Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial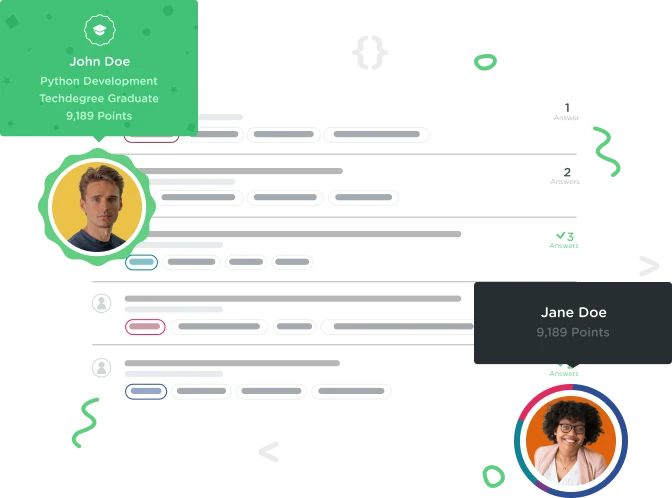
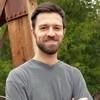
Shadd Anderson
Treehouse Project ReviewerPez Dispenser not dispensing the extra 6 pez
On the console, when I run the code, the dispenser only does the 12 "Chomp!"s, and then ate all the pez. After loading it with 4, and then 2 more pez, it doesn't do anything extra. No extra 6 Chomps at the end.
Here is my code:
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new pez dispenser.");
PezDispenser dispenser = new PezDispenser("Yoda");
System.out.printf("The dispenser character is %s\n",dispenser.getCharacterName());
if(dispenser.isEmpty()){
System.out.println("It is currently empty");
}
System.out.println("Loading...");
dispenser.load(MAX_PEZ);
if(!dispenser.isEmpty()){
System.out.println("It is no longer empty");
}
while(dispenser.dispense()){
System.out.println("Chomp!");
}
if (dispenser.isEmpty()){
System.out.println("Ate all the pez!");
}
}
dispenser.load(4);
dispenser.load(2);
while(dispenser.dispense()){
System.out.println("Chomp!");
}
}
and here is the code for the PezDispenser.java, if that helps as well:
public class PezDispenser {
public static final int MAX_PEZ = 12;
private String mCharacterName;
private int mPezCount;
public PezDispenser(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
public boolean dispense() {
boolean wasDispensed = false;
if (!isEmpty()){
mPezCount--;
wasDispensed = true;
}
return wasDispensed;
}
public boolean isEmpty(){
return mPezCount == 0;
}
public void load() {
load(MAX_PEZ);
}
public void load(int pezAmount) {
int newAmount = mPezCount + pezAmount;
mPezCount = newAmount;
}
public String getCharacterName() {
return mCharacterName;
}
}
1 Answer
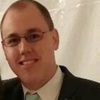
Jeremiah Montano
7,221 PointsYou put the last statement outside of the main class. If you move the brackets as shown below, it should work.
}// move this one
dispenser.load(4);
dispenser.load(2);
while(dispenser.dispense()){
System.out.println("Chomp!");
}
}
//down here
Shadd Anderson
Treehouse Project ReviewerShadd Anderson
Treehouse Project ReviewerD'oh! I could have sworn I checked for that already. I was pretty tired when I went over it though..... Thanks!
Chris Bensen
2,835 PointsChris Bensen
2,835 PointsThis doesn't explain the answer for me. If you could explain how you came to this answer?
Shadd Anderson
Treehouse Project ReviewerShadd Anderson
Treehouse Project ReviewerBen, In my particular case, I had closed the brackets on my main method before putting my code. Looking back at it now, it was pretty easy to do, considering the organization of my code was pretty awful. Lol. A good habit is to always write your closing brackets at the same time as you open them, then fill it in. That way you don't lose track of where you are, or have to go back trying to find your missing brackets, or in my case, writing outside of ones you've already closed.