Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial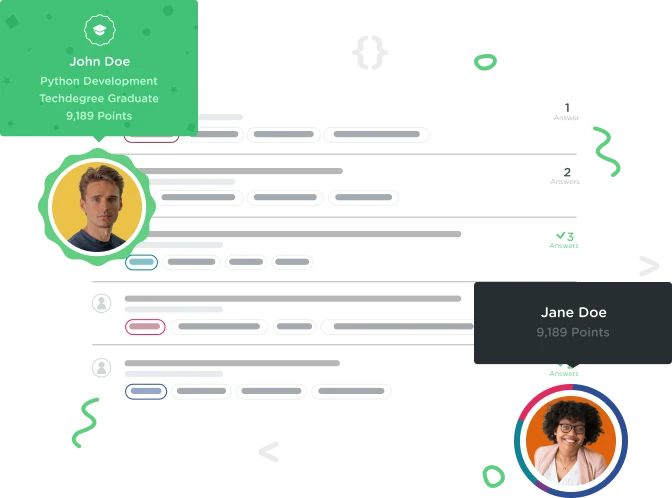

David Ritchey
2,753 Pointspart one method fix
i am not sure how to go about writing this code, i am sure i have overlooked something but where should i start?
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char letter = lastName;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
letter = lastNameInput.charAt(0);
if('A' <= letter >= 'N'){
lineNumber = 1;
}
else{
lineNumber =2;
}
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
3 Answers

Yanuar Prakoso
15,196 PointsHi David...
You need to fix your code from the top I guess, starting from the variable declaration and also the if statement:
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char letter = lastName; //<-- you just need to declare char letter, please do not put lastName directly here
//--- you can however do char letter = lastName.chatAt(0);
//or better yet: char letter = lastName.toUpperCase().charAt(0); to ensure all letters are capitalized
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
letter = lastNameInput.charAt(0);//<-- you haven't declare variable named lastNameInput please refer the code
//---- mentioned in my revision above
if('A' <= letter >= 'N'){//<--- you only need to tell Java if letter <='M'
//--- since smallest capitalized letter in alphabetic order is 'A' and Java knows that.
lineNumber = 1;
}
else{
lineNumber =2;
}
This is how I will do to pass the challenge:
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char firstLetterOfLastName;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
firstLetterOfLastName = lastName.toUpperCase().charAt(0);
if(firstLetterOfLastName <= 'M'){
lineNumber = 1;
} else {
lineNumber = 2;
}
return lineNumber;
}
}
I hope this can help a little.

David Ritchey
2,753 Pointspublic int getLineNumberFor(String lastName) { int lineNumber = 0; String lineA = "abcdefghijklm"; char letter = lastName.charAt(0); boolean apply = lineA.indexOf(letter) 'a' > 'n';
if(apply)
{ lineNumber = 1;
}
else{
lineNumber= 2;
}
return lineNumber;
}
}
/ i ended up with this but i think this was a overcomplicated /

Yanuar Prakoso
15,196 PointsYup I really think that is over complicated, As I said before Java is smart enough to understand alphabetical orders. You should take advantage of that and make your code simpler. Moreover, I am not sure this part of your code:
String lineA = "abcdefghijklm";
char letter = lastName.charAt(0);
boolean apply = lineA.indexOf(letter) 'a' > 'n';//<--this one? Will it work okay with Java compiler?
will work with Java compiler. Do you get any error whatsoever when running this code?

David Ritchey
2,753 Pointsit did have an error, that was me typing thoughts out, i see how you made yours work and other than converting to upper case i wanted to convey that but wasn't sure how, after seeing your code it makes much more sense now, thank you for the help!