Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial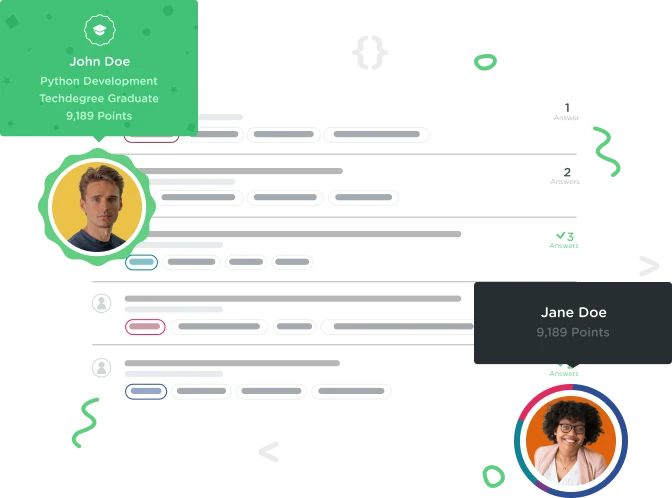
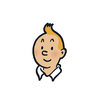
Yeeka Yau
7,410 Pointspeewee.ProgrammingError: column "id" does not exist
Hi, I am following along the tutorial on my local machine and using PostgreSQL as my database. I have already created the database, and a table 'staff', with the corresponding fields username and points.
However, when I try to run my script, I get the following error:
Traceback (most recent call last):
File "C:\Python34\lib\site-packages\peewee.py", line 3191, in execute_sql
cursor.execute(sql, params or ())
psycopg2.ProgrammingError: column "id" does not exist
LINE 1: ..." ("username", "points") VALUES ('yeyau', 10) RETURNING "id"
^
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "C:\Users\yeyau\Documents\tsc_exchange\staff.py", line 42, in <module>
add_staff()
File "C:\Users\yeyau\Documents\tsc_exchange\staff.py", line 25, in add_staff
Staff.create(username=s_member['username'],points=s_member['points']) #Staff is our db, create adds a new record to the db
File "C:\Python34\lib\site-packages\peewee.py", line 4127, in create
inst.save(force_insert=True)
File "C:\Python34\lib\site-packages\peewee.py", line 4287, in save
pk_from_cursor = self.insert(**field_dict).execute()
File "C:\Python34\lib\site-packages\peewee.py", line 2980, in execute
cursor = self._execute()
File "C:\Python34\lib\site-packages\peewee.py", line 2470, in _execute
return self.database.execute_sql(sql, params, self.require_commit)
File "C:\Python34\lib\site-packages\peewee.py", line 3199, in execute_sql
self.commit()
File "C:\Python34\lib\site-packages\peewee.py", line 3048, in __exit__
reraise(new_type, new_type(*exc_args), traceback)
File "C:\Python34\lib\site-packages\peewee.py", line 123, in reraise
raise value.with_traceback(tb)
File "C:\Python34\lib\site-packages\peewee.py", line 3191, in execute_sql
cursor.execute(sql, params or ())
peewee.ProgrammingError: column "id" does not exist
LINE 1: ..." ("username", "points") VALUES ('yeyau', 10) RETURNING "id"
^
My code is:
from peewee import *
db = PostgresqlDatabase(database='tsc_exchange', user='postgres', password='postgres')
class Staff(Model):
username = CharField(db_column='username', max_length=255, unique=True)
points = IntegerField(default=10)
class Meta:
database = db
staff = [
{'username': 'yeyau', 'points': 10},
{'username': 'crystal', 'points': 20},
{'username': 'spacecadet', 'points': 30},
{'username': 'marple', 'points': 40},
{'username': 'sherlock', 'points': 50},
{'username': 'doc', 'points': 60}
]
def add_staff():
for s_member in staff:
try:
Staff.create(username=s_member['username'],points=s_member['points']) #Staff is our db, create adds a new record to the db
except IntegrityError:
s_member_record = Staff.get(username=staff['username'])
if s_member_record['points'] != s_member[points]:
s_member_record.points = s_member['points']
s_member_record.save()
def top_staff():
staff = Staff.select().order_by(Staff.points.desc()).get()
return staff
if __name__ == '__main__':
db.connect()
db.create_tables([Staff], safe=True)
add_staff()
print("The staff member with the most points is {0.username}".format(top_staff()))
I tried to google the error message and perhaps the closest thing I could find was (I'm also curious as to which table it knows my Staff model is referring to in the db? My db currently has one table staff, but what if it had more?):
https://github.com/coleifer/peewee/issues/44
I tried to explicitly specify which column username refers to in my DB, but still the same error.
I'm not too sure how else to trouble shoot this - any help/ideas would be greatly appreciated!
2 Answers
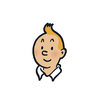
Yeeka Yau
7,410 PointsFigured it out - I had actually not only created the database in postgresql beforehand, but also the table as well (without the id column), seems like when the script is run, an additional column of id is created in the db - hence where the error occurred.
Basically don't create the table beforehand, just the DB.

Frederick Pearce
10,677 PointsNot sure I can help much with this, but one thought would be to start adding in test functions to make sure you have the right classes, column headers etc. Just adding print statements to check the value of things is a quick and dirty way to test things too. Or, you can also try the python debugger (pdb). Kenneth Love has nice lectures on testing and pdb. Good luck!!!
I also found this link where someone seemed to be having a similar issue that they were able to fix:
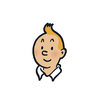
Yeeka Yau
7,410 PointsThanks for your help Frederick. Appreciate your suggestions, I managed to figure it out.
Frederick Pearce
10,677 PointsFrederick Pearce
10,677 PointsGlad you figured it out. So you just got rid of the db.create_tables(...), is that right? Can you post the corrected code? I'm trying to learn more about databases in python... Thank you!
Yeeka Yau
7,410 PointsYeeka Yau
7,410 PointsThe python code is actually the same, it's just that I created the table in postgres beforehand, when I did not need to.
Frederick Pearce
10,677 PointsFrederick Pearce
10,677 PointsOk, thanks for letting me know. I kept going through it and it looks very similar to the one in Kenneth Love's lecture, so couldn't see what was wrong. Glad you fixed it and thanks for the learning opportunity!