Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial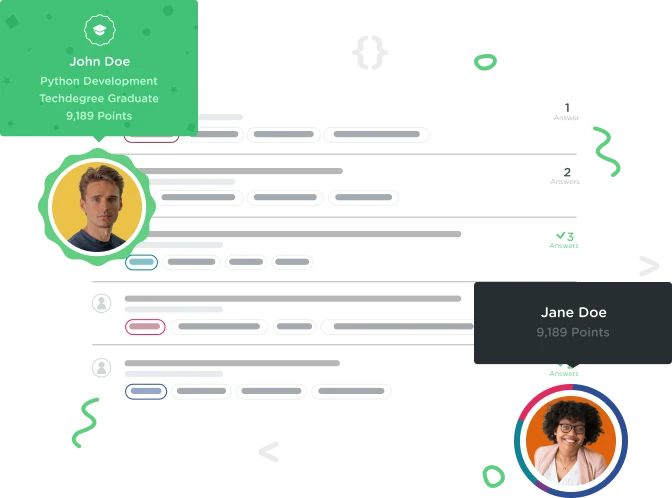
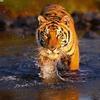
Konrad Pilch
2,435 PointsPHP Authentication Problem
Well, it kinda doesnt work:
connect.php
<?php
$dbhost = "localhost";
$dbusername = "root";
$dbpassword = "root";
$db = "youtube_guy";
$connect = mysqli_connect($dbhost, $dbusername, $dbpassword, $db) or die("Could not connect to the database");
index.php
<?php
?>
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<form action="login.php" method="POST">
<table>
<tr>
<td>Username</td>
<td><input type="text" name="username" /></td>
</tr>
<tr>
<td>Password</td>
<td><input type="password" name="password" /></td>
</tr>
<tr>
<td></td>
<td><input type="submit" name="submit" value="Login" /></td>
</tr>
</table>
</form>
</body>
</html>
login.php
<?php
SESSION_START();
// Check whether user has come from form
if(!isset($_POST['submit'])){
echo "Please log in to continue";
die("<br /><a href='index.php'>Log In </a>");
}
//Assigning variables
$username = $_POST['username'];
$passwordAttempt = $_POST['password'];
$hashPasswordAttempt = md5($passwordAttempt);
//Check forminputs
if($username == "" || $username == NULL){
echo "Please enter a username";
die("<br /><a href='index.php'>Go back </a>");
}
if($passwordAttempt == "" || $passwordAttempt == NULL){
echo "Please enter a passwordAttempt";
die("<br /><a href='index.php'>Go back </a>");
}
include ('connect.php');
$query ("SELECT * FROM users WHERE username ='$username'");
$result = mysqli_query($connect, $query);
$hits = mysqli_affected_rows($connect);
if($hits < 1){
echo "Incorrect username and password combination";
die("<br /><a href='index.php'>Go back </a>");
}
while($row = mysqli_fetch_assoc($result)){
$password = $row['password'];
if($password != $hashPasswordAttempt){
echo "Incorrect username and password combination";
die("<br /><a href='index.php'>Go back </a>");
}else(){
// All check complete session starting
$_SESSION['username'] = $username;
header("location: loggedin.php");
die("");
}
}
loggedin.php
<?php
SESSION_START();
if(!isset($_SESSION['username'])){
echo "Incorrect username and password combination";
die("<br /><a href='index.php'>Go back </a>");
}
$username = $_SESSION['username'];
echo "Hello <strong> $username </strong> you have successfully logged in.";
It doesnt work when i click the submit button.
2 Answers
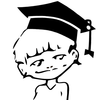
simhub
26,544 Pointshi Konrad,
first of all you should check for some error messages.
