyslexia, but my mind isn't wrapping around any of this very well - Sorry, if I'm a little slow with all this, but I do appreciate your efforts to at least try and help me understand... P
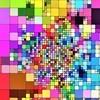
james south
Front End Web Development Techdegree Graduate 33,271 Pointsi think it means any type of number - float or int. so supply some numbers as arguments and return their product.

Umesh Ravji
42,386 PointsHey SC3 Rocks, in a nutshell the question wants you to create a function that is able to take any number of arguments and multiply them all together. So calling multiply(3,4)
and multiply(3,4,5,6,7,8)
should work. You are in the right direction for sure.
def multiply(*args):
total = 1
# Multiply all the values with the total using a loop... for x in args
return total

SC3 Rocks
33,474 PointsNot entirely sure I understand what you're saying, Umesh, but I'll give it a try...