Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial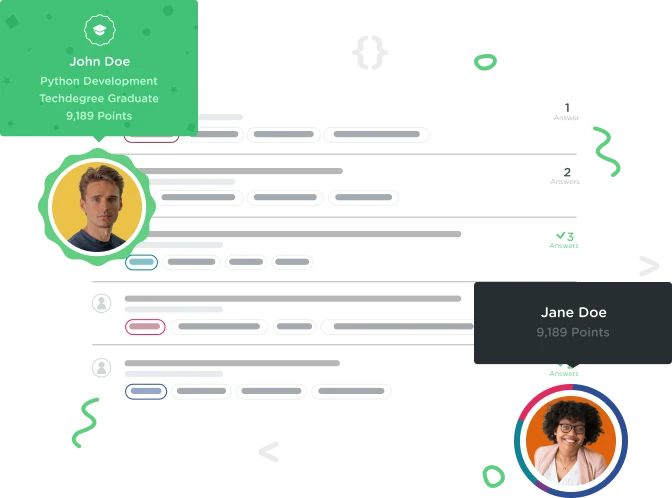
Aldrin Mabanta
11,768 PointsPerform: Traversing Elements with querySelector. Console "Uncaught TypeError: Cannot read property 'children" error
I keep getting this error ... can anybody check my line 76 - the 1st for loop? This is what console is telling me on Chrome and Safari, rechecked everything but I cannot find any issue on my spellings (or maybe I'm missing something).
//problem: user interaction doesnt provide desired results.
//solution: add interaction with the user to manage daily task.
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incomplete-task"); //incomplete-task
var completedTasksHolder = document.getElementById("completed-task"); //completed-task
//add a new task
var addTask = function () {
console.log("Add Task .....");
//when button is pressed
//create a new list item with the test from the #new-task
//input checkbox
//label
//input (text)
//button.edit
//button.delete
//each elements needs modified and appended
}
//edit an existing task
var editTask = function (){
console.log("Edit Task .....");
//when edit button is pressed
//if the class of parent is editMode
//switch from .editMode
//label text become the input value
//else
//switch to .editMode
//input value becomes the labels text
//Toggle .editMode
}
//delete an existing task
var deleteTask = function (){
console.log("Delete Task .....");
//when delete buitton is pressed
//remove the parent list item from the "ul"
}
//mark a task as complete
var taskCompleted = function(){
console.log("TaskCompleted .....");
//when the checkbox is checked
//append the task list item to the "completed task"
}
//mark a task as incomplete
var taskIncomplete = function (){
console.log("Task Incomplete .....");
//when the checkbox is unchecked
//append the task list item to the "incompleted task"
}
var bindTaskEvents = function (taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select taskListItems
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind taksinComplete to checkboxes
checkBox.onchange = checkBoxEventHandler;
}
//set click handler to the addTask functions
addButton.onclick = addTask;
//cycle over incompleteTasksHolder ul list items
for(var i = 0; i < incompleteTasksHolder.children.length; i++){
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//for each list item
//cycle over completedTasksHolder ul list items
//for each list item
//bind events to list items children (taskInComplete)
for(var i = 0; i < completedTasksHolder.children.length; i++){
//bind events to list items children (taskIncomplete)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
Here's the error on the console for Chrome & Safari
"Uncaught TypeError: Cannot read property 'children' of null"
2 Answers

Sjors Theuns
6,091 PointsHi Aldrin,
if I understood it right, the error "Uncaught TypeError: Cannot read property 'children' of null" is on line 76.
What the error is saying, is that it's trying to read the children of a null object. In this case incompleteTasksHolder seems to be null.
This variable is set with the following code:
var incompleteTasksHolder = document.getElementById("incomplete-task");
Are you sure the id "incomplete-task" exists? Because if not, the variable incompleteTasksHolder will be null resulting in the error.
Hope this helps.

Sjors Theuns
6,091 PointsHi Aldrin,
glad I could help.
Don't forget to close your question, at the moment it's still "open".
Best regards
Aldrin Mabanta
11,768 PointsAldrin Mabanta
11,768 PointsSjors - Thank you for the response, you brought light to the tunnel. The error came from a misspelled div id in this case its both "incomplete-tasks" and "completed-tasks" just missed an "s" at the ends. And I thought I was anal with spelling :( ...
So thank you for your help!!!
Best regards,
Aldrin