Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial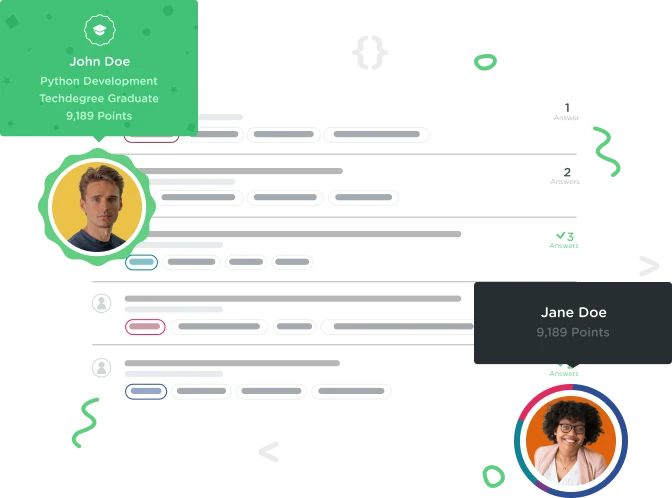
Tiffany McAllister
25,806 PointsPHP Form Issues
I am building a one-page portfolio website using Bootstrap and have a simple contact form, however, it does not work when testing in XAMPP. I know it won't actually send the email, but after I submit the form it just refreshes the page and does not display any error/success messages.
I have pasted the relevant code below. If anyone can help me work out why it isn't working, I'd really appreciate it!
<?php
if(isset($_POST['submit'])){
$to = "tiffany@tmcallister.net";
$subject = "Contact form submission from $name";
$name = $_POST['name'];
$email = $_POST['email'];
$message = $_POST['message'];
if ($name == "" || $email == "" || $message == ""){
$error_blank = "<p>One or more required fields have been left blank. Please try again.</p>";
}
elseif (!filter_var($email, FILTER_VALIDATE_EMAIL)){
$error_email = "<p>The email address you entered is not valid. Please try again.</p>";
}
elseif (mail($to, $subject, $message, "From: $name\n")) {
$success = "<p>Thanks for the message! I will get back to you as soon as possible.</p>";
}
else {
$error = "<p>Something went wrong and the form was not submitted. Please try again.</p>";
}
}
?>
<!-- CONTACT SECTION -->
<section id="contact">
<div class="container">
<h2>Contact</h2>
<?php if(isset($error_blank)){
echo $error_blank;
}
elseif (isset($error_email)){
echo $error_email;
}
elseif (isset($success)){
echo $success;
}
elseif (isset($error)){
echo $error;
}
?>
<form role="form" action="<?php htmlentities($_SERVER['PHP_SELF']); ?>" method="post">
<div class="form-group">
<label for="name">Name:</label><span class="label label-danger"><span class="icon-warning"></span> This is a required field.</span>
<input class="form-control" id="name" type="text" name="name" value="<?php if (isset($name)) { echo htmlspecialchars($name); } ?>" placeholder="Type your name">
</div>
<div class="form-group">
<label for="email">Email:</label><span class="label label-danger"><span class="icon-warning"></span> This is a required field.</span>
<input class="form-control" id="email" type="email" name="email" value="<?php if (isset($email)) { echo htmlspecialchars($email); } ?>" placeholder="Type your email address">
</div>
<div class="form-group">
<label for="message">Message:</label><span class="label label-danger"><span class="icon-warning"></span> This is a required field.</span>
<textarea rows="5" class="form-control" id="message" name="message" placeholder="Type your message"><?php if (isset($message)) { echo htmlspecialchars($message); } ?></textarea>
</div>
<input type="submit" class="btn btn-default" value="Send Message">
</form>
<div class="top-arrow">
<a href="#page-top" title="Back to top">
<span class="icon-up-arrow">
<span class="sr-only">Back to top</span>
</span>
</a>
</div>
</div>
</section>
3 Answers
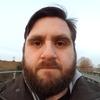
Paul Johnson
18,924 PointsYou haven't named you button "submit" so isset($_POST['submit']) is always false.
<input type="submit" class="btn btn-default" name="submit" value="Send Message">
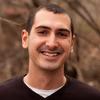
tihomirvelev
14,109 PointsI saw one existing problem and one potential problem. When I corrected them, the code worked fine on my XAMPP.
The first problem is that the submit button don't have "name=" parameter, so the if(isset($_POST['submit']))
can't receive the input properly and don't know if it's set.
Do it like this: <input type="submit" class="btn btn-default" name="submit" value="Send Message">
You might encounter a second problem and that is in the second elseif statement. The code there is properly written right now but If your php.ini file is not properly configured and there is not included a sendmail_path, the mail() function won't return either true or false.
In that case the condition will be passed and the program will always go to the else statement.
So if you encounter the second problem, try to find information how to set your php.ini and sendmail_path. Here is something to start: http://stackoverflow.com/questions/5832487/php-mail-function-doesnt-work
Hope that helps. Good luck!
PS: Sorry. I didn't saw Paul Johnson's comment. :)
Tiffany McAllister
25,806 PointsAhhh, I knew it was going to be something silly like that haha. Thanks so much guys! An extra set of eyes always helps :)
Paul Johnson
18,924 PointsPaul Johnson
18,924 PointsIf you use $_SERVER["REQUEST_METHOD"] === "POST" instead of isset you wouldn't need the name attribute on the button.