Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial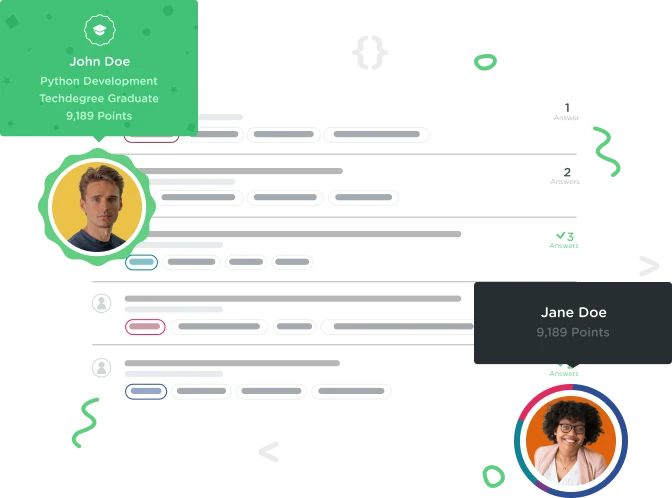

evanritscher
10,209 PointsPage just displays [object Object] five times.
Hello,
Bellow is my attempt at this challenge. When I look in the console, I see all the data in the array though not in the prettiest form. Worst though, the page itself just displays [object Object] five times. Where have I gone wrong?
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
var listHTML ='<p>';
for (var prop in students){
console.log(students[prop]);
listHTML+= students[prop];
}
listHTML+='</p>'
document.write(listHTML);
2 Answers
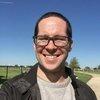
Kevin Foytik
14,553 PointsIt looks like you're looping through the objects themselves rather than the properties. In other words, the "prop" in your loop is actually referring to everything within the {}, i.e. the objects. So, if you're trying to log names, for instance, you should use:
for (var object in students) {
console.log(object.name);
}

Tonye Jack
Full Stack JavaScript Techdegree Student 12,469 PointsA Better way
var message;
var students = [
{name:'Tome',
Track:'iOS',
Achievements: 4,
Points: 233
},
{name:'Luke',
Track:'Java',
Achievements: 7,
Points: 433
},
{name:'Tonye',
Track:'JavaScript',
Achievements: 10,
Points: 2500
},
{name:'Jane',
Track:'FrontEnd Developement',
Achievements: 3,
Points: 577
}
];
function print(message){
var x = document.getElementById('output');
x.innerHTML = message;
}
for(var i= 0; i < students.length; ++i){
message += ('<hr>');
message +=('<p><b>Student: </b>' + students[i].name + '</p>');
message += ('<p>Track: ' + students[i].Track + '</p>' );
message +=('<p>Achievements: ' + students[i].Achievements + '</p>');
message +=('<p>Points: ' + students[i].Points + '</p>');
message +=('<br>');
}
print(message)
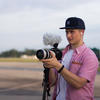
Christopher Johnson
12,829 PointsThis doesn't work. By including a
message = ('<hr>');
at the beginning of the loop, you reset the message each time the loop loops (that's what '=' does compared to adding to the current set value '+='. You can simply add
print('<hr>' + message);
at the end of the script.
Full code:
/*
2 steps:
1st: create data structure to hold information on group(5) of students (array, objects represent 1 student:
name,
track (treehouse),
achievements (number value),
points (# earned).
2nd: access each students objects and print each key and property
Report should look as:
<h2> Student: X </h2>
<p>track: X</p>
<p>Points: X</p>
<p>Achievements: X</p>
...
Loop through each element of the array, build up a message that contains all the student records.
*/
var message = '';
var students = [
{
name: "Clark Kent",
track: "Full-stack",
achievements: 99,
points: 99
},
{
name: "Bruce Wayne",
track: "Full-stack",
achievements: 100,
points: 100
},
{
name: "Barry Allen",
track: "Front-end",
achievements: 80,
points: 80
},
{
name: "Bruce Banner",
track: "C++",
achievements: 10,
points: 10
},
{
name: "Bruce Genner",
track: "No one knows",
achievements: 0,
points: 0
}
]
function print(message){
var outputDiv = document.getElementById("output");
outputDiv.innerHTML = message;
}
for (var i = 0; i < students.length; i++) {
message += '<p><b>Student: ' + students[i].name + '</b></p>';
message += '<p>Track: ' + students[i].track + '</p>';
message += '<p>Achievements: ' + students[i].achievements + '</p>';
message += '<p>Points: ' + students[i].points + '</p>';
message += '<br>';
}
print('<hr>' + message);
Also, it's a good habit to use spaces in the same way throughout the document.

Tonye Jack
Full Stack JavaScript Techdegree Student 12,469 PointsThe message = ('<hr>'); was clearly a mistake. Thanks