Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial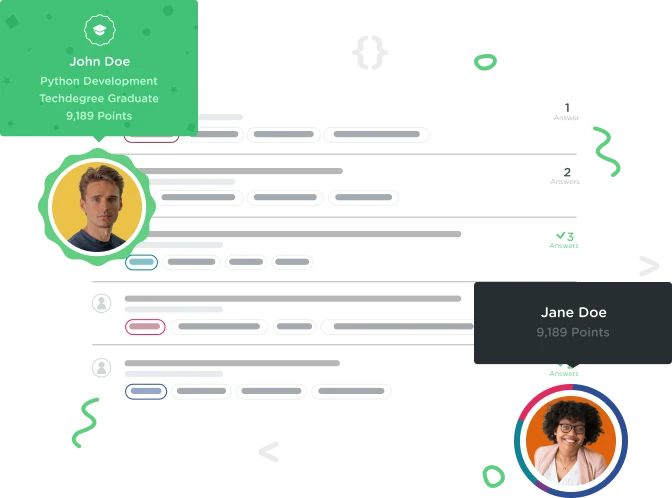
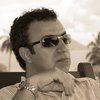
Shahin Zangenehpour
19,146 Pointspausing game run loop
is anyone aware of an effective method to pause the game run loop such that it can be associated with a pause button?
1 Answer

Nick Janes
5,487 PointsI've created a small tutorial that will give you a pause button and you can then play around with it to your pleasing.
We have a property we can use in our SKScene called 'paused'
Paused - A Boolean value that determines whether to run actions, animations, particle systems, and physics simulations in the scene graph.
The problem with just toggling paused is that the update method and the touchesBegan method are still called whilst the scene is paused. This leads to problems like when you un-pause, a ton of space cats may spawn or you can tap the screen a whole bunch while paused to shoot a ton of fireballs at once.
Step 1: Create a property inside of our gameplay scene that will be our button.
@interface THGamePlayScene ()
@property (nonatomic) SKSpriteNode *pauseButton;
Step 2: Then initialize it in initWithSize.
-(id)initWithSize:(CGSize)size {
if (self = [super initWithSize:size]) {
...
_pauseButton = [SKSpriteNode spriteNodeWithColor:[UIColor redColor] size:CGSizeMake(50, 50)];
_pauseButton.position = CGPointMake(self.frame.size.width-35, 35);
[self addChild:_pauseButton];
...
}
return self;
}
Step 3: Now we check if the user taps our button in the touchesBegan method. I've also added a little touch up to the color of the sprite so that we can see if it is going to resume or pause. This effect looks really cool!
if ( CGRectContainsPoint(_pauseButton.frame, position) ) {
self.scene.paused = !self.scene.paused;
if (self.scene.paused == true) {
_pauseButton.color = [UIColor greenColor];
} else if (self.scene.paused == false) {
_pauseButton.color = [UIColor redColor];
}
}
Excellent! Now we just need to fix a few bugs, one being to stop the space cats from spawning while paused and the other to stop allowing shots while paused.
Step 4: To Stop the user from making a super shot, which is actually really fun to do, you need to add this to the touches began method. All we're doing is surrounding the shoot projectile method call so that they can't shoot while paused.
if ( self.scene.paused == false ) {
[self shootProjectileTowardsPosition:position];
}
Step 5: Lastly, I surrounded the whole game content in the update method with an if statement so that the spawning only occurs whilst the game isn't paused.
- (void) update:(NSTimeInterval)currentTime {
if (self.scene.paused == false) {
//GAME UPDATE CONTENT
}
}

Nick Janes
5,487 PointsThere you go! This should do the trick! Let me know if you have any questions, requests or need help in any other way!
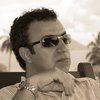
Shahin Zangenehpour
19,146 PointsThanks so much! I have implemented all the code but Xcode nags that position [as in if ( CGRectContainsPoint(_pauseButton.frame, position)] is an undeclared identifier.
So I changed it to _pauseButton.position to make Xcode stop nagging. The code runs well but I have a strange behaviour. No matter where I touch on the screen, the game pauses and then resumes with the subsequent touch with extra blasts coming from SpaceCat towards the touched position.
Did you get the same behaviour?

Nick Janes
5,487 PointsPut the code from step 3 under the shoot projectile method call, and that should do the trick. And for the fireballs that go everywhere, did you put the if statement around the [self shootProjectileTowardsPosition:position] line?
Give me the code to your touchesBegan method.
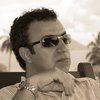
Shahin Zangenehpour
19,146 PointsVery cool indeed! It works well and as expected. I had put the 2nd if block separately under shootProjectile... method, my bad!
I'll start customizing the button! Thanks for all your help.

Nick Janes
5,487 PointsYeah sure thing! If you have any more questions feel free to tag me by putting @NickJanes and then clicking my name in the list! I just did colors for testing but you can set it to different images. Let me know how it turns out!
Nick Janes
5,487 PointsNick Janes
5,487 PointsI've done it before in my other games, I just downloaded the project files and am looking for a way to do it, I'll get back to you.