Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial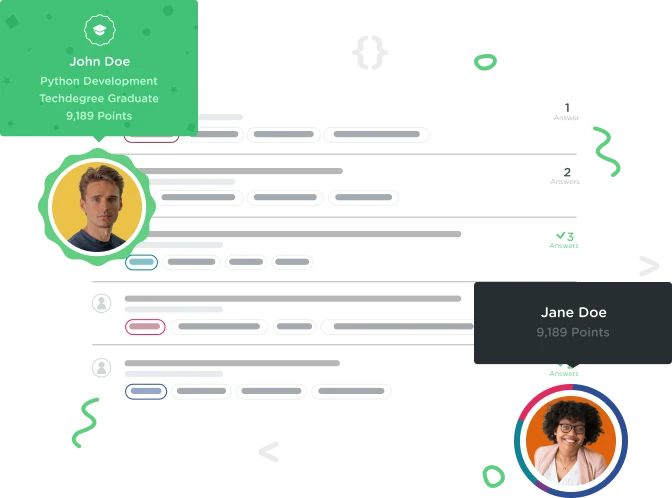
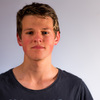
bremersharp
2,886 PointsPHP and MySQL Problems
Hi I am making a website that uses MySQL to save data from a form. The problem that im having is when I submit the form it works fine. But when i refresh the same page with out filling in the form it creates a new blank output.
Here is the code that i used to post the data.
mysql_connect("localhost","root","root");//database connection
mysql_select_db("employees");
$order = "SELECT * FROM data_employees ORDER BY name";
//order to search data
//declare in the order variable
$result = mysql_query($order);
//order executes the result is saved
//in the variable of $result
while($data = mysql_fetch_row($result)){
echo("<tr><td>$data[1]</td><td>$data[2]</td><td>$data[3]</td></tr>");
}
?>
if you have any suggestions feel free to let me know. Goes the same with hints an tips thanks
2 Answers
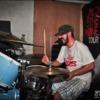
Mike Costa
Courses Plus Student 26,362 PointsHi Bremer,
I would try to steer clear of using mysql functions in PHP because future versions of PHP will not be able to support it. The PHP manual suggests using MySQL improved functions
http://www.php.net/manual/en/class.mysqli.php
or PDO MySQL function (for an object oriented way of connecting with a database)
http://www.php.net/manual/en/ref.pdo-mysql.php
For mysqli, (to keep things a little simple for your project), you could try this:
// mysqli_connect mankes the connection to your DB. parameters: (host, user, pw, database)
$connection = mysqli_connect("localhost", "root", "root", "employees");
//echos an error if there's no connection
if (!$connection) {
trigger_error ('Could not connect to MySQL: ' . mysqli_connect_error() );
}
// query string
$query = "SELECT * FROM data_employees ORDER BY name";
// get result using mysqli_query.
$result = mysqli_query($connection, $query);
// while loop to retrieve data from the table
while($row = mysqli_fetch_row($result)){
echo "This is element 0: " . $row[0] . "<br>";
echo "This is element 1: " . $row[1] . "<br>";
echo "This is element 2: " . $row[2] . "<br>";
}
// Pending you no longer need this result later in the script, you can free it from memory
mysqli_free_result($result);
This script works with my databases and I've gotten no errors and the proper output. Of course, you can arrange the output how you would like, I just separated each element to show what was happening. Hope this helps! :)
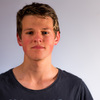
bremersharp
2,886 PointsThanks for your help. Just one more thing, do you think that having an AUTO_INCREMENT would be the cause of the problem. But thanks and ill see if it helps.
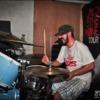
Mike Costa
Courses Plus Student 26,362 PointsThe auto increment shouldn't be a problem. The code I posted just retrieves the information from a table in the database. If the problem is still occurring, then there might be an issue with another part of your code. If you want to post the rest of your form script here, I can try to help you a little further. :)
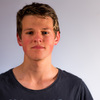
bremersharp
2,886 PointsThanks I fixed the problem with the database. I tried out your code and it worked fine (no errors) but i couldn't get it to display a table. Ill post the rest of my code when i get a chance. (I'm on a phone). Its been good to have the extra help.
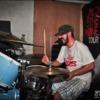
Mike Costa
Courses Plus Student 26,362 PointsIf displaying a table is all you need, try this:
echo "<table><tr><td>$row[1]</td><td>$row[2]</td><td>$row[3]</td></tr></table>";
and then style the table to your liking. That should display it in a table.
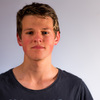
bremersharp
2,886 PointsThanks for your help I got it working.
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsChris Howell
Python Web Development Techdegree Graduate 49,702 PointsSo I went ahead and copy/pasted your code into a new blank PHP document. I put in my database connection info to connect to my local database. I replaced the query fields to match fields that exist in my database.
The query worked just fine for me and displays. Since I am unable to see the rest of the code. What you could do is set your mysql_connect() and mysql_select_db with a die() statement afterwards. inside the die() statement put a string stating something like: mysql_connect("localhost","root","root") or die("Could not connect to database"); mysql_select_db("employees") or die("Database does not exist");
if you dont set something like this up and database connection fails for whatever reason or it is unable to find the database. It wont notify you or the user what happened. If it cant connect, it will "kill" it immediately and then present the die() message string. So you know where it failed.
Im no pro with MySQL, but that is at least my understanding. Someone else might have a better way.
Your code worked my database only changing the connection and query strings. So I would double check those.