Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial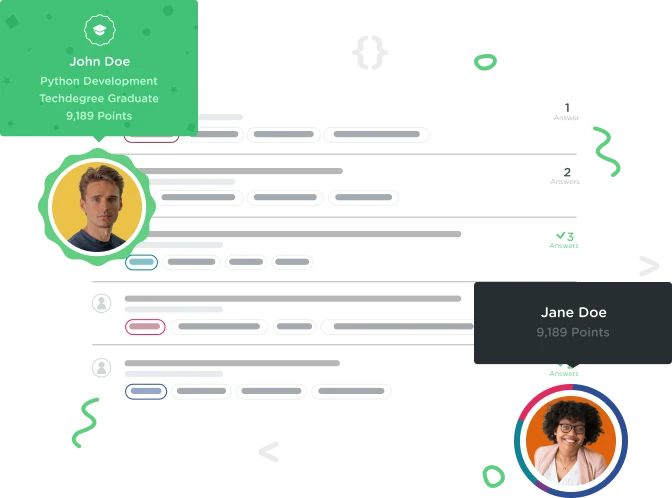

William J. Terrell
17,403 PointsParse error: syntax error, unexpected '->'... in products.php on line 13 [SOLVED]
I've been having a lot of trouble throughout this course with error messages that I just can't figure out. This one in particular reads:
Parse error: syntax error, unexpected '->' (T_OBJECT_OPERATOR) in C:\xampp\htdocs\treehouse\PDO_tutorial\inc\products.php on line 13
Line 13 of products.php is as follows:
$results = db->query("
Does it not recognize the -> operator?
I've included the entirety of products.php below.
<?php
/*
* Returns the four most recent products, using the order of the elements in the array
* @return array a list of the last four products in the array;
the most recent product is the last one in the array
*/
function get_products_recent() {
require(ROOT_PATH . "inc/database.php");
try {
/* This is Line 13 */$results = db->query("
SELECT name, price, img, sku, paypal
FROM products
ORDER BY sku DESC
LIMIT 4
");
} catch (Exception $e) {
echo "Data could not be retrieved.";
exit;
}
$recent = $results->fetchAll(PDO::FETCH_ASSOC);
$recent = array_reverse($recent);
return $recent;
}
/*
* Looks for a search term in the product names
* @param string $s the search term
* @return array a list of the products that contain the search term in their name
*/
function get_products_search($s) {
require(ROOT_PATH . "inc/database.php");
try {
$results = db->prepare("
SELECT name, price, img, sku, paypal
FROM products
WHERE name LIKE ?
ORDER BY sku ASC
");
$results->bindValue(1, "%" . $s . "%");
$results->execute();
} catch (Exception $e) {
echo "Data could not be retrieved.";
exit;
}
$matches = $results->fetchAll(PDO::FETCH_ASSOC);
return $matches;
}
/*
* Counts the total number of products
* @return int the total number of products
*/
function get_products_count() {
require(ROOT_PATH . "/inc/database.php");
try {
$results = db->query("
SELECT COUNT(sku)
FROM products
");
} catch (Exception $e) {
echo "Data could not be retrieved.";
exit;
}
return intval($results->fetchColumn(0));
}
/*
* Returns a specified subset of products, based on the values received,
* using the order of the elements in the array .
* @param int the position of the first product in the requested subset
* @param int the position of the last product in the requested subset
* @return array the list of products that correspond to the start and end positions
*/
function get_products_subset($positionStart, $positionEnd) {
require(ROOT_PATH . "inc/database.php");
$offset = $positionStart - 1;
$rows = $positionEnd - $positionStart + 1;
try {
$results = db->prepare("
SELECT name, price, img, sku, paypal
FROM products
ORDER BY sku ASC
LIMIT ?, ?
");
$results->bindParam(1, $offset, PDO::PARAM_INT);
$results->bindParam(2, $rows, PDO::PARAM_INT);
$results->execute();
} catch (Exception $e) {
echo "The data could not be retrieved";
exit;
}
$subset = $results->fetchAll(PDO::FETCH_ASSOC);
return $subset;
}
/*
* Returns the full list of products. This function contains the full list of products,
* and the other model functions first call this function.
* @return array the full list of products
*/
function get_products_all() { /* Nothing uses this function anymore, so the array has been removed. */
require(ROOT_PATH . "inc/database.php");
try {
$results = $db -> query("SELECT name, price, img, sku, paypal FROM products ORDER BY sku ASC");
} catch ( Exception $e ) {
echo "Query could not be processed.";
exit;
}
$products = ($results->fetchAll(PDO::FETCH_ASSOC));
return $products;
}
/*
* Returns an array of product information for the product that matches the SKU
*Returns a boolean of "False" if no product matches the SKU
@param int $sku the sku
@return mixed array list of product information for the one matching product
boolean False if no product matches
*/
function get_product_single($sku) {
require(ROOT_PATH . "inc/database.php");
try {
$results = $db->prepare("SELECT name, price, img, sku, paypal FROM products WHERE sku = ?");
$results->bindParam(1, $sku);
$results->execute();
} catch (Exception $e) {
echo "Query could not be processed.";
exit;
}
$product = $results->fetch(PDO::FETCH_ASSOC);
if ($product === false) {
return $product;
}
$product["sizes"] = array();
try {
$results = $db->prepare("
SELECT size
FROM product_sizes ps
INNER JOIN sizes s
ON ps.size_id = s.id
WHERE product_sku = ?
ORDER BY `order`");
$results->bindParam(1, $sku);
$results->execute();
} catch (Exception $e) {
echo "Data could not be retrieved.";
exit;
}
while ($row = $results->fetch(PDO::FETCH_ASSOC)) {
$produce["sizes"][] = $row["size"];
}
return $product;
}
?>
Any insight would be appreciated.
Thanks!
[SOLVED]
Never mind, I was just missing the $ sign ^^;
But I am still having trouble with this problem if anyone felt obliged to assist :)
1 Answer

Aamir Mirza
25,077 PointsMy PDO skills are a bit rusty but I hope this works :)
Try typing in $db->query instead of db->query.
Aamir Mirza
25,077 PointsAamir Mirza
25,077 PointsLikewise for all the other objects. $db->prepare in place of db->prepare
William J. Terrell
17,403 PointsWilliam J. Terrell
17,403 PointsSorry, I caught that, but thanks! :)