Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial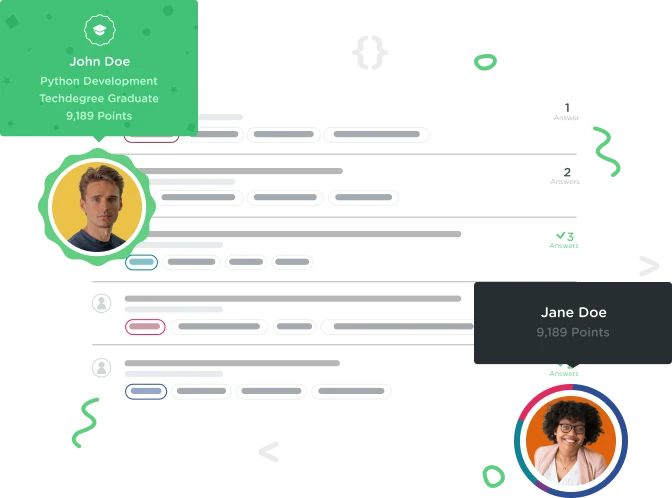
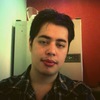
Jonathan Fernandez
8,325 PointsPassing Array data from table view controller to view controller?
Hi everyone.
So I'm basically trying to pass an array of a user's friends from a table view controller to a view controller.
The only way I know how is through what was thought in the self-destructing app class through segues.
Shown below is my code:
<p>
// Trying to retrieve data in this view controller (ThisControllerIsOfType: ViewController)
// ShareToFriendsViewController.h
//...
#import <UIKit/UIKit.h>
#import <Parse/Parse.h>
@class JWFFriendsTableViewController;
@interface JWFShareToFriendsViewController : UIViewController
@property (strong, nonatomic) NSMutableArray *friends; //Trying to pass data here
@property (strong, nonatomic) NSMutableArray *selectedFriends;
- (IBAction)cancel:(id)sender;
@end
//...
// Trying to Pass friends Array from this view controller to ShareFriendsViewController via Segue (ThisControllerIsOfType: TableViewController)
// FriendsViewController.m
//...
//Passing data from one view controller to the next via segue.
- (void) prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
if ([segue.identifier isEqualToString:@"showEditFriends"]) {
JWFEditFriendsTableViewController *viewController = (JWFEditFriendsTableViewController *)segue.destinationViewController;
viewController.friends = [NSMutableArray arrayWithArray:self.friends];
} else if ([segue.identifier isEqualToString:@"showShareToFriends"]) {
JWFShareToFriendsViewController *viewController = (JWFShareToFriendsViewController *)segue.destinationViewController;
viewController.friends = [NSMutableArray arrayWithArray:self.friends];
}
}
//...
</p>
Basically I get a bug every time I try to access the view controller and the app crashes. I have tried using a regular table view controller and it seems to pass the array data just fine. So is it not possible to share data from different view controller types?
If it doesn't work then the only other solution I know is to query again and get user relations and assign the objects to a new array within the view controller instead, but I want to avoid queries as much as possible.
Also Shown below is a screenshot of the bug I'm getting:
4 Answers
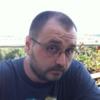
Robert Bojor
Courses Plus Student 29,439 PointsIf your destination view controller is embedded into a navigation controller then the segue.destinationViewController refers to the navigation controller itself and not to your view controller. Your view controller is the rootViewController in this case or the view at index 0 in the navigation stack.
I've run a test on this and you can use the following code inside prepareForSegue:sender:
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
UINavigationController *nav = segue.destinationViewController;
RBSecondViewController *svc = [nav.viewControllers objectAtIndex:0];
svc.receivedData = (NSMutableArray *)_sentData;
}
If you want the test project you can get it here: http://www.robertbojor.com/PassDataTest.zip

Meek D
3,457 Pointsare you using parse as you back-end database
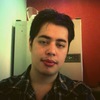
Jonathan Fernandez
8,325 Pointsyes I'm using Parse
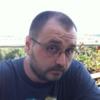
Robert Bojor
Courses Plus Student 29,439 PointsHi Jonathan,
Do you have the friends property defined in JWFShareToFriendsViewController header file?
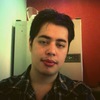
Jonathan Fernandez
8,325 PointsYes it's right at the header. If you look at comments in the code I have pasted I have:
1 header file for JWFShareToFriendsViewController - There is an NSMutableArray for friends.
1 implementation file for FriendsViewController.m
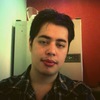
Jonathan Fernandez
8,325 PointsOk I have been doing some test and have some new info to share based on my test. To note I had been using a modal transition and embedding a navigation bar to the view controller I'm trying to pass data to. Now when I remove the Navigation Bar Embedded, the NSLog shows that it gets the array data on the next view controller! : ) & the app doesn't crash!! : D
(But now I have to use push segue to show the navigation bar.. in which I kind of prefer modal but it works for now)
After all this I would just like to know why it doesn't work with an embedded navigation bar? If anyone can explain this to me I'd be really grateful!! : )

Meek D
3,457 Pointsto use the push segue you must use a navigation controller
Jonathan Fernandez
8,325 PointsJonathan Fernandez
8,325 PointsThank you so much for explaining this and even taking the time to even test it out. I have been able to use your code and the data passes through and I can continue on with a modal design! : )
Now I have a new problem, which is passing this array data to a tableView-View within the ViewController that had received this array data lol. Although I think that question will be posted on another forum as the original question had been answered. Once again Thanks! : )
Robert Bojor
Courses Plus Student 29,439 PointsRobert Bojor
Courses Plus Student 29,439 PointsAdd the destination view controller as the datasource and delegate for the tableView, and use the receivedData array to output the number of rows in section and also go through it using the cellForRowAtIndexPath method...
You can add an additional check inside viewWillAppear and see if the receivedData array is empty and add an UILabel to the screen to notify the user that the table is empty...