Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial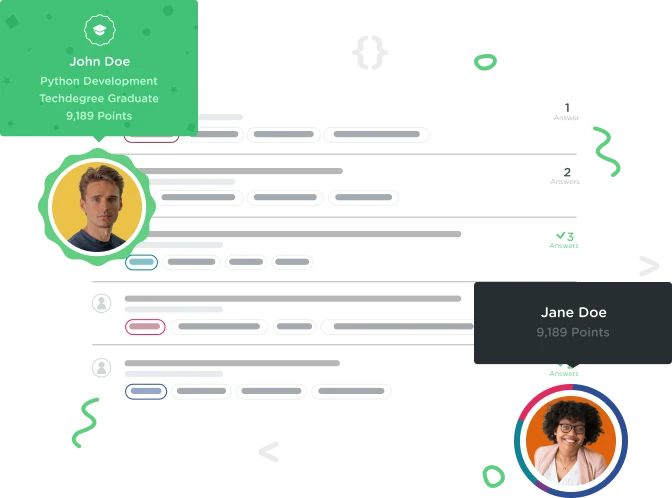
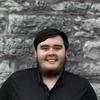
Michael Hulet
47,913 PointsPhone Numbers Print Twice
I've gotten to the part of the video where we write the print_phone_numbers
method, and for some reason, all my phone numbers print twice. Here is my version of the aforementioned method:
def print_phone_numbers
puts "\nPhone Numbers\n" + ("-" * 20)
phone_numbers.each do |number|
puts number
end
end
Here is the output of calling that method (after adding some phone numbers):
Phone Numbers
--------------------
Home: (555)-555-5555
Work: (555)-555-5555
Home: (555)-555-5555
Work: (555)-555-5555
Curiously, if I add another puts
statement in the each
loop, so the print_phone_numbers
method looks like this:
def print_phone_numbers
puts "\nPhone Numbers\n" + ("-" * 20)
phone_numbers.each do |number|
puts "Hi"
puts number
end
end
The output looks like this:
Phone Numbers
--------------------
Hi
Home: (555)-555-5555
Hi
Work: (555)-555-5555
Home: (555)-555-5555
Work: (555)-555-5555
Any idea how I could fix this?
EDIT: Here's the contents of both files involved in this program, since it was requested
require "./phone_number.rb"
class Contact
attr_writer :first_name, :middle_name, :last_name
attr_reader :phone_numbers
def initialize
@phone_numbers = []
end
def first_name
return @first_name
end
def middle_name
return @middle_name
end
def last_name
return @last_name
end
def full_name
name = first_name
if !middle_name.nil?
name += " #{middle_name}"
end
return name += " #{last_name}"
end
def last_first
name = last_name + ", " + first_name
if !middle_name.nil?
name += " #{middle_name.slice(0, 1)}"
end
return name
end
def first_last
return first_name + " " + last_name
end
def to_s(format = "full_name")
case format
when "full_name"
return full_name
when "last_first"
return last_first
when "first"
return first_name
when "last"
return last_name
else
return first_last
end
end
def add_phone_number(kind, number)
phone_number = PhoneNumber.new
phone_number.kind = kind
phone_number.number = number
phone_numbers.push phone_number
end
def print_phone_numbers
puts "\nPhone Numbers\n" + ("-" * 20)
iterations = 0
phone_numbers.each do |number|
puts number
iterations += 1
puts iterations
end
end
end
michael = Contact.new
michael.first_name = "Michael"
michael.last_name = "Hulet"
michael.add_phone_number("Home", "(555)-555-5555")
michael.add_phone_number("Work", "(555)-555-5555")
puts michael.print_phone_numbers
class PhoneNumber
attr_accessor :kind, :number
def to_s
return "#{kind}: #{number}"
end
end
2 Answers

Owen Tran
6,822 Pointsdef print_phone_numbers
puts "\nPhone Numbers\n" + ("-" * 20)
phone_numbers.each do |number|
puts number # <<< remove this
end
end

Owen Tran
6,822 PointsWhat is phone_numbers? Can you post the rest of your code? And how are you calling the method?
This works:
phone_numbers = {"Home:" => '(555)-555-5555', "Work:" =>'(555)-555-5555'}
puts "\nPhone Numbers\n" + ("-" * 20)
phone_numbers.each do |key,number|
puts key + number
end
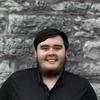
Michael Hulet
47,913 Pointsphone_numbers
is an array instance variable of the Contact
class. Per your request, I edited my post to include all of my code
Owen Tran
6,822 PointsOwen Tran
6,822 Pointsor at the bottom
puts michael.print_phone_numbers
remove the puts to just :
michael.print_phone_numbers
The put here means you are putting the return value, then you are putting AGAIN in the method, that's why it's putting twice. Make sense?
Owen Tran
6,822 PointsOwen Tran
6,822 PointsSo remove one of the puts, doesn't matter which one
Michael Hulet
47,913 PointsMichael Hulet
47,913 PointsI removed one of the extraneous
puts
statements, and it fixed my problem. Thanks so much!Owen Tran
6,822 PointsOwen Tran
6,822 PointsYou're welcome :)