Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial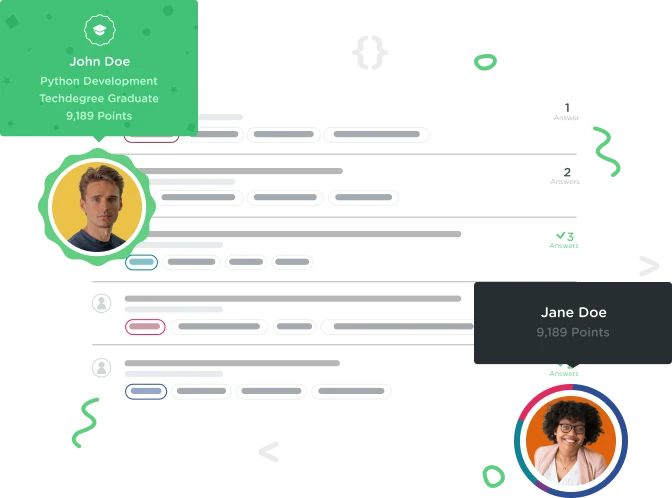
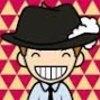
Aaron Selonke
10,323 PointsPassing the WebClient Challange
1) In task Three Directions say "In a using statement, create a MemoryStream object named stream, and pass the treehouseBytes variable as a parameter. Add another using statement around a new StreamReader object named reader. Pass the stream into the StreamReader constructor."
using(var webClient = new WebClient())
{
byte[] treehouseBytes = webClient.DownloadData("https://www.teamtreehouse.com");
}
If I wrap the new MemoryStream object in a new Using statement how can I pass the treehouseBytes in as a parameter. It does not exist before or after the webclient's using statement. How does this go?
2) Task One directions remind us that "The WebClient class implements IDisposable, so wrap it in a using statement." ...Why were we not wrapping the WebClient object in a using statement when learning how to use it in the video??
2 Answers
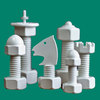
Steven Parker
231,275 PointsThe variable
treehouseByte
exists within the first using statement, and the new using statement will be placed after treehouseBytes is created but still within the original using.I wondered about that one myself. Perhaps a video boo-boo?

Ethan Batten
3,229 PointsYou have the to nest the using statements. Check out my example below. I also believe its a mess up in the wording.
using System; using System.IO; using System.Net;
namespace Treehouse.CodeChallenges { class Program { static void Main(string[] args) { Console.WriteLine(GetTreehouseHome()); }
public static string GetTreehouseHome()
{
string treehouse = "";
// Put code here
using(WebClient webClient = new WebClient())
{
byte[] treehouseBytes = webClient.DownloadData("https://www.teamtreehouse.com");
using(var stream = new MemoryStream(treehouseBytes))
{
using(var reader = new StreamReader(stream));
}
}
return treehouse;
}
}
}
Aaron Selonke
10,323 PointsAaron Selonke
10,323 PointsYeah, right before you replied I was starting to think that you have to nest the using statements
I found this is a better way to organize the code from the project, because its easier to remember the syntax and logic of all the pieces working together
Michael Kroon
16,255 PointsMichael Kroon
16,255 Points@StevenParker:: Yeah, it took some monkeying around but I was finally able to complete this challenge with the help of your comments. Even though nothing is being done with the 3rd 'using' block, yet, I still needed the brackets to actually pass the challenge. Here's my solution: