Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial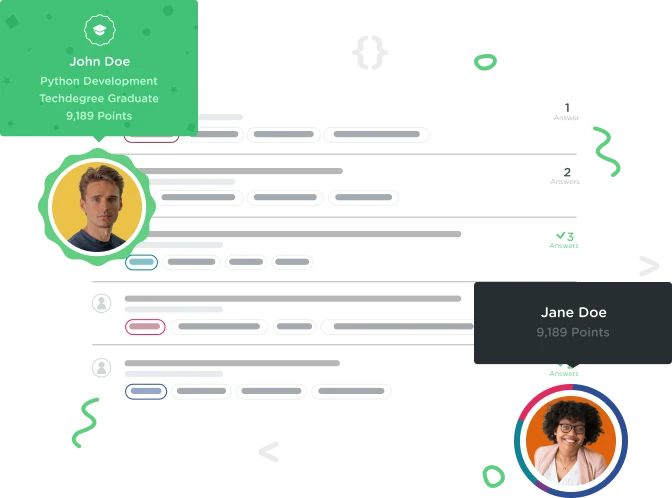
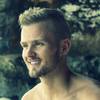
Torger Angeltveit
11,228 PointsPassing a GET variable through a link with PHP
Hi, I have trouble recieving and re using the variable i send through a GET varible.
THE VARIABLE: is a result from the mysqli dabase:
$product_id = $row['produkt_id'];
THE LINK on the index.php:
<a href="pages.php?id=<?php echo $product_id; ?>">
the code on page.php
if($_GET['id']==$product_id){
echo $product_id;
} else {
echo "failed";
}
When i push the link the URL is correct: mywebsite.com/pages.php?id=27 But the value of the varible cant be used when i try to echo it out. The if statement returns "failed"
2 Answers

Maximillian Fox
Courses Plus Student 9,236 PointsHi there,
You haven't specified the $product_id variable on your page.php. Therefore, your conditional will always return false.
However what you can do instead is use a conditional to see if $_GET['id'] has been set, and if so return the product id into a variable. Here's how you'd do it:
<?php
if(isset($_GET['id'])){
$product_id = $_GET['id'];
echo $product_id;
} else {
echo "failed";
}
?>
However there is a case where the product ID could actually be an empty string. So let's add another part to our conditional to check that the product ID is not an empty string.
<?php
if(isset($_GET['id']) && $_GET['id'] !== ''){
$product_id = $_GET['id'];
echo $product_id;
} else {
echo "failed";
}
?>
Now, you can actually improve this code even further. Let's assume that you only want to return a page with the product ID if and only if the product ID entered is a valid number, and if it exists in the page. Else, just go to the main products page. You can do this with your header function like this:
<?php
// Check if the ID is set and the ID is NOT an integer
if(isset($_GET['id'] && !is_int($_GET['id'])){
header('Location:pages.php');
exit;
}
// Since the above code will cause the script to stop if this is true,
// I no longer need an else statement. So continue the code as normal
$product_id = (isset($_GET['id']) ? $_GET['id'] : 0);
// This is a ternary operator, something very powerful i think you should get to know :)
echo $product_id;
?>
This will mean that a product has to be specified with a number in order to assign the product id. If not, it will redirect the user to pages.php and assign the ID to 0.
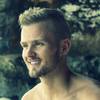
Torger Angeltveit
11,228 PointsAhh, it works! Thank you so much! I have been struggling with this the whole day! That was about the only thing i didnt try hehe. Thanks!

Maximillian Fox
Courses Plus Student 9,236 PointsNo problem :) Just remember when going to another page on a website, variables will not get passed along unless they are within the $_GET array, or if you include the file at the start of your script which has the declared variable.
Happy coding!