Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial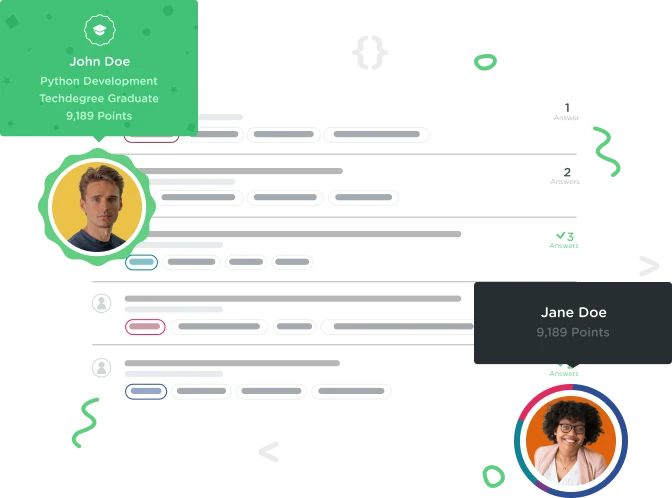

Rino Saint
5,575 PointsPHP Array Help!
Hello Treehouse-ers,
How do I make it such that the first instance of '$isbn' in the h1 element works just like the second one is in the 'li' element ?!
Thank you very much in advance!
PS bonus points if you can make me understand why the '$isbn => $book' is working because I thought you had to have the array laid out the same way [ie. 978029323 (isbn) => The Great Escape (book title) ] yet the array isn't laid out this way and yet the code I inputed in the 'li' works?!
<?php
$books["978-0743261690"] = "Gilgamesh";
$books["978-0060931957"] = "The Odyssey";
$books["978-0192840509"] = "Aesop's Fables";
$books["978-0520227040"] = "Mahabharta";
$books["978-0393320978"] = "Beowulf";
?><html>
<head>
<title>Five Great Books <?php echo " (" . $isbn . ")" ?></title>
</head>
<body>
<h1>Five Great Books</h1>
<ul>
<?php foreach($books as $isbn => $book) { ?>
<li><?php echo $book . " (" . $isbn . ")" ; ?></li>
<?php } ?>
</ul>
</body>
</html>
3 Answers

Cristian Altin
12,165 Points1) For a webpage you can only have one title at a time that will appear in the browser's tab. You wrote h1 but the line of code with $isbn is inside the head title. H1 has only the title for the book list.
2) $isbn is defined only inside the loop so you can only use it inside unless you define it elsewhere too but you'll have to access the array again with another loop or just the book you need.
3) $isbn => $book is basically telling for each iteration of the loop to read the key as $isbn and the value as $book. Since this is an array with keys as indexes, so also strings can be keys instead of classical 0,1,2,3,4,etc, you add a key and value like this: $myHashArray["myKey1"] = myValue;
Note: the $books array has strings as keys because it is wrapped in "" (and there's a "-" inside too) so it's not handled as a number

Rino Saint
5,575 PointsCristian you're a STAR!
Thank you!!
Just to make sure I understand you:
1) I am a retard for doing confusing those! Out of interest if I wanted to make what I did work, would I have to create that foreach loop thing around both the head and h1 tags or is there a more elegant way of doing that? (so the isbn is shown in brackets next to the two titles (title & h1) and is being pulled from the array by php)
2) Think that's pretty much answering 1) but I'm still a little shady as to whether there's a more elegant way of referencing the isbn once and then pulling this value from the foreach loop for use elsewhere...?
3) Thank you for clearing this up, I think I remember watching that in a prior movie - my memory failed me big time.

Cristian Altin
12,165 PointsThank you man!! XD
You're not a retard, you're a learner. Programmers are learner for life. The more you become pro the more you see that it's a whole world bigger than you can ever learn in a lifetime. So you learn bit by bit everyday knowing that you'll never stop the fun!
1) The point is that you need to decide what to have in the title. There are some useful functions to know:
echo array_search('Gilgamesh', $books); This function lets you search the associative array $books and will output the isbn
$new_array = array_keys($books); This function will create an indexed array (hence 0,1,2,3,4,...) with each $books key as value of new_array. Basically it creates a list of all the keys present in $books only that being an indexed array you can now echo new_array[2] to get the isbn of the third entry.
You can also do: echo array_keys($books)[0] to get the first isbn, [1] for the second and so on. You can use it if you don't need to have a new array for later use.
$new_array = array_values($books); Does exactly the same but instead of giving you $books keys it gives the values (the book names in your case). And yes, you can also get the third book title by doing echo array_values($books)[2]
In your code you need to decide what to do in the page's head title. If you want to output only the first book title just echo array_values($books)[0];
If you want to output all books in one line you can do this: echo join(array_values($books), ', '); where the second parameter is the separator, just a comma in this case.
For other needs keep using the foreach you already know.

Cristian Altin
12,165 PointsBTW if you want to stay DRY (Don't Repeat Yourself) and need to iterate more often creating the same list you can do this:
<?php
$books["978-0743261690"] = "Gilgamesh";
$books["978-0060931957"] = "The Odyssey";
$books["978-0192840509"] = "Aesop's Fables";
$books["978-0520227040"] = "Mahabharta";
$books["978-0393320978"] = "Beowulf";
function myfunc($value, $key){
echo "<li>" . $value . " (" . $key . ")</li>";
}
?><html>
<head>
<title>Five Great Books</title>
</head>
<body>
<h1>Five Great Books</h1>
<ul>
<?php array_walk($books, 'myfunc'); ?>
</ul>
<h1>Five Great Books...again</h1>
<ul>
<?php array_walk($books, 'myfunc'); ?>
</ul>
</body>
</html>
As you can see I replaced the whole foreach part with array_walk() which calls myfunc (remember to pass the function name as a string, it's not a mistake on my part). Then I added myfunc in the first section. Don't be puzzled, array_walk passes the two arguments for you so you don't need to care about sending them, just receive them in myfunc. You might notice that I placed the li tag inside the function to have it work as expected.
So now you can call it as many times as needed, it's DRY and the code is cleaner as you requested. Enjoy!