Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial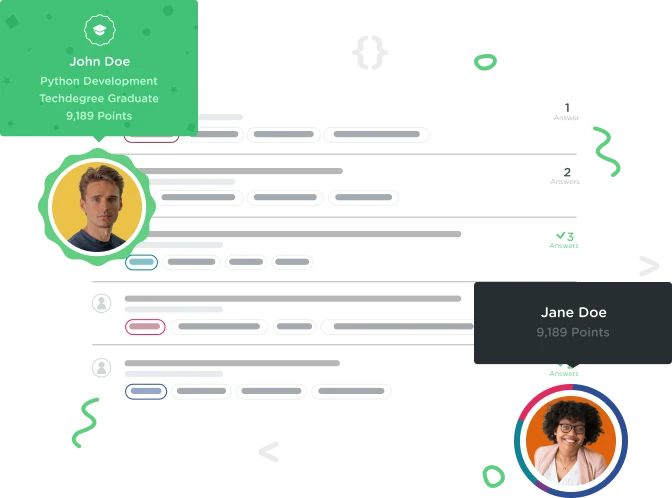
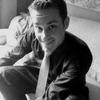
Joshua Farley
11,312 PointsPaypal - User-Defined Dollar Amount
I am trying to get a Paypal button to accept the input from the user as the dollar amount to be billed. This is for a bill payment option that allows the user to put in their invoice number and invoice amount, as each user's total will likely be different.
I assigned IDs to the relevant fields. The main thing that I am needing help with is the JavaScript. I am not certain that I have it right.
I need it to:
- select the value that the user enters
- feed that value into the Paypal value amount (initially set at $4.00)
- update on user input keyup event
- successfully submit to Paypal
Here is the code:
<form action="https://www.paypal.com/cgi-bin/webscr" method="post" target="_top"> <input type="hidden" name="cmd" value="_xclick"> <input type="hidden" name="business" value=busn@domain.com"> <input type="hidden" name="lc" value="US"> <input type="hidden" name="item_name" value="Bill Payment"> <input type="hidden" name="amount" id="payAMT" value="4.00"> <input type="hidden" name="currency_code" value="USD"> <input type="hidden" name="button_subtype" value="services"> <input type="hidden" name="no_note" value="0"> <input type="hidden" name="bn" value="PP-BuyNowBF:btn_paynowCC_LG.gif:NonHostedGuest"> <table> <tr> <td> <input type="hidden" name="on0" value="Invoice Number:">Invoice Number: </td> </tr> <tr> <td> <input type="text" name="os0" maxlength="200"> </td> </tr> <tr> <td> <input type="hidden" name="on1" value="Amount:">Amount: </td> </tr> <tr> <td> <input type="text" name="os1" id="valInput" maxlength="200"> </td> </tr> </table> <input type="image" src="https://www.paypalobjects.com/en_US/i/btn/btn_paynowCC_LG.gif" border="0" name="submit" alt="PayPal - The safer, easier way to pay online!"> <img alt="" border="0" src="https://www.paypalobjects.com/en_US/i/scr/pixel.gif" width="1" height="1"> </form>
<script>
var $sendAmount = $("#payAMT");
var $userAmount = $("#valInput").val();
function $updateAmount( $sendAmount ) {
$sendAmount.attr(value, $userAmount).keyup();
}
</script>
1 Answer
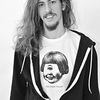
eck
43,038 PointsHey Joshua! I have edited your JS into something that does what I believe you are trying to do:
var $sendAmount = $("#payAMT");
var $userAmount = $("#valInput");
// When a keyup event occurs while #valInput is active...
$userAmount.keyup(function(){
// Set the value of #payAMT to equal the value of #valInput
$sendAmount.val( $(this).val() );
});
I also noticed that you are missing some quotation marks when you declared the value for the business input.
If you have any questions about that jQuery code, let me know! :D
Joshua Farley
11,312 PointsJoshua Farley
11,312 PointsThanks Erik, I believe that this is exactly what I was looking for!
Update, there was just one extra thing that this needed, the .append(). This was the final code that I used:
eck
43,038 Pointseck
43,038 PointsAppend will not do anything if you do not pass it an argument, but I am also pretty sure you do not need or want it in this situation :P