Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial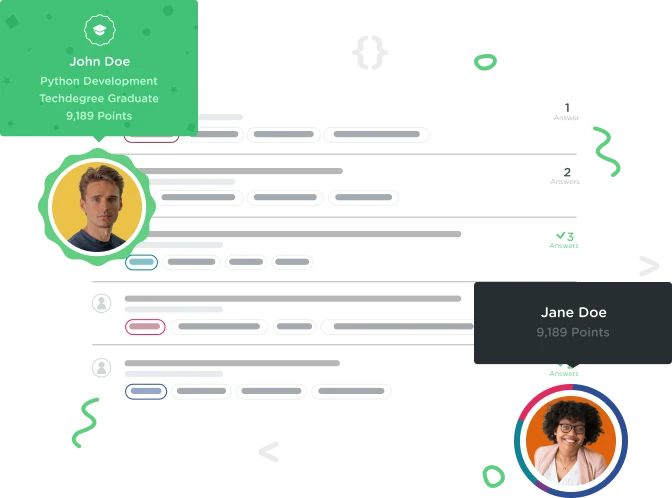

jordan stanley
7,541 PointsPause doesn't switch back to Resume when the timer switches to break
When the timer switches to break the pause/resume button stays as pause. If I click pause it changes to resume and when I click that it starts to count down and work normally. I thought it might be a problem with the clearAttemptStyles method but I can't see what would be wrong with it? I've included my controller and fxml code, any insight would be much appreciated.
package com.teamtreehouse.pomodoro.controllers;
import com.teamtreehouse.pomodoro.model.Attempt; import com.teamtreehouse.pomodoro.model.AttemptKind; import javafx.animation.Animation; import javafx.animation.KeyFrame; import javafx.animation.Timeline; import javafx.beans.property.SimpleStringProperty; import javafx.beans.property.StringProperty; import javafx.event.ActionEvent; import javafx.fxml.FXML; import javafx.scene.control.Label; import javafx.scene.control.TextArea; import javafx.scene.layout.VBox; import javafx.scene.media.AudioClip; import javafx.util.Duration;
public class Home { private final AudioClip mApplause; @FXML private VBox container;
@FXML private Label title;
@FXML private TextArea message;
private Attempt mCurrentAttempt;
private StringProperty mTimerText;
private Timeline mTimeline;
public Home() {
mTimerText = new SimpleStringProperty();
setTimerText(0);
mApplause = new AudioClip(getClass().getResource("/sounds/applause.mp3").toExternalForm());
}
public String getTimerText() {
return mTimerText.get();
}
public StringProperty timerTextProperty() {
return mTimerText;
}
public void setTimerText(String timerText) {
this.mTimerText.set(timerText);
}
public void setTimerText(int remainingSeconds) {
int minutes = remainingSeconds / 60;
int seconds = remainingSeconds % 60;
setTimerText(String.format("%02d:%02d", minutes, seconds));
}
private void prepareAttempt(AttemptKind kind) {
reset();
mCurrentAttempt = new Attempt(kind, "");
addAttemptStyle(kind);
title.setText(kind.getDisplayName());
setTimerText(mCurrentAttempt.getRemainingSeconds());
mTimeline = new Timeline();
mTimeline.setCycleCount(kind.getTotalSeconds());
mTimeline.getKeyFrames().add(new KeyFrame(Duration.seconds(1), e -> {
mCurrentAttempt.tick();
setTimerText(mCurrentAttempt.getRemainingSeconds());
}));
mTimeline.setOnFinished(e -> {
saveCurrentAttempt();
mApplause.play();
prepareAttempt(mCurrentAttempt.getKind() == AttemptKind.FOCUS ?
