Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial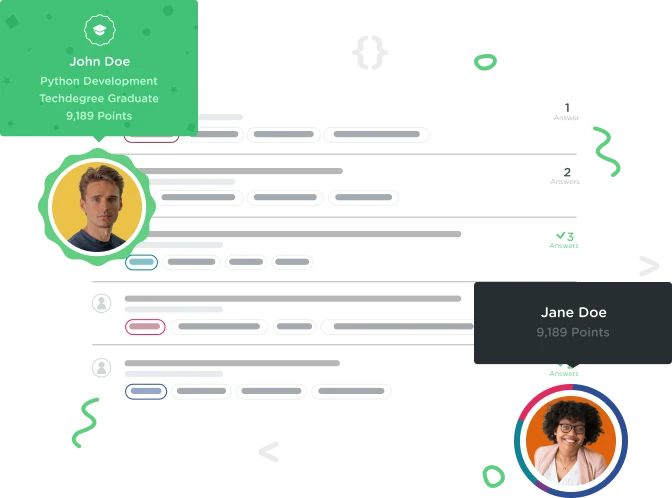

Ziad Alian
1,520 Points./PezDispenser.java:16: error: ';' expected
i'm getting an error
that's my Example.java
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new Pez Dispenser.");
PezDispenser dispenser = new PezDispenser ("Yoda");
System.out.printf("The dispnenser chracter is %s\n",
dispenser.getCharacterName());
if (dispenser.isEmpty()) {
System.out.println("It's curently empty");
}
System.out.println("loading...");
dispenser.load();
if (!dispenser.isEmpty()) {
System.out.println("It's not longer empty");
}
while (dispenser.dispense()) {
System.out.println("Chomp!");
}
if (dispenser.isEmpty()) {
System.out.println("Ate all the PEZ");
}
}
}
and this is PezDispenser.java
public class PezDispenser{
public static final int MAX_PEZ = 12;
private String mCharacterName;
private int mPezCount;
public PezDispenser(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
public boolean dispense() {
boolean wasDispensed = false;
if (!isEmpty()) {
mPezCount--;
wasDispensed = true;
}
return wasDispensed;
}
public boolean isEmpty(){
return mPezCount == 0;
}
public void load() {
mPezCount = MAX_PEZ;
}
public String getCharacterName(){
return mCharacterName;
}
}
in Console it's shows me this error
./PezDispenser.java:16: error: ';' expected
return wasDispensed
^
1 error
then treehouse:~/workspace$ javac Example.java && java Example
We are making a new Pez Dispenser.
The dispnenser chracter is Yoda
It's curently empty
loading...
2 Answers
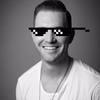
Seph Cordovano
17,400 PointsYou probably need to either save the new version where wasDispensed has the ";" at the end or if you're using the console recompile the saved version before running it again. Often beginners forget that they have to recompile after new saved versions. I recommend just chaining both commands:
$ javac Example.java && java Example
This will recompile both Example.java and PezDispenser.java since they are connected in the code.

ALBERT QERIMI
49,872 Pointsreturn wasDispensed ; You forgot ; at the end

Ziad Alian
1,520 Pointspublic boolean dispense() { boolean wasDispensed = false; if (!isEmpty()) { mPezCount--; wasDispensed = true; } return wasDispensed; }
i don't think that i forgot the semicolon
Siddesh Gannu
3,565 PointsSiddesh Gannu
3,565 PointsThanks! I was facing a similar problem. Recompiling did the trick
Seph Cordovano
17,400 PointsSeph Cordovano
17,400 PointsSiddesh Gannu, no problem! Glad it helped!