Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial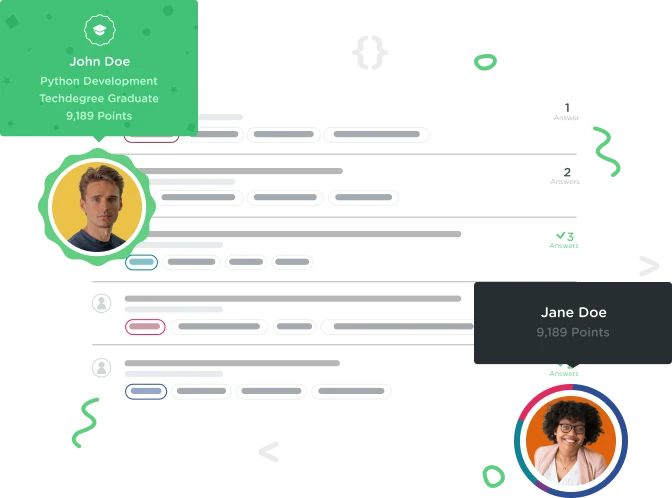
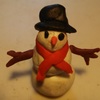
Joni Carlson
11,940 PointsPhotos display vertically not horizontally. What is missing?
My Flickr photos show, but instead of displaying horizontally like in the video, mine are displaying vertically.
flickr.js
$(document).ready(function() {
var flickerAPI = "http://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=?";
$('form').submit(function (evt) {
var $submitButton = $('#submit');
var $searchField = $('#search');
evt.preventDefault();
$searchField.prop("disabled",true);
$submitButton.attr("disabled", true).val("searching....");
var animal = $searchField.val();
$('#photos').html('');
$.getJSON(flickerAPI, {
tags: animal,
format: "json"
},
function(data){
var photoHTML = '';
if (data.items.length > 0) {
$.each(data.items,function(i,photo) {
photoHTML += '<li class="grid-25 tablet-grid-50">';
photoHTML += '<a href="' + photo.link + '" class="image">';
photoHTML += '<img src="' + photo.media.m + '"></a></li>';
}); // end each
} else {
photoHTML = "<p>No photos found that match: " + animal + ".</p>"
}
$('#photos').html(photoHTML);
$searchField.prop("disabled", false);
$submitButton.attr("disabled", false).val("Search");
}); // end getJSON
}); // end click
}); // end ready
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX Flickr Photo Feeder</title>
<link href='http://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<div class="heading">
<h1>Flickr Photo Feeder</h1>
<p>Choose which kind of animal you'd like to return photos of...</p>
</div>
<ul class="filter-select">
<li><button>Dog</button></li>
<li><button>Cat</button></li>
<li><button>Moose</button></li>
</ul>
</div>
<div id="photos">
</div>
</div>
</div>
</div>
<script src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
<script src="js/flickr.js"></script>
</body>
</html>
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsBefore you loop through the results and create the li
elements for each of them, you need to have a ul
(unordered list) element to put them in.
In the video you'll notice he assigns the photoHTML
variable the opening ul
tag initially, and the last thing concatenated to it is the closing tag.
e.g.
var photoHTML = '<ul>';
...
photoHTML += '</ul>';
Note that you shouldn't have a p
tag as a direct child of a ul
tag, so the 'No photos found' text should be put in an li
element also or instead of the p
tag.
Orlando Watson
12,186 PointsOrlando Watson
12,186 PointsThat sounds like a CSS problem. Check if you added the relevant classes to each list item when building them.