AttemptKind.BREAK : AttemptKind.FOCUS);
});
}
private void saveCurrentAttempt() {
mCurrentAttempt.setMessage(message.getText());
mCurrentAttempt.save();
}
private void clearAttemptStyles() {
container.getStyleClass().remove("playing");
for (AttemptKind kind : AttemptKind.values()){
container.getStyleClass().remove(kind.toString().toLowerCase());
}
}
private void reset() {
clearAttemptStyles();
if (mTimeline != null && mTimeline.getStatus() == Animation.Status.RUNNING) {
mTimeline.stop();
}
}
private void addAttemptStyle(AttemptKind kind) {
container.getStyleClass().add(kind.toString().toLowerCase());
}
public void playTimer() {
container.getStyleClass().add("playing");
mTimeline.play();
}
public void pauseTimer() {
container.getStyleClass().remove("playing");
mTimeline.pause();
}
public void handleRestart(ActionEvent actionEvent) {
prepareAttempt(AttemptKind.FOCUS);
playTimer();
}
public void handlePlay(ActionEvent actionEvent) {
if (mCurrentAttempt == null) {
handleRestart(actionEvent);
}
playTimer();
}
public void handlePause(ActionEvent actionEvent) {
pauseTimer();
}
}
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.?> <?import javafx.scene.image.Image?> <?import javafx.scene.image.ImageView?> <?import javafx.scene.layout.?> <?import javafx.scene.shape.SVGPath?> <VBox stylesheets="/css/main.css" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml" fx:controller="com.teamtreehouse.pomodoro.controllers.Home" fx:id="container"> <children>
<Label fx:id="title" text="Pomodoro"/>
<Label fx:id="time" text="${controller.timerText}"/>
<HBox styleClass="buttons">
<children>
<StackPane>
<children>
<StackPane styleClass="nested-action,play">
<children>
<HBox styleClass="svg-container">
<SVGPath styleClass="svg"
content="M1.6 17C1 17 0 16.2 0 15.6V1.3C0 .1 2.2-.4 3.1.4l8.2 6.4c.5.4.8 1 .8 1.7 0 .6-.3 1.2-.8 1.7l-8.2 6.4c-.5.2-1 .4-1.5.4z"/>
</HBox>
<Button text="Resume" onAction="#handlePlay"/>
</children>
</StackPane>
<StackPane styleClass="nested-action,pause">
<children>
<HBox styleClass="svg-container">
<SVGPath styleClass="svg"
content="M10.2 17c-1 0-1.8-.8-1.8-1.8V1.8c0-1 .8-1.8 1.8-1.8S12 .8 12 1.8v13.4c0 1-.8 1.8-1.8 1.8z" />
<SVGPath styleClass="svg"
content="M10.2 17c-1 0-1.8-.8-1.8-1.8V1.8c0-1 .8-1.8 1.8-1.8S12 .8 12 1.8v13.4c0 1-.8 1.8-1.8 1.8z" />
</HBox>
<Button text="Pause" onAction="#handlePause"/>
</children>
</StackPane>
</children>
</StackPane>
<StackPane styleClass="nested-action,restart">
<children>
<HBox styleClass="svg-container">
<SVGPath styleClass="svg"
content="M21 2.9C19.1 1 16.6 0 13.9 0c-1.1 0-2 .9-2 1.9s.9 1.9 2 1.9c1.7 0 3.2.6 4.4 1.8 2.4 2.4 2.4 6.3 0 8.7-1.2 1.2-2.7 1.8-4.4 1.8-1.7 0-3.2-.6-4.4-1.8-1.8-1.8-2.3-4.4-1.4-6.7L9.3 10c.2.4.5.7.9.8.4.1.8.1 1.2-.1.8-.4 1.1-1.3.8-2.1L10 4.3c0-.5-.2-1-.6-1.4l-.1-.1-.1-.2c-.2-.4-.5-.7-.9-.8-.4-.1-.8-.1-1.2.1L.9 4.8C.1 5.2-.2 6.1.2 6.9c.2.4.5.7.9.8.4.1.8.1 1.2-.1l2-.9c-1.2 3.5-.4 7.6 2.4 10.4C8.6 19 11.2 20 13.9 20s5.3-1 7.2-2.9C25 13.2 25 6.8 21 2.9z"/>
</HBox>
<Button text="Restart" onAction="#handleRestart"/>
</children>
</StackPane>
</children>
</HBox>
<TextArea fx:id="message" promptText="What are you doing?"/>
<ImageView>
<image>
<Image url="/images/pomodoro.png"/>
</image>
</ImageView>
</children>
</VBox>
2 Answers

Mustafa Alordowny
4,495 PointsI am not sure of the answer but try this. add else to handleplay method
public void
handlePlay(ActionEvent actionEvent) {
if (mCurrentAttempt == null) {
handleRestart(actionEvent);
} else {
playTimer();
}
}
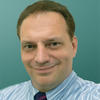
Mark Jones
Full Stack JavaScript Techdegree Graduate 29,362 PointsThis should work
public void playTimer(){
container.getStyleClass().add("playing");
mTimeLine.play();
}
public void pauseTimer(){
container.getStyleClass().remove("playing");
mTimeLine.pause();
}
fxml file
<StackPane styleClass="nested-action,play">
css file
.pause {
visibility: hidden;
}
.playing .pause {
visibility: visible;
}
.playing .play {
visibility: hidden;
}
Oshedhe Munasinghe
8,108 PointsOshedhe Munasinghe
8,108 PointsThanks!! :)