Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial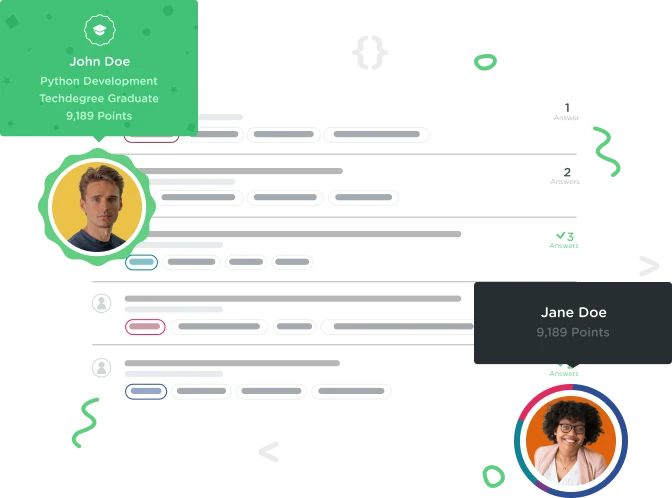

Chris Nelson
4,978 PointsPHP opening and closing brackets - 2 ways to do it?
I learned that PHP statements start and end with brackets and question marks :
<?php ... ?>
On this video, however, - http://teamtreehouse.com/library/build-a-simple-php-application/adding-a-contact-form/working-with-get-variables -
I noticed that Randy used both opening and closing brackets in the beginning, and opening and closing brackets at the end of the php statement. The video didn't elaborate on why he was doing that. So, for example, Randy entered:
<?php if (isset($_GET["status"]) AND $_GET["status"] == "thanks") { ?>
<p>Thanks for the email! I’ll be in touch shortly!</p>
<?php } else { ?>
....
<?php } ?>
Is that way a variation from coding it this way? :
<?php if (isset($_GET["status"]) AND $_GET["status"] == "thanks") { <p>Thanks for the email! I’ll be in touch shortly!</p> } else { .... } ?>
What's the difference between these two methods? When and Why do we need to code it "Randy's way" ?
thanks in advance.
6 Answers
