Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial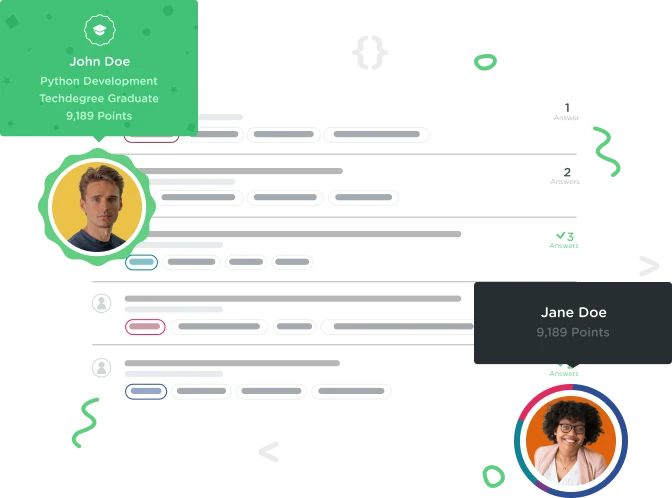
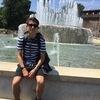
Mitch Little
11,870 PointsPassing an instance, to an instance. Is this okay?
After completing this code challenge, I have found a two different methods. Just curious as to which is written better.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location
}
}
let someBusiness = Business(name: "London", location: Location(latitude: 51.5074, longitude: 0.1278))
'''
Or
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location
}
}
let londonLocation = Location(latitude: 51.5074, longitude: 0.1278)
let someBusiness = Business(name:" London", location: londonLocation)
1 Answer
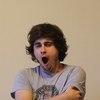
Arman Arutyunov
21,900 PointsIt is totally okay both ways you did. Which is better depends on the situation. If somewhere else you would need to use this exact location (for example for creating another instance of a business) then I would do the same following the DRY principle. If you are sure you would never ever use this location again then it is shorter to initialise Location directly as a parameter of Business. But what I'd also say, when you instantiate Location to a different constant, it looks much cleaner. I would prefer to write clean and readable code even if it is 1 line longer.
Hope it helps!