Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial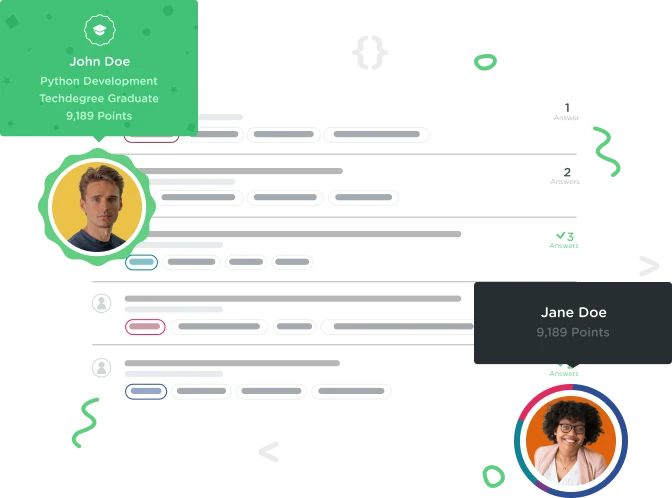
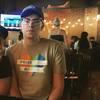
Steven Sullivan
11,616 PointsPassing arguments to functions
This code is pre-populated in the Workspace:
function print(message) { var div = document.getElementById('output'); div.innerHTML = message; }
Why is 'message' passed in? Does setting any string as an argument, declare it as a variable? Why wasn't it just declared before?
I see that it's used inside the function, but why wasn't it just declared in the code block if it was never previously declared?
function print() { var message = div.innerHTML; var div = document.getElementById('output'); }
Why wouldn't this work, or be equally as practical?
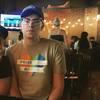
Steven Sullivan
11,616 PointsWhen you pass-in an argument you are kind of declaring a variable, it's kind of like saying "this is, whatever I say it is, whenever I say it is"
Understood. So it's a placeholder until you decide what to do with it.
And after that it holds the content or value of whatever you assigned it to?
Also, could you pass in the placeholder, AND a previously declared variable?
function print(message, previouslyDeclaredVariable) {
};
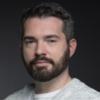
Cory Harkins
16,500 PointsI don't see any reason to, but that doesn't mean I really know beyond a shadow of a doubt that you can't.
I'm pretty sure (90%) you can't though, because those arguments are local and specific to that function alone.
You won't use the arguments outside of that function, i.e,
red = 'hello';
green= 'darkness';
blue = 'myoldfriend';
function color(red, green, blue) {
red= red;
green=green;
blue= blue;
console.log(red + green + blue);
}
console.log(red + ' ' + green + ' ' + blue);
color('way','down', 'yonder');
both results in two different things.
Instead of declaring in the function that red is now 'way', I gave it an argument that says hold this until I tell you what to do with it. In this instance I call it 'way'... if I wanted to, it could be 'caddywampus', etc. That's the power of local variables, and arguments.
When I console.log the variables, those are global, so the console.log doesn't recognize, or even see the variable change in the function.
I wouldn't think of using an argument to declare my variables within the argument parameters, rather I would say I have an argument, and inside the function assign the argument to the variable.
Here's something too, if you use arguments in your function, YOU HAVE to use them within your function.
this:
function balls(x) {
console.log('I have none');
}
is a no-go.
function balls(x) {
console.log('I ' + x + ' something');
}
balls('ate');
// the console will log "I ate something"
1 Answer
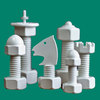
Steven Parker
230,274 PointsPassed-in parameters do not need to be explicitly declared in the function.
As Cory mentioned, they serve as placeholders for existing variables who's names are not known until the function is called. So "message" stands for a string which will be given to the function at a later time.
Now, regarding your second question and example:
function print() {
var message = div.innerHTML;
var div = document.getElementById('output');
}
You asked: "Why wouldn't this work, or be equally as practical?"
Well, for starters, you can't perform these statements in this order. You would need to initialize "div" first, before you could use it to access the innerHTML property. But the other line copies the current innerHTML of the div
into the variable message. Then both of these variables go "out of scope" when the function ends (which means they don't exist any more), so there's no lasting effect of the function running.
This is very different from what the original function does. It doesn't care what the current innerHTML value might be, but it changes it to the value that was passed in. This change persists after the function ends.
Does that clear it up?
Cory Harkins
16,500 PointsCory Harkins
16,500 Pointsmessage is being used as a placeholder. The goal for building functions is to have the intent to RE-USE them.
When you pass-in an argument you are kind of declaring a variable, it's kind of like saying "this is, whatever I say it is, whenever I say it is"
Your other example wouldn't work because you have no string attached to the var message.
In your example... for it to work:
In the first example, the message can be ANYTHING. print('cheetos');
The second can only be what you declared locally to that function. print();