Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial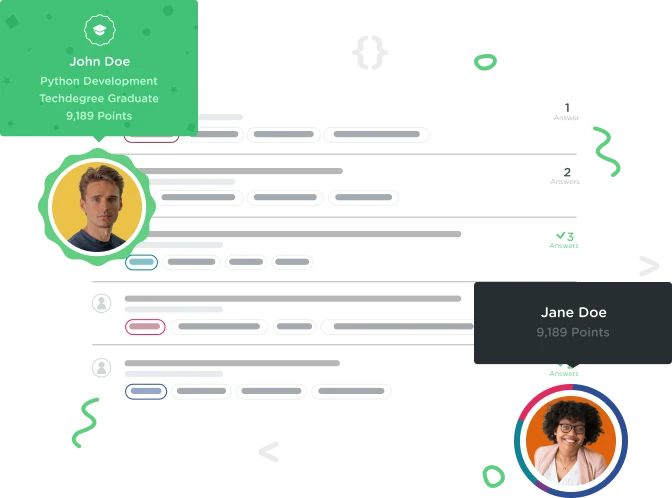
Mark Cabangon
3,940 PointsPHP form not working...
I had built a form with bootstrap v3 framework and implemented php validation. It is not working...not sending email and not showing up my "success" or "fail" message after submit is pressed. Should I post my code or the address: http://www.nalldirections.com/contact.php
6 Answers

LaVaughn Haynes
12,397 PointsYou have quite a few problems. First of all you need to specify your form method as POST (on your form) since you are checking $_POST in your PHP. Second of all you need to provide a name attribute on your form inputs (submit, email, message, name). The only one you supplied is "human". I don't think you ever make it into your first if block. You can try echoing out strings in blocks to verify that you are actually entering them as you are developing your code. Anyway, those fixes should get you to the mail block although I'm not sure your mail will actually be sent. I never specified my headers the way you do with $from so I don't know if that would work. I usually did something like this:
$headers = 'MIME-Version: 1.0' . "\r\n";
$headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n";
$headers .= "From: me@mydomain.com\r\n";
$headers .= "Reply-To: me@mydomain.com\r\n";
$headers .= "X-Mailer: PHP \r\n";
if( mail($to, $subject, $message, $headers) ){
...do something
}else{
...do something else
}
Plus on my server my domain in my from address has to match my actual domain (it's an anti spam thing I believe) so you may have to use an @nalldirections.com address. Good luck.
Mark Cabangon
3,940 PointsThanks, I was following a tutorial and this is what was provided. I will work on it and update if I am able to get it to work. Thanks for taking the time to look. I'll let you guys know, Mark C

LaVaughn Haynes
12,397 Pointsno problem. I actually did make the changes so I know it works. I'm not sending the form though, just printing out what would be sent. http://www.bonvon.com/treehouse/phpform/
Mark Cabangon
3,940 PointsLaVaughn... It works! I've sent a couple emails and it sent. Now, there is one more thing that has occurred with each submitted form. Each form sent got emailed twice. Is it because I have an "submit" as an id and a type?

LaVaughn Haynes
12,397 PointsThat sounds more like a double-submission issue. I think that's common. There are multiple ways to approach that (JavaScript, redirects, cookies, sessions, database). This post might give you some ideas. http://technoesis.net/prevent-double-form-submission/ Think about what might work best for your site. for example, if you chose JavaScript, I personally don't like the idea of disabling submit because what if there is a legit reason for submitting again? You could maybe disable it for 3 seconds only Or disable and redirect, but if they don't have js enabled they can still double submit anyway. Is that acceptable? And so on and so on.
Mark Cabangon
3,940 PointsI'm not very familiar with php, but looking at the code. Does the second line:
if ($_POST["submit"]) {
and:
if (mail ($to, $subject, $body, $from)) {
-toward the bottom both tell the form to send the information? I'm trying to understand the code by reading the actions, but not enough knowledge to understand completely.
Does anyone think that might be the cause of the form being submitted twice?
Mark Cabangon
3,940 PointsMark Cabangon
3,940 Points