Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial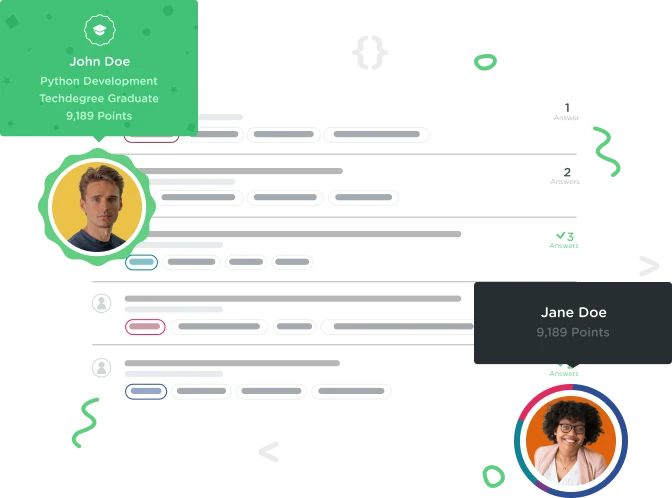

Tim Wang
9,068 Pointsparameters in the callback functions fed into asyncHandler
Why is it that, in some routes, callback functions fed into asyncHandler have only two parameters (req, res), and in other routes, those callback functions have three parameters (req, res, next)?
Since the callback function inside the asyncHandler takes 3 parameters, doesn't it make more sense that cb functions should all have 3 parameters?
1 Answer
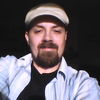
Robert Manolis
Treehouse Guest TeacherHey Tim Wang, great question. The callbacks in the route handlers don't have to have that third parameter unless you're going to be calling the next() method specifically and directly inside of the route handlers' callback.
And the route handlers' callbacks not having the next parameter shouldn't impede the asynchHandler helper function from utilizing its own next() method.
For an example, here's a version of that asyncHanler helper function that I have in a project:
/* Async try/catch */
exports.asyncHandler = (cb) => {
return async (req,res,next) => {
try {
await cb(req,res,next);
} catch(err) {
next(err);
}
}
}
For the above to work, we just need to pass a callback function to it. The next() method called in the catch block is a reference to the first next parameter in the return statement. Remember, with the way this asyncHandler is being used, it is itself a callback function to another method like .get or .post, which passes the req and res to it. And Express sets it up so it has access to that next() method as the third parameter if its present. But that first next parameter in the example should impact the second next parameter. If the first one was missing, then you wouldn't be able to call the next() method in the catch block above. And if the second next parameter was missing, then maybe you wouldn't be able to call the next() method in the callback.
To test this out and get a better feel and understanding for it, try changing the name of the first next parameter, and then give the next method in the catch block the same name, and see if it all still works. Hope that helps.