Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial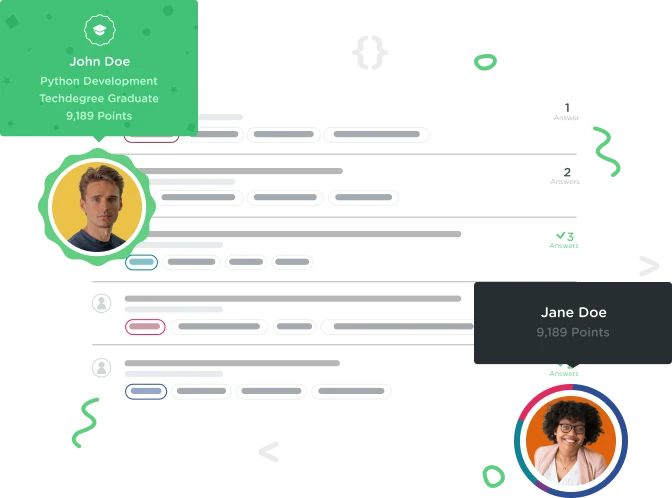
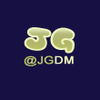
Jonathan Grieve
Treehouse Moderator 91,253 PointsParcelable weather data not displaying
There's no errors in the files and logcat seems to be functioning okay. But when I run the app in the emulator no data is displayed and I get message instead.
There must be something I've missed, I just can't figure out what. Here's my latest Repo. https://github.com/jg-digital-media/Stormy-Android-Lists-and-Adapters-
package teamtreehouse.com.stormy.weather;
import android.os.Parcel;
import android.os.Parcelable;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.TimeZone;
/**
* Created by Jonnie on 14/05/2017.
*/
public class Day implements Parcelable {
private long time;
private String summary;
private double temperatureMax;
private String icon;
private String timezone;
public long getTime() {
return time;
}
public void setTime(long time) {
this.time = time;
}
public String getSummary() {
return summary;
}
public void setSummary(String summary) {
this.summary = summary;
}
public int getTemperatureMax() {
return (int) Math.round(temperatureMax);
}
public void setTemperatureMax(double temperatureMax) {
this.temperatureMax = temperatureMax;
}
public String getIcon() {
return icon;
}
public void setIcon(String icon) {
this.icon = icon;
}
public String getTimezone() {
return timezone;
}
public void setTimezone(String timezone) {
this.timezone = timezone;
}
public int getIconId() {
return Forecast.getIconId(icon);
}
public String getDayOfTheWeek() {
SimpleDateFormat formatter = new SimpleDateFormat("EEEE");
formatter.setTimeZone(TimeZone.getTimeZone(timezone));
Date dateTime = new Date(time * 1000);
return formatter.format(dateTime);
}
@Override
public int describeContents() {
return 0;
}
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeLong(time);
dest.writeString(summary);
dest.writeDouble(temperatureMax);
dest.writeString(icon);
dest.writeString(timezone);
}
//default constructor for Day class
public Day() {
} ;
//read parcelable data
private Day(Parcel in) {
//order of data is important - read data in order it was written
time = in.readLong();
summary = in.readString();
temperatureMax = in.readDouble();
icon = in.readString();
timezone = in.readString();
}
//creator field/object
public static final Creator<Day> CREATOR = new Creator<Day>() {
@Override
public Day createFromParcel(Parcel source) {
return new Day(source);
}
@Override
public Day[] newArray(int size) {
return new Day[size];
}
};
}
package teamtreehouse.com.stormy.ui;
import android.app.Activity;
import android.app.ListActivity;
import android.content.Intent;
import android.os.Bundle;
import android.os.Parcelable;
import android.widget.ArrayAdapter;
import java.util.Arrays;
import teamtreehouse.com.stormy.R;
import teamtreehouse.com.stormy.adapter.DayAdapter;
import teamtreehouse.com.stormy.weather.Day;
public class DailyForecastActivity extends ListActivity {
private Day[] mDays;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_daily_forecast);
Intent intent = getIntent();
//parcelable array
Parcelable[] parcelables = intent.getParcelableArrayExtra(MainActivity.DAILY_FORECAST);
mDays = Arrays.copyOf(parcelables, parcelables.length, Day[].class);
DayAdapter adapter = new DayAdapter(this, mDays);
}
}
1 Answer

Sana Khateeb
3,979 PointsI had this exact same error and it took me forever to figure out, turns out in your DailyForecastActivity.java you still need to set the list adapter. Right after you create the DayAdapter adapter you need to have this line:
setListAdapter(adapter)
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsI still have such a lot to learn but thanks for this. I must have forgotten to add this when following along :)
Fahad Mutair
10,359 PointsFahad Mutair
10,359 Pointsthanks for saving my day