Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial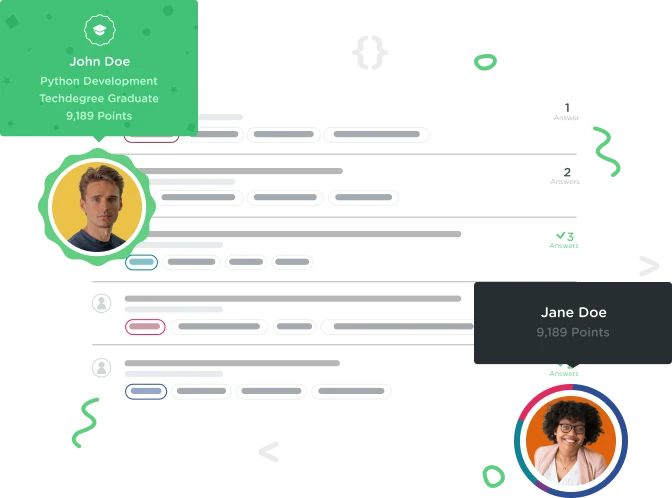

Simon Clay
3,999 PointsPHP Functions: Why is it better to return a variable than echo the results?
Randy's 'Build a Simple PHP Application' is great. I've learned so much from it.
With regard to the Functions section, I wonder why it's better to return an variable rather than echo the results within the function?
I have tried to apply the same technique to my own sample code below. My code works as seen here, but when I try to return a variable instead of echoing the results it outputs nothing to the screen.
Can anyone shed any light or improve my code below?
Many thanks.
<?php
$products = array();
$products["1940s-1950s"] = array(
"name" => "1940's - 1950's",
"type" => "Club Issues",
"listing" => "1940s-1950s.htm",
"section" => "1"
);
$products["arsenal"] = array(
"name" => "Arsenal",
"type" => "Football Club",
"listing" => "aresenal.htm",
"section" => "1"
);
$products["aldershot"] = array(
"name" => "Aldershot",
"type" => "Football Club",
"listing" => "aldershot.htm",
"section" => "1"
);
$products["current_season"] = array(
"name" => "Current Season Programmes (2014/2015)",
"type" => "",
"listing" => "2014-15season.htm",
"section" => "2"
);
function getListView($products, $section, $type) {
echo "<ul>";
foreach ($products as $product_id => $product) {
if (($product["section"] == $section) && ($product["type"] == $type)) {
echo "<li>";
echo '<a href="?s=' . $product_id . '">';
echo($product["name"]);
echo "</a>, </li>";
}
}
echo "</ul>";
}
?>
<h2>Section 1</h2>
<h3>Club Issues</h3>
<?php getListView($products,"1","Club Issues") ?>
<h3>Selected Clubs</h3>
<?php getListView($products,"1","Football Club") ?>
<h2>Section 2</h2>
<?php getListView($products,"2","") ?>
2 Answers
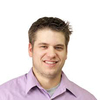
Kevin Korte
28,149 PointsThis code would work
<?php
$products = array();
$products["1940s-1950s"] = array(
"name" => "1940's - 1950's",
"type" => "Club Issues",
"listing" => "1940s-1950s.htm",
"section" => "1"
);
$products["arsenal"] = array(
"name" => "Arsenal",
"type" => "Football Club",
"listing" => "aresenal.htm",
"section" => "1"
);
$products["aldershot"] = array(
"name" => "Aldershot",
"type" => "Football Club",
"listing" => "aldershot.htm",
"section" => "1"
);
$products["current_season"] = array(
"name" => "Current Season Programmes (2014/2015)",
"type" => "",
"listing" => "2014-15season.htm",
"section" => "2"
);
function getListView($products, $section, $type) {
$html = "<ul>";
foreach ($products as $product_id => $product) {
if (($product["section"] == $section) && ($product["type"] == $type)) {
$html.= "<li>";
$html.= '<a href="?s=' . $product_id . '">';
$html.= ($product["name"]);
$html.= "</a>, </li>";
}
}
$html.= "</ul>";
return $html;
}
?>
<h2>Section 1</h2>
<h3>Club Issues</h3>
<?php echo getListView($products,"1","Club Issues") ?>
<h3>Selected Clubs</h3>
<?php echo getListView($products,"1","Football Club") ?>
<h2>Section 2</h2>
<?php echo getListView($products,"2","") ?>
And really the reason why that I can think of is separation of concerns. You keep you logic code from producing any view able content, and you call that logically code from the views, and show it's results. Small applications, this isn't as necessary, which is why it's valid to ask. You could see how though if this site scaled, this could be a problem if you had some functions that had echo's in them, and some functions that returned, it would be hard to keep straight.
Wordpress deals with this by the function name, which is one option. For instance, the_permalink()
will echo the URL from the function. get_the_permalink()
will only return it. You can see by the name of the function that would make sense.

Simon Clay
3,999 PointsThat is great Kevin, thank you!
I like the $html.=
I guess that's a shorthand way of writing $html = $html . "<li> etc";
?
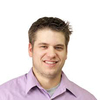
Kevin Korte
28,149 PointsYes sir, each line keeps building that html variable. It's a common convention used when a function is building html. I think I originally stole that idea from Wordpress, but I've seen it used elsewhere too. It's handy and familiar.