Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial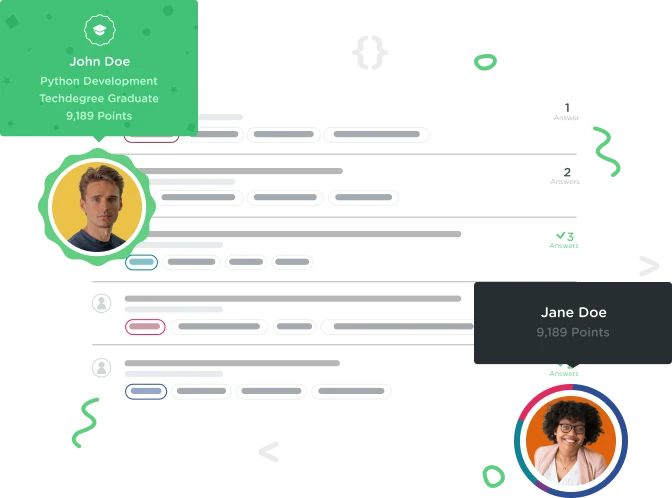
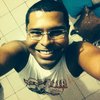
Bruno Calheira
12,625 PointsPerfecting the Basic Node.js App
I created a repository on GitHub to continue the exercise of Node.js Basic course.
Someone else would like to work (fun) with me ?
Fork me and enjoy!
https://github.com/calheira/basic-node-app
I finished the stage 2 of the project.
:D
Perfecting the Application
1 - Create user friendly error messages
2 - DONE! Make the first argument the topic area
e.g. node app.js Ruby chalkers davemcfarland joykesten2
3 - Extract all printing functions in to a printer.js file and import it in to your profile.js file.
4 - Other command line app ideas
A weather app - e.g. node weather.js 90210
A stock app - e.g. node stocks.js AAPL
8 Answers

Haziq Mir
Courses Plus Student 4,656 PointsI just got done steps 2 and 3. Here is mine: https://github.com/haziqmir/treehouse-points :)
Mathew Leland
19,236 PointsI didn't like the forecast.io since it doesn't let you enter zip codes (and asks for latitude and longitude instead). So I used wunderground's API and just adapted what we did in Chalkers' Node basics course:
var http = require("http");
//print out message
function printMsg(city, temp) {
var msg = "The forecast for " + city + " is " + temp;
console.log(msg);
}
//print out error message
function printError(error) {
console.error('Problem with request: ' + error.message);
}
function get(zip) {
//connect to api url
var request = http.get("http://api.wunderground.com/api/YourKey/forecast/geolookup/condiitons/q/" + zip + ".json", function(response){
var body = "";
//read the data
response.on('data', function(chunk) {
body += chunk;
});
response.on('end', function(){
//console.log(body);
if(response.statusCode ===200){
try {
//parse the data (read the data from a string in a program friendly way
var profile = JSON.parse(body);
//print out the data
printMsg(profile.location.city, profile.forecast.txt_forecast.forecastday[0].fcttext);
} catch(error) {
//handling a parse error
printError(error);
}
} else {
//handling status code error
printError({message: "There was an error getting a profile for " + zip + ". (" + http.STATUS_CODES[response.statusCode] +")"});
}
});
});
//Connection Error
request.on('error', printError);
}
module.exports.get = get;
And then in a separate file:
var forecast = require("./weather");
var cities = process.argv.slice(2);
cities.forEach(forecast.get);
This should allow you to create a query like so: "node forecast.js 10029" and get a forecast for the next few hours.
Seth Shober
30,240 PointsHere is mine. I used my own data set of zip codes to make the conversion to coordinates before passing it to forecast.io.
There is much room for improvement and expansion.
Flags would be nice (ex. --week to get weekly forcast, --daily to receive daily, -c for current);
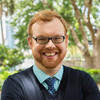
Andrew Chalkley
Treehouse Guest TeacherKeep at it you two!
Seth Shober
30,240 PointsHas anybody found an API that actually takes a zip code? Here's a CSV of all zip codes in the US with latitude/longitude coordinates you can then pass to any weather API. Just use the csvtojson module from NPM to convert the data to JSON.
I wrote a function that takes a zipcode and checks it to the data we now have stored. I ran a for loop to match the zip code and grab its coordinates. Hopefully this helps for now.
//TAKE A ZIP CODE AND CHECK IT WITH ZIP CODE JSON DATA
function getZipCoordinates(zip,data) {
//search for zip code
for(var i = 0; i < data.length; i++) {
var zipObj = data[i];
//if we have a match
if(zipObj.zipcode === zip){
//show me the coordinates as well as the matching object
var lat = zipObj.latitude;
var lon = zipObj.longitude;
console.log("latitude: " + lat);
console.log("longitude: " + lon);
console.log(zipObj);
//lets do some AJAX now
var url = "https://api.forecast.io/forecast/APIKEY/"+ lat + "," + lon;
//write your ajax call here
break; //stop the loop since we've got what we need
}
}
}
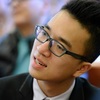
chanlennon
3,468 PointsExchangeRate Apps I have used yahoo query language api to build the exchange rate app(not stock app suggested though). And still not factorized at this moment, just wrap it all up by all the techniques learned in this course. Thank you very much chalkers!
The app need to pass in argv in pairs of currency.
Example:
node app.js usdcny eurusd

Alan Black
Courses Plus Student 6,305 PointsI didn't see anybody get this yet, so I tried it and created an example one with the Zip Code input to find the weather for that given location. Instead of the csv file, and parsing through it, you can use the Google Maps API for Geocoding to accomplish that. There is a node module available for it which I added link for in the code comments. There's really no error catching, and you can only enter one Zip Code at the moment, so those are things you could explore to enhance the application.

Ryan Scharfer
Courses Plus Student 12,537 PointsHi Robert,
The way I did it was somewhat similar. It first makes a get request to a Google API to get the longitude and latitude of a certain zip code, and only when that data is completely received does it make a second get request to the Forecast API to get the current temperature.
var https = require('https');
var userInput = process.argv.slice(2);
var lat = "";
var long = "";
var request = https.get('https://maps.googleapis.com/maps/api/geocode/json?components=country:' + userInput[0] + '|postal_code:' + userInput[1] + '&key=AIzaSyBso3tn5O2u_5E2aLRTUwgv2W15uMrjKBw', function(response){
var myData = '';
response.on("data", function(chunk){myData = myData + chunk});
response.on("end", function(){
var parsed = JSON.parse(myData);
lat = parsed.results[0].geometry.location.lat;
long = parsed.results[0].geometry.location.lng;
var request1 = https.get("https://api.forecast.io/forecast/611868f135a2a802ef15d57914517558/"+lat+","+long, function(response){
var data = "";
response.on("data", function(chunk){data = data + chunk});
response.on("end", function(){
var parsedData = JSON.parse(data);
var currentTemperature = parsedData.currently.temperature;
console.log("The current temperature at that location is "+currentTemperature+".");
});
})
})
})

Ryan Carson
23,287 PointsAwesome! :)