Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial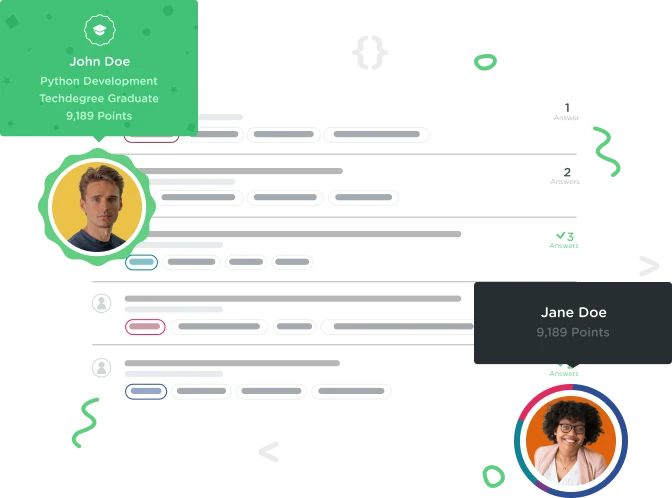

shu Chan
2,951 PointsPassing the state from one page down a component and back up to another page React.js
Hello I have this.state.order here that I wish to pass down to a component and back up to another page but I can't figure out how to do that
the state.order is here on this page
// Framework
import React, { Component } from "react";
// Components
import { Alert, Row, Col } from "reactstrap";
import Page from "../components/Page.jsx";
import Product from "../components/Product";
class Shop extends Component {
constructor(props) {
super(props);
this.state = {
merchants: [],
error: null,
loading: true,
data: null,
order: []
};
}
componentWillMount() {
Meteor.call("merchants.getMerchants", (error, response) => {
if (error) {
this.setState(() => ({ error: error }));
} else {
this.setState(() => ({ merchants: response }));
}
});
Meteor.call("merchants.alert", (error, response) => {
if (error) {
this.setState(() => ({ error: error }));
} else {
this.setState(() => ({ data: response }));
console.log(response);
}
});
}
componentDidMount() {
setTimeout(() => this.setState({ loading: false }), 800); // simulates loading of data
}
goBack = () => this.props.history.push("/");
goCart = () => this.props.history.push("/cart");
onAddToCart(cartItem) {
let { order } = this.state;
order.push(cartItem);
console.log(order);
}
render() {
const { loading } = this.state;
const { merchants, error } = this.state;
const { data } = this.state;
const getProductsFromMerchant = ({ products, brands }) =>
products.map(({ belongsToBrand, ...product }) => ({
...product,
brand: brands[belongsToBrand]
}));
const products = merchants.reduce(
(acc, merchant) => [...acc, ...getProductsFromMerchant(merchant)],
[]
);
if (loading) {
return (
<Page
pageTitle="Shop"
history
goBack={this.goBack}
goCart={this.goCart}
>
<div className="loading-page">
<i
className="fa fa-spinner fa-spin fa-3x fa-fw"
aria-hidden="true"
/>
<br /> <br />
<span>Loading...</span>
</div>
</Page>
);
}
return (
<Page pageTitle="Shop" history goBack={this.goBack} goCart={this.goCart}>
<div className="shop-page">
<h1>
{data}
</h1>
{products.map(({ id, ...product }) =>
<Product
{...product}
key={id}
history
data
onAddToCart={this.onAddToCart.bind(this)}
/>
)}
</div>
</Page>
);
}
}
export default Shop;
the component that every page loads on is here
// @flow
// Framework
import React from "react";
// Components
import Header from "../components/Header.jsx";
import Footer from "../components/Footer.jsx";
// Material UI
import MuiThemeProvider from "material-ui/styles/MuiThemeProvider";
export const Page = ({ children, pageTitle, history, goBack, goCart, order }) =>
<div className="page">
<Header goBack={goBack} goCart={goCart} history>
{pageTitle}
</Header>
<main>
<MuiThemeProvider>
{children}
</MuiThemeProvider>
</main>
<Footer />
</div>;
export default Page;
and the page I need to get the state to is here
// Framework
import React, { PureComponent } from "react";
// Components
import Page from "../components/Page.jsx";
import Button from "../components/Button.jsx";
class Home extends PureComponent {
goBack = () => this.props.history.push("/shop");
goCart = () => this.props.history.push("/cart");
render() {
return (
<Page pageTitle="Cart" history goBack={this.goBack} goCart={this.goCart}>
<div className="home-page">
<h2>
This is the cart page {this.props.order}
</h2>
<Button
onClick={() => {
this.props.history.push("/shop");
}}
>
Go shopping
</Button>
</div>
</Page>
);
}
}
export default Home;
I just can't figure out how to pass information down to a component and up to another page. Please help!