Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial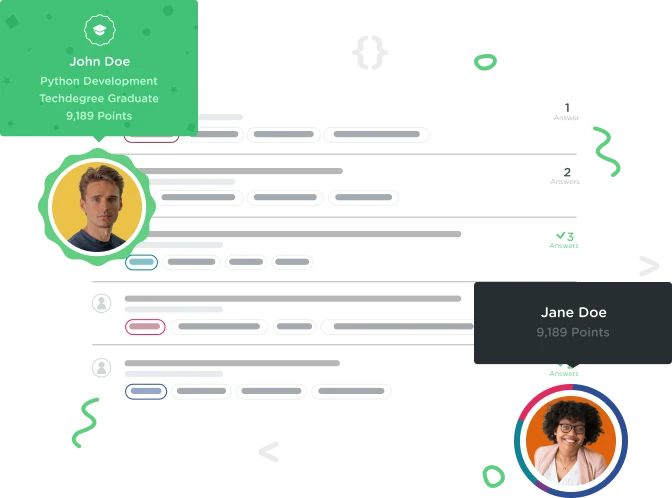

Anders Kristiansen
30 PointsPass javascript variable to rails controller
Hi, I am trying to use ajax to pass a javascript array to the rails controller. I have no idea if this is right but the way I'm currently trying to do this in js is:
$.ajax({
url : "/customurl",
type : "post",
data : data_value
});
My question is how should I create this route in rails, and how can I get the array in the controller, so it can be used? Of course, if there's a better way of doing this, I am more than happy to get an answer!
Thanks
1 Answer

Kyle Daugherty
16,441 PointsHi Anders,
You can do that by passing your data as an object and using the JSON.stringify method:
var array = [1, 2, 3, 4, 5];
$.ajax({
url : "/customurl",
type : "post",
data : { data_value: JSON.stringify(array) }
});
In your controller, the data would then be accessible by:
params[:data_value]
Hope this helps! It looks like you may have a second question about creating routes in Rails, but I wasn't sure exactly what your question was. Let me know.
Cheers!
Edit: One more thing...when trying out your ajax request, you can see how the data is being sent by taking a look in the console of the Chrome developer tools. Specifically, look at the Network tab and under the Name column you should see the request you made. Click that particular request and under the Headers tab there will be a section for Form Data.