Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial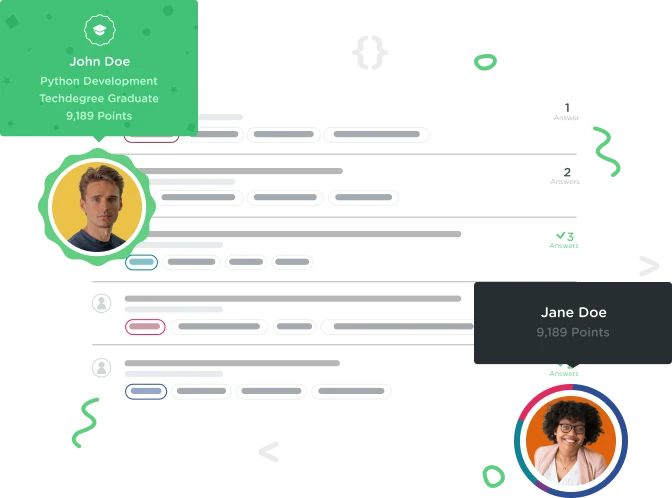
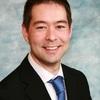
Mark Chesney
11,747 Pointsparameter specification for np.repeat()
Why does this code work?
import numpy as np
threes = np.repeat(3, 3)
But these all throw the same error?
threes = np.repeat(3, 3, dtype=np.uint8) # OK I expected this to fail
threes = np.repeat([3, 3], dtype=np.uint8)
threes = np.repeat((3, 3), dtype=np.uint8)
I ran ?threes
and still didn't figure it out.
Thanks!
1 Answer
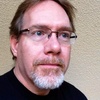
Chris Freeman
Treehouse Moderator 68,423 PointsThe method np.repeat
does not take dtype
as an argument. From help(np.repeat)
:
repeat(a, repeats, axis=None)
Repeat elements of an array.
Parameters
----------
a : array_like
Input array.
repeats : int or array of ints
The number of repetitions for each element. `repeats` is broadcasted
to fit the shape of the given axis.
axis : int, optional
The axis along which to repeat values. By default, use the
flattened input array, and return a flat output array.
Returns
-------
repeated_array : ndarray
Output array which has the same shape as `a`, except along
the given axis.
So, your first 3 is being treated as an array-like object. Instead, insert the array type you wish:
>>> import numpy as np
>>> np.repeat(3, 3)
array([3, 3, 3])
>>> np.repeat([3], 3)
array([3, 3, 3])
>>> x = np.array(3, dtype=np.uint8)
>>> np.repeat(x, 3)
array([3, 3, 3], dtype=uint8)
Post back if you need more help. Good luck!!
Mark Chesney
11,747 PointsMark Chesney
11,747 PointsThank you so much Chris! Yes, in hindsight, the CLI returns this error:
I wonder how I'd missed that. My advice to everyone is to try to get plenty of sleep before coding :)