Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial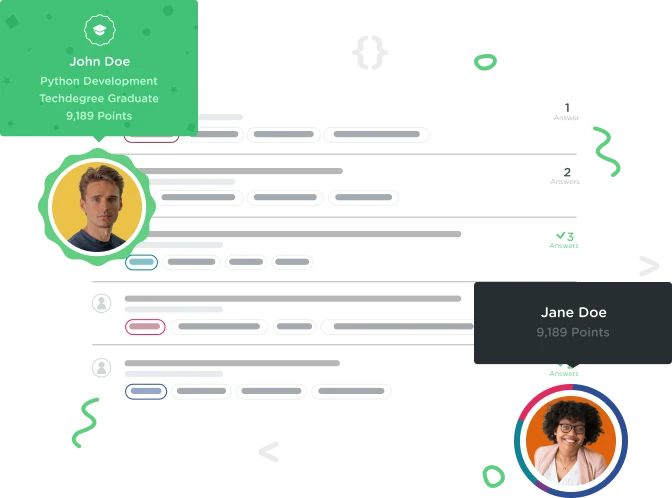

swiftfun
4,039 PointsParameters in a method
Two questions:
1- Is any function inside a struct necessarily called a Method? Do we no longer call them Functions? Please clarify when do we call it Method and when do we call them Functions
2- Do we ever use parameters in a func which is inside a struct? Since the struct itself is a blueprint and later on we will use it to input (in this case first and last name) will we ever need to use parameters in a function, or will it always be empty () ?
struct Person {
let firstName: String
let lastName: String
func getFullName() -> String { // Are func parameters always empty when inside a struct?
return "\(firstName) \(lastName)"
}
}
let aPerson = Person(firstName: "John", lastName: "Doe")
let fullName = aPerson.getFullName()
4 Answers
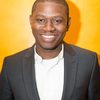
Welby Obeng
20,340 PointsAny function associated with class or structure is called a method. If itβs not associated with class or structure itβs called a function. A function is a piece of code that is called by name, whereas a method is a piece of code that is called by name that is associated with an object (instance of class or structure). It can be passed data to operate on (ie. the parameters) and can optionally return data (the return value).
You can provide method parameters inside a class or structure.

swiftfun
4,039 PointsThank you Welby,
Why would you include method parameters in a struct instead of using stored properties in the struct?
I see you could include "age" in the previous example as a method parameter, wouldn't it be more convenient to include that in a struct? Just trying to think of an example in a functioning app, still learning the theory and not quite sure about how everything will work out in an app. When would you use structs or functions?
struct Person {
let firstName: String
let lastName: String
func getFullName(age: Int) -> String {
return "\(firstName) \(lastName) is \(age) years old."
}
}
let aPerson = Person(firstName: "John", lastName: "Doe")
let fullName = aPerson.getFullName(3)
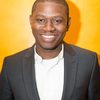
Welby Obeng
20,340 PointsGood question, let's say someone wants a full name with spacing and not with spacing...
if getFullName method takes in a bool called WithSpacing, that can be set true or false, I can have an if statement that check if WithSpacing is true and return fullname with spacing, if it's false I can return full name without spacing...
You wouldn't want to set WithSpacing variable as a property of the struct object because it's really pretaining to the getFullName method

swiftfun
4,039 PointsMakes sense. Hopefully you or someone else could provide more insight and examples