Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial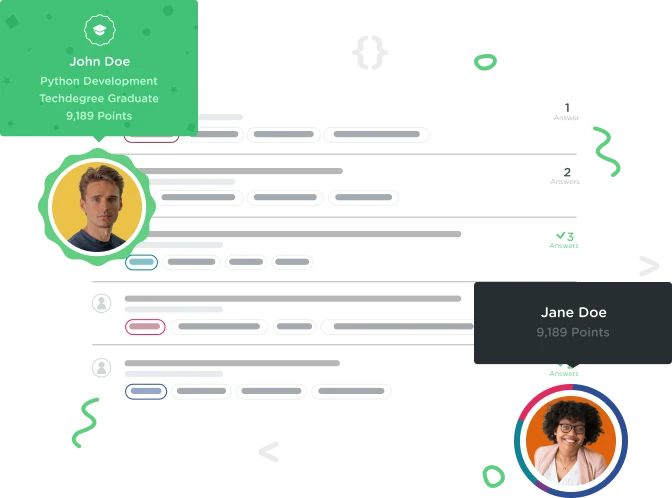

orange sky
Front End Web Development Techdegree Student 4,945 PointsPassing a value in a function in an array
Hello!
Based on the code below, I have some console and array related questions:
1) When I type 'mixture' in the console, on the side, there is a column with the heading, 'Filter properties'. Under this heading, you see:
0: "apple" 1:"orange" 2: "blue"
3:mixture<() length:4 proto: array[0]
a)The 3rd element in the array has an anonymous function. What does the '<' in the console mean? 3:mixture<()
b) I didnt not create a prototype, so what does: proto: array[0] mean
2) Why does show(10) return 'undefined'. Storing the function in the element of the array into another variable to then pass a value for that function in the new variable, seem to work in the video, why doesn't it work for me. I thought console.log(show(10)) should return 20
**this is what I get, when I type show(10) directly in the console:
show(10) undefined
Cheers!
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: The Math Object</title>
<link rel="stylesheet" href="core.css">
<script>
mixture = ["apple", "orange", "blue",function(a){a *2} ];
var show = mixture[3];
console.log(show(10));
</script>
</head>
<body>
</body>
</html>
2 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsYour anonymous function multiples the parameter by 2
but it doesn't do anything with the result of that operation. It's not returning it or storing it in some variable, so that's why show(10);
doesn't do anything.
You've created an array. Array, like any other object, will have a prototype property linked to the object it was created from. If this wasn't done automatically, you wouldn't be able to use any of the array methods on your array. Think about it, you create an array, but you don't define any methods on it, and yet you can still use them: sort
, shift
, pop
and so on.
This is because your array object inherits these through a prototype link to the "parent" object.
I believe the mixture<()
part is Firefox-specific debugging info. Since it's an anonymous function, it's hard to keep track of it (and other anonymous functions) in the call stack. Firefox tries to make this easier by letting you know where this function is (inside the mixture
array).

orange sky
Front End Web Development Techdegree Student 4,945 PointsHello Dino,
I have added 'return' to my function, but you said show(10) was not stored anywhere, but var show.... is giving me access to the function in the array, by referring to its position, shouldn't that be enough? var show = mixture[3];
sorry, I am not too sure how to store it, but I was able to get it to print by adding a return.
Cheers!!!
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: The Math Object</title>
<link rel="stylesheet" href="core.css">
<script>
mixture = ["apple", "orange", "blue",function(a){return a *2} ];
var show = mixture[3];
console.log(show(10));
</script>
</head>
<body>
</body>
</html>

Dino Paškvan
Courses Plus Student 44,108 PointsNo, I did not say that show(10)
isn't stored or displayed anywhere.
I said that the result of the operations that you do with the parameter passed to the function wasn't stored anywhere or returned. Now you are returning it so you can output it with console.log or even store it in a varaible:
var resultOfShow = show(10);