Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial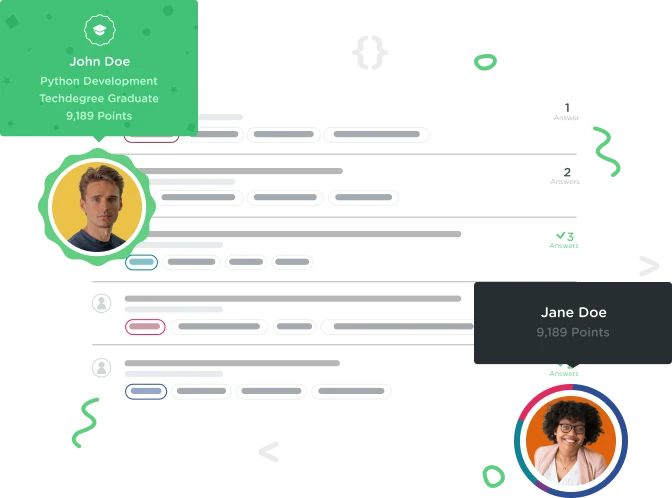
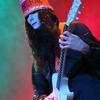
Zachary Baker
Courses Plus Student 11,504 PointsParse error: syntax error, unexpected '$results' (T_VARIABLE) in /home/treehouse/workspace/inc/functions.php on line 47
I have a Parse error on line 47 of my function.php file. I followed the video and I tried to fix the problem, but to no avail, I get the same thing. What do I do from here?
3 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Zachary,
I think it would be line 46 you have your else
statement. There's a special character at the end of that line right after the left curly brace that you should try deleting. I think it's the delete character, code 0x7f
That might be causing your syntax error. You might not be able to see it in workspaces but if you paste that part of your code into a text editor then you should be able to see it. I see it as a box in both notepad++ and sublime text.
See the comment in the code
if (is_integer($limit)) {
$results = $db->prepare($sql. " LIMIT ? OFFSET ?");
$results->bindParam(1,$limit,PDO::PARAM_INT);
$results->bindParam(2,$offset,PDO::PARAM_INT);
} else { // this is line 46. There's a special character here after the left curly brace that you should delete.
$results = $db->prepare($sql);
}
$results->execute();
If that doesn't solve your problem you can post a snapshot of your workspace and that will make it easier to troubleshoot.
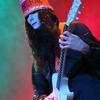
Zachary Baker
Courses Plus Student 11,504 Points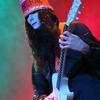
Zachary Baker
Courses Plus Student 11,504 PointsThis is the snapshot of my workspace.

Jason Anello
Courses Plus Student 94,610 PointsDid you try deleting the character that I mentioned in my answer?
I forked your workspace and deleted that character and it seems to be rendering fine now.
You have to go to the end of line 46, and then hit the backspace key one time. It won't delete the curly brace but it will delete that special character you have.
I'm not sure how you got it in there though.
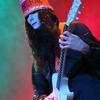
Zachary Baker
Courses Plus Student 11,504 PointsThanks Jason! That solved everything and everything is now running. I really appreciate the help!
Zachary Baker
Courses Plus Student 11,504 PointsZachary Baker
Courses Plus Student 11,504 Points