Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial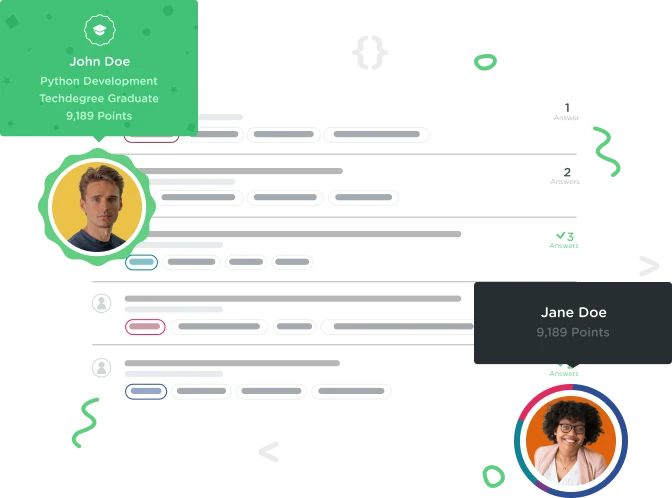

Rino Saint
5,575 PointsPHP Challenge Task 2 (and likely Task 3) Help
Hello Treehouse-ers,
Please help. I'm having trouble trying to understand exactly what the POST element etc does and what this task is asking me to do. The Challenge is under the "Build a Simple PHP Website" Stage 5 - Integrating with PayPal.
Thanks in advance!
<!DOCTYPE html>
<html>
<head>
<title>Ye Olde Ice Cream Shoppe</title>
</head>
<body>
<p>Your order has been created. What flavor of ice cream would you like to add to it?</p>
<form action="process.php" method="post">
<label for="flavor">Flavor</label>
<select id="flavor">
<option value="">— Select —</option>
<option value="Vanilla">Vanilla</option>
<option value="Chocolate">Chocolate</option>
<option value="Strawberry">Strawberry</option>
<option value="Cookie Dough">Cookie Dough</option>
</select>
<input type="submit" value="Update Order">
</form>
</body>
</html>
7 Answers
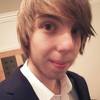
Connor Glynn
6,381 PointsHey Rino,
You need either the 'post' or 'get' attribute, on your form element, to send the information within the form to the page you declare in the 'action' attribute. These will then save all the data you send from the form, into a array called either '$_POST' or '$_GET' (depending on which one you used). This allows you to access all the data that the user entered into the form to validate it by using the 'name' attribute value you specified in each element as the key in the '$_GET' or '$_POST' array.
For example: In your code they are sending their favorite flavor to a 'process.php' file via the 'post' method. Therefore in your process.php file you could echo out this flavor using the '$_POST' array and using the 'name' attribute value on the select element as the key as shown below.
echo $_POST['flavor'];
// prints the selected flavor, from the form, on the screen
and if this was sent via the 'get' method you would replace the '$_POST' with '$_GET' to make it work.
The difference between these is a little more advanced. The overall idea is that the 'get' method will send the variables in the URL so anyone can easily see them, the 'post' method doesn't. So if you are sending sensitive data such as email, passwords etc. use the 'post' method. But if you are just sending data anyone can see use the 'get' method for easy access (and it also helps with debugging as you can see the values of the variables in the URL instead of echoing them all the time).
You can read some more about it here
Hope that helps! :)
Connor

Rino Saint
5,575 PointsFirst of all Connor - You're a LEGEND!
Thank you!
Next let me just make sure I understand you. So POST sends the info to an array located where I say after 'action'. Then from the drop down once the user hits submit the answer will be stored here ready for me to echo out when required?
I'm still stumped as to which 2 HTML elements of the code I'm supposed to be changing...
I'm assuming I should add a name value with "flavor" after the id value on the <select> tag?? Something like:
<select id="flavor" name="flavor">
Maybe I'm also supposed to add a $_POST['flavor'] somewhere then??
Please help Connor :S
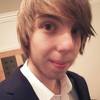
Connor Glynn
6,381 PointsAhh yes, sorry. The key for the array comes form the 'name' attribute on the element not the ID attribute. (So the ID on the select element means nothing for what we want to do, it's just there to trick us ;) ).
The task is asking us to be able to access the value of the flavor using the 'post' array and use a key of flavor.
To accomplish this, we set the method to 'post' on the form element. This will save the data in the '$_POST' array.
Next we need to give the select element a NAME of flavor for the key (Not ID). So:
<select id="flavor" name="flavor">
</select>
This will then allow us to access the value of the select by using the key 'flavor'.
Sorry about that, I confused myself a little xD
So just to make sure its the 'name' attribute that's used for the key not the ID like i said before! :) (I'll change my answer so it's right, so I don't confuzzle anyone else)
Hope that helps more! ;P
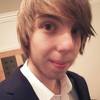
Connor Glynn
6,381 PointsYou seem to understand everything though! :D

Rino Saint
5,575 PointsYay talk about team effort Connor!
Finally finished that quiz. Thank you very much for your help - greatly appreciated :D

Rino Saint
5,575 PointsPs apparently with Question 2 I only had to change that ONE HTML tag, not TWO like it states in the question which really confused me.
Also not too sure how I label your answer as the best answer aside from the one I've already done so far. Kudos regardless Connor!
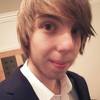
Connor Glynn
6,381 PointsYou're very welcome! :) Happy to help out a fellow developer any day ;P

Rino Saint
5,575 PointsHey Hector,
Not sure what you put in the 'form' tag of your code as it's not visible (should be method=$post etc). But yes adding name="flavor" looks right to me??
Connor's a gun and likely knows more about a possible error than I would by about a factor of one million.
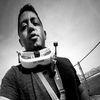
hector villasano
12,937 PointsThanks =]
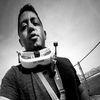
hector villasano
12,937 PointsWouldnt this be correct <form method="POST" action="process.php">
<label for="flavor">Flavor</label>
<select id="flavor" name="flavor">
<option value="">— Select —</option>
<option value="Vanilla">Vanilla</option>
<option value="Chocolate">Chocolate</option>
<option value="Strawberry">Strawberry</option>
<option value="Cookie Dough">Cookie Dough</option>
</select>
<input type="submit" value="Update Order" >
</form>
i get an error: It looks like you have added the right attribute (method) to the right element (the form), but it has the wrong value. Hint: we need to set the request method so that values are accessible in the $_POST array.
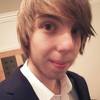
Connor Glynn
6,381 PointsAll your code there seems to be correct, just make sure you're form element looks like this
<form action="process.php" method="post">
and that should work :)
The action will send it to the process.php file (where you can add form validation etc.) The method saves it in the $_POST array (which it's telling you it's using in the question). Hope that helps! :)
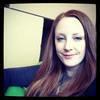
Becky Steele
16,229 PointsA heads up to those of you who have added method="post" to the form element and name="flavor" to the select element...
If you're still getting the error despite this (like I was), I recommend leaving the exercise and coming back in. Add the method="post" to form first, and then go down to select and add the name="flavor" attribute. Couldn't get mine to take otherwise.
Rino Saint
5,575 PointsRino Saint
5,575 PointsChallenge Task 2 of 3
Next, we need the flavor selected in the dropdown to be submitted to this process.php file. We need to be able to access it in the “flavor” element of the $_POST array, like this: $_POST["flavor"]. What two changes do we need to make to the HTML? (Hint. We need to add two new attributes. The first needs to be added to one existing HTML element, the second needs to be added to a different existing HTML element.)
https://teamtreehouse.com/library/build-a-simple-php-application/integrating-with-paypal/html-forms