Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial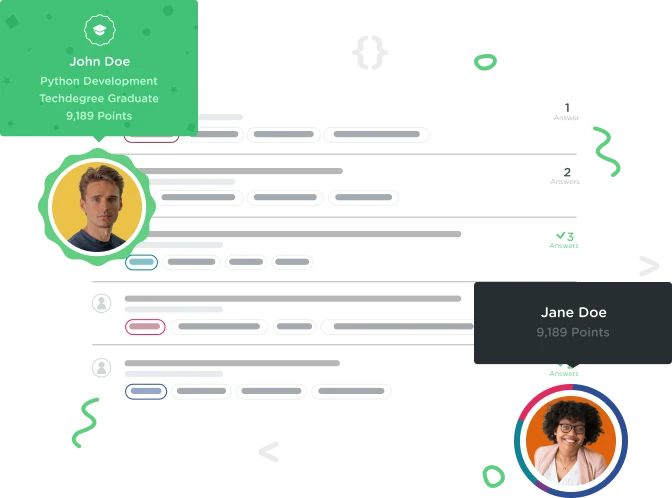
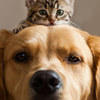
Devin Scheu
66,191 PointsPeewee Help (Python)
Question: Next, create a new Challenge. The language should be "Ruby", the name should be "Booleans".
Code:
from models import Challenge
all_challenges = Challenge.select()
Challenge = [
{ 'language': 'Ruby'
'name': 'Booleans'}
]
3 Answers
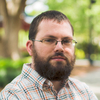
Kenneth Love
Treehouse Guest Teacherderekdekalb is correct. You need to create a new instance of the model.

Bryan Manhollan
Courses Plus Student 7,863 PointsThis one definitely threw me for a loop. For anyone still stuck on it, see below.
from models import Challenge
from peewee import *
all_challenges = Challenge.select()
Challenge.create(language="Ruby", name="Booleans")
sorted_challenges = all_challenges.order_by(Challenge.steps)

james white
78,399 PointsHere's the link to the challenge:
http://teamtreehouse.com/library/using-databases-in-python/meet-peewee/first-queries
Challenge 3 of 4 was the easy part.
The hard part was the final challenge:
Challenge Task 4 of 4
Finally, make a variable named sorted_challenges that is all of the Challenge records, ordered by the steps attribute. The order should be ascending, which is the default direction.
I was totally thrown off by this question, and I research for hours to find something about 'steps'.
Stupid me!
And just so no one else wastes time with a badly worded question,
the question should have said:
Finally, make a variable named sorted_challenges that retrieves all of the select() Challenge records, sorted using 'order_by()'.
In which case the code is obvious:
from models import Challenge
all_challenges = Challenge.select()
Challenge.create(language='Ruby', name='Booleans')
sorted_challenges = Challenge.select().order_by()
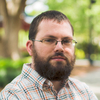
Kenneth Love
Treehouse Guest TeacherOh, hey, thanks, you actually found a mistake on my part. (and by that I mean, I fixed the code challenge so your code above no longer passes because that's not what I originally wanted to have pass)
The model has a steps
attribute (like how it has 'language' and 'name'). You need to specify sorting the records by that attribute in the order_by
method. We did something similar to this in the video where we found the highest-scoring student.
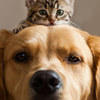
Devin Scheu
66,191 PointsI also had trouble with the forth part of this challenge, thanks for clearing it up for me Kenneth Love.
derekdekalb
15,072 Pointsderekdekalb
15,072 PointsI think we're relating our python dictionary objects to database table rows, so you want to create a row where the language value is 'Ruby' and your name value is 'Booleans'. It looks like your Challenge is an array with a single dictionary item.
If you try to create a record with a collection, you'll get an error.
Use this syntax instead:
Challenge.create(language='Ruby', name='Booleans')