Put this on top of your login.php page
<?php
ini_set('display_errors','On');
ini_set('error_reporting',E_ALL|E_STRICT);
SESSION_START();
also on line 57 you should get rid of parentheses () :
while($row = mysqli_fetch_assoc($result)){
$password = $row['password'];
if($password != $hashPasswordAttempt){
echo "Incorrect username and password combination";
die("<br /><a href='index.php'>Go back </a>");
line 57 -> }else(){
// All check complete session starting
$_SESSION['username'] = $username;
header("location: loggedin.php");
die("");
}
after replicate your code the error message says:
Notice: Undefined variable: query in ./login.php on line 36
it might be a different message in your case.
But if not you just have to add [=] hier:
include ('connect.php');
line 36 -> $query = ("SELECT * FROM php_auth WHERE username ='$username'");
$result = mysqli_query($connect, $query);
$hits = mysqli_affected_rows($connect);
hope this helps!
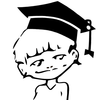
simhub
26,544 PointsBtw, what do you think to use this in a production site? of course, if i put security in it and look for better hashing system as well as some security from blog etc... ?
- Security is the most important issue if you want to work in production mode
i think the best way to do it is to learn how some modern php frameworks like laravel etc.. did it.
it’s good to understand the way they handle security issues
and as well, talking about this code, is this the modern way to do it? with OOP?
- think so?! but i believe there is no modern or old way to do it.
as long it is secure and it works.
Also, i read somewhere, but im not sure, that phpMyadmin is not used as for production? really?
- SSH tunnel might be more secure but i am not familiar with phpMyadmin
Well, SQL, SELECT * FROM etc.. this is a programming language right? it has nothing to do with phpMyadmin?
- Sql is a special-purpose programming language designed for managing data held in a relational database management system(RDBMS) -{wiki}
phpMyAdmin is a free software tool written in PHP, intended to handle the administration of MySQL over the Web.
it can be addapted to any other server or host whatever ?
- here is a nice article you should read https://www.digitalocean.com/community/tutorials/understanding-sql-and-nosql-databases-and-different-database-models
But i am sure that you will find better solutions and answers then mine so don't rely too much on my thoughs
keep up the good work ;)
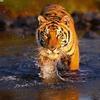
Konrad Pilch
2,435 PointsWould you say, learning laraver, would be more useful in production site? but the thing thath im learning PURE PHP , thats the best way to understand PHP and also make any other thing that framewokr might not include right? I mean, its allways better to know how CSS instead of using Bootstrap form day one right? same with PHP frameworks. ?
Well, i read or hear somewhere that the old way was kinda e.g connecting to the database like mysqli... ( localhost, root, root, database name ) ...
now is OOP and different things ? or its just database : p
I check that out :) thank you the tunel.
Oh right, i was right in my head about SQL , i even read that from internet, i got confused somehow . Good that SQL is super easy , although sometimes can be confusing, still easy :)
I try to put the code into my test production site and ill see how it goes :D i will definitely see blogs abotu security etc.. as well as somebody told me , or you wrote this , to check laraver security how it works.
Sorry, i have my head everywhere , i write all the time and read people blogs, yt, treehouse replies, and question/answers all the time i jsut odnt know to who im writing what in a way : p i jsut knwo whats writen xd lol , but im getting better, mainly because of my poor time management i have .
Thank you :)
Konrad Pilch
2,435 PointsKonrad Pilch
2,435 PointsWow. Such easy mistakes , i was about to get my head off xdd
Thank you very much for this help! And nice way to helo others :) im going to do the same, but i think maybe to ut ----- after each answer like 1. 2. maybe i dont know xd
Btw, what do you think to use this in a production site? of course, if i put security in it and look for better hashing system as well as some security from blog etc... ?
Well, meaning, if i finish my site, with login, categories, topics etc.. as a test, and then i upgrade the security, will you think it would be fair enough? and as well, talking about this code, is this the modern way to do it? with OOP?
Also, i read somewhere, but im not sure, thath phpMyadmin is not used as for production? really?
Well, SQL, SELECT * FROM etc.. this is a programming langauge right? it has nothing to do with phpMyadmin? it can be addapted to any other server or host whatever ?
Thank you very much ! This sadisfaction that the login is working its amazing :D