Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial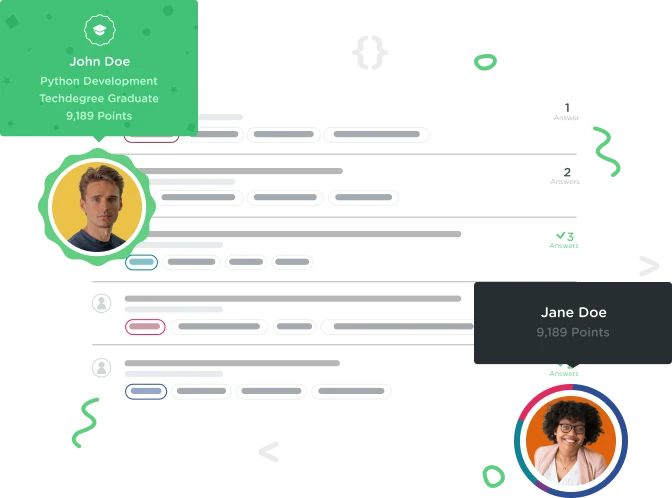

Iain Simmons
Treehouse Moderator 32,305 PointsPass second argument to map method instead of using bind
When updating the map
method in the Application
component, you say that we have to use the bind
method on the anonymous function, but I believe you can actually just pass this
as a second argument to the map
function to tell it what should be used as this
within it.
e.g.
{this.state.players.map(function(player, index) {
return (
<Player
key={player.id}
name={player.name}
score={player.score}
onScoreChange={function(delta) {
return this.onScoreChange(index, delta);
}}
/>
);
}, this)}
2 Answers

Seth Kroger
56,413 PointsGiven that you're using an ES6 arrow function to avoid the other bind() for onScoreChange, you can just use an arrow function for map as well. (Tried it out on my old workspace for the course and it works).
{ this.state.players.map((player, index) => {
return (
<Player
onScoreChange={delta => this.onScoreChange(index ,delta)}
name={player.name}
score={player.score}
key={player.id} />
);
}) }

Iain Simmons
Treehouse Moderator 32,305 PointsYeah I was using ES2015/ES6 for my local project files, but meant to share the ES5 code in the forums here because that's what @jim was using. I've updated my original question to reflect that.
Also, you could omit the curly braces, return and parentheses around your <Player />
component if you really want to make things compact with arrow functions. :)
{this.state.players.map((player, index) =>
<Player
onScoreChange={delta => this.onScoreChange(index ,delta)}
name={player.name}
score={player.score}
key={player.id} />
)}
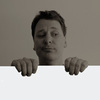
Sean T. Unwin
28,690 Pointsbind()
and passing an argument representing what this
should be, inside the called function, as a callback are essentially the same thing. However, bind()
is safer and more explicit.
See this StackOverflow answer for more information.

Iain Simmons
Treehouse Moderator 32,305 Pointsbind
creates a new function, so I wouldn't say they are the same. And if you are creating new functions with bind
within a component's render
method, you're creating them every time the component re-renders (i.e. on every state change, etc).
In any case, I much prefer the ES2015/ES6 use of arrow functions for this sort of thing.
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsJim Hoskins