Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial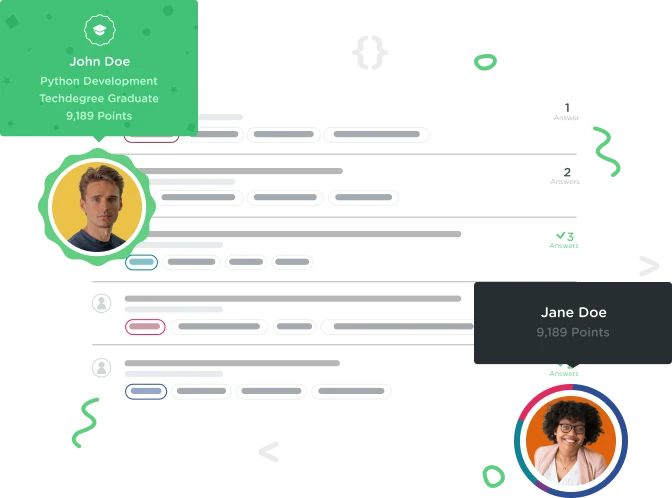

Taylor Skidmore
1,096 PointsParsing Data Returned in JSON: "JSONException: Unterminated string at character 5792"
Following along with the videos, I get the error in the title. After doing some research I figured out that the probably cause is getContentLength()
is not returning the correct value, thus overflowing the read buffer. From the docs:
By default, this implementation of HttpURLConnection requests that servers use gzip
compression. Since getContentLength() returns the number of bytes transmitted, you
cannot use that method to predict how many bytes can be read from getInputStream().
Instead, read that stream until it is exhausted: when read() returns -1. Gzip
compression can be disabled by setting the acceptable encodings in the request
header:
So, I figured that with gZip compression, the values were getting a little messed up. I went to the URL and saved the raw JSON, then got the character count for that: 5792. Being the same length as the array, there should be no overflow, yet the error show the JSON being cut off in the wrong place. I went ahead and made a paste of the full error, and you can clearly see the mismatch.
I even downloaded the project files and tried it there but still with no luck. Is this an error in the code or am I doing something completely wrong?
2 Answers

Ben Jakuben
Treehouse TeacherThis is an error! We discussed this in the Forum a while back and have linked to it as a "Known Issue" in the Teacher Notes on the video page. Check out the solution (and discussion) in that other thread. I will loop back and update the project at some point, too.

Michael Dvorscak
7,003 PointsI had the same issue, and after some investigation the problem seemed to be coming from the HTML escape sequence &
(the '&' symbol)
My fix was to instead use OkHttp ; like we previously used in Stormy (Weather App). Here is my updated doInBackground function
//at the top of main activity
private String blogFeedURL = "http://blog.teamtreehouse.com/api/get_recent_summary/?count=" + NUMBER_OF_POSTS;
protected OkHttpClient mClient = new OkHttpClient();
protected Request mRequest = new Request.Builder()
.url(blogFeedURL)
.build();
protected JSONObject doInBackground(Object[] params) {
JSONObject jsonResponse = null;
try {
Call call = mClient.newCall(mRequest);
//We can use the blocking version since we aren't on the main thread
Response response = call.execute();
if (response.isSuccessful()) {
String jsonData = response.body().string();
jsonResponse = new JSONObject(jsonData);
} else {
Log.d(TAG, "There with the connection");
}
} catch (MalformedURLException e) {
Log.e(TAG, "Exception caught: ", e);
} catch (IOException e){
Log.e(TAG, "Exception caught: ", e);
} catch (JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
return jsonResponse;
}
and of course adding: compile 'com.squareup.okhttp:okhttp:2.2.0' to the Gradle dependencies
This was a much cleaner/easier solution.
Taylor Skidmore
1,096 PointsTaylor Skidmore
1,096 PointsHmm,
I changed the Reader reader deceleration to a type of InputStreamReader and everything seemed to be working more consistently.
After changing that type deceleration back to Reader, the code still works. What exactly is happening to produce this error?
Also, I am using the official treehouse blog, and I was still running into the issue.
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherI saw this a while back with the Treehouse Blog, and the solution I linked to above fixed it at that time. Have you tried replacing the whole
doInBackground
method with this version?:Taylor Skidmore
1,096 PointsTaylor Skidmore
1,096 PointsYes, I just did, and now it's working on each attempt without a hitch.
I was just curious as to why it was throwing that error in the first place, sorry if I wasn't terribly clear. Everything is working perfectly fine now :)
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherGotcha - I was never able to figure out exactly what the problem is. It appears to be an issue on the Treehouse Blog when certain special characters are used in titles. At that point I don't know if it's an encoding issue or it somehow breaks the content-length property...
Gerd Hirschmann
2,369 PointsGerd Hirschmann
2,369 PointsThis is only for those who are still executing this "deprecated" course and use BenΒ΄s code example from above. It want work, you have to change the URL from "grepscience.com" back to "blog.teamtreehouse.com". Grepscience.com returns no JSON data therefore we cannot parse it. If we go back to the blog from teamtreehouse and add the lines from the tutorial and it works like a charm :) Here is my complete code from the "MainListActivity.java" file, feel free to c/p it: