Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial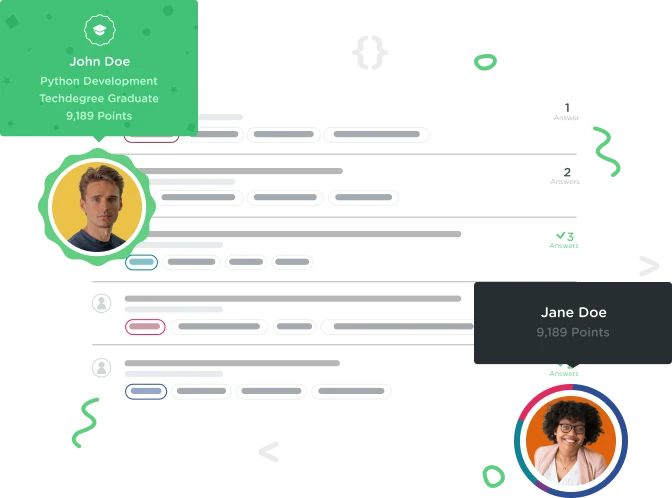

Jack Ryder
7,286 PointsPhoto Bombers - Access Tokens
During the Introducing Gesture Recognizers video i seem to keep getting the error 400 even when Sam's code turns successful,
here's my code, any help would be greatly appreciated!
#import "PhotoCell.h"
#import "SAMCache/SAMCache.h"
@implementation PhotoCell
- (void) setPhoto:(NSDictionary *)photo {
_photo = photo;
NSURL *url = [[NSURL alloc] initWithString:_photo [@"images"] [@"thumbnail"] [@"url"]];
[self downloadPhotoWithURL:url];
}
- (id)initWithFrame:(CGRect)frame
{
self = [super initWithFrame:frame];
if (self) {
// Initialization code
self.imageView = [[UIImageView alloc] init];
UITapGestureRecognizer *tap = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(like)];
tap.numberOfTapsRequired = 2;
[self addGestureRecognizer:tap];
[self.contentView addSubview:self.imageView];
}
return self;
}
- (void) layoutSubviews {
[super layoutSubviews];
self.imageView.frame = self.contentView.bounds;
}
- (void) downloadPhotoWithURL: (NSURL *) url{
NSString *key = [[NSString alloc] initWithFormat:@"%@-thumbnail", self.photo [@"id"]];
UIImage *photo = [[SAMCache sharedCache] imageForKey:key];
if (photo) {
self.imageView.image = photo;
return;
}
NSURLSession *session = [NSURLSession sharedSession];
NSURLRequest *request = [[NSURLRequest alloc] initWithURL:url];
NSURLSessionDownloadTask *task = [session downloadTaskWithRequest:request completionHandler:^(NSURL *location, NSURLResponse *response, NSError *error) {
NSData *data = [[NSData alloc] initWithContentsOfURL:location];
UIImage *image = [[UIImage alloc] initWithData:data];
[[SAMCache sharedCache] setImage:image forKey:key];
dispatch_async(dispatch_get_main_queue(), ^{
self.imageView.image = image;
});
} ];
[task resume];
}
- (void) like {
NSLog(@"Link: %@", self.photo[@"Link"]);
NSURLSession *session = [NSURLSession sharedSession];
NSString *accessToken = [[NSUserDefaults standardUserDefaults] objectForKey:@"AccessToken"];
NSString *urlString = [[NSString alloc] initWithFormat:@"https://api.instagram.com/v1/media/%@/likes?access_token=%@", self.photo[@"id"], accessToken];
NSURL *url = [[NSURL alloc] initWithString:urlString];
NSMutableURLRequest *request = [[NSMutableURLRequest alloc] initWithURL:url];
request.HTTPMethod = @"Post";
NSURLSessionDataTask *task = [session dataTaskWithRequest:request completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
dispatch_async(dispatch_get_main_queue(),^{
[self showLikeCompletion];
});
NSLog(@"response %@", response);
NSLog(@"data %@", [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding]);
}];
[task resume];
}
- (void) showLikeCompletion {
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Liked!" message:nil delegate:nil cancelButtonTitle:nil otherButtonTitles:nil, nil];
[alert show];
double delayInSeconds = 1.0;
dispatch_after(dispatch_time(DISPATCH_TIME_NOW, (int64_t)(delayInSeconds * NSEC_PER_SEC)), dispatch_get_main_queue(), ^{
[alert dismissWithClickedButtonIndex:0 animated:YES];
});
}
@end
3 Answers

Jack Ryder
7,286 PointsYeah I've got that code exactly in my PhotoViewController, here's my code:
#import "PhotosViewController.h"
#import "PhotoCell.h"
#import <SimpleAuth/SimpleAuth.h>
@interface PhotosViewController ()
@property (nonatomic) NSString *accessToken;
@property (nonatomic) NSArray *photos;
@end
@implementation PhotosViewController
- (instancetype) init {
UICollectionViewFlowLayout *layout = [[UICollectionViewFlowLayout alloc] init];
layout.itemSize = CGSizeMake(106.0, 106.0);
layout.minimumInteritemSpacing = 1.0;
layout.minimumLineSpacing = 1.0;
return (self = [super initWithCollectionViewLayout:layout]);
}
- (void) viewDidLoad {
[super viewDidLoad];
self.title = @"Photo Bombers";
[self.collectionView registerClass:[PhotoCell class] forCellWithReuseIdentifier:@"photo"];
self.collectionView.backgroundColor = [UIColor whiteColor];
NSUserDefaults *userDefaults = [NSUserDefaults standardUserDefaults];
self.accessToken = [userDefaults objectForKey:@"accessToken"];
if (self.accessToken == nil) {
[SimpleAuth authorize:@"instagram" options:@{@"scope": @[@"likes"]} completion:^(NSDictionary *responseObject, NSError *error) {
self.accessToken = responseObject[@"credentials"][@"token"];
[userDefaults setObject:self.accessToken forKey:@"accessToken"];
[userDefaults synchronize];
[self refresh];
}];
}
else {
[self refresh];
}
}
- (void) refresh {
NSURLSession *session = [NSURLSession sharedSession];
NSString *urlString = [[NSString alloc]initWithFormat:@"https://api.instagram.com/v1/tags/photobomb/media/recent?access_token=%@", self.accessToken];
NSURL *url = [[NSURL alloc] initWithString:urlString];
NSURLRequest *request = [[NSURLRequest alloc] initWithURL:url];
NSURLSessionDownloadTask *task = [session downloadTaskWithRequest:request completionHandler:^(NSURL *location, NSURLResponse *response, NSError *error) {
NSData *data = [[NSData alloc] initWithContentsOfURL:location];
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:kNilOptions error:nil];
self.photos = [responseDictionary valueForKeyPath:@"data"];
dispatch_async(dispatch_get_main_queue(), ^{
[self.collectionView reloadData];
});
}];
[task resume];
}
- (NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section {
return [self.photos count];
}
- (UICollectionViewCell *) collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath {
PhotoCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:@"photo" forIndexPath:indexPath];
cell.backgroundColor = [UIColor lightGrayColor];
cell.photo = self.photos [indexPath.row];
return cell;
}
@end

Thomas Nilsen
14,957 PointsOk I think I see the problem. When you store the token with NSUserDefaults, you store it as 'accessToken'. In you PhotoCell.m, when you are retrieving the key in - (void)like, you write:
NSString *accessToken = [[NSUserDefaults standardUserDefaults] objectForKey:@"AccessToken"];
Captial 'A'. change that to small.

Jack Ryder
7,286 Pointsso simple!! cheers man

Thomas Nilsen
14,957 PointsNo problem :)

Bradley White
21,285 PointsIm having the same problem. I've checked these areas already. Any help?
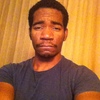
Stephen Whitfield
16,771 PointsAre you still needing help with this?
Thomas Nilsen
14,957 PointsThomas Nilsen
14,957 PointsDid you remember to go in the PhotosViewController and do this:
Notice the options' dictionary that gets passed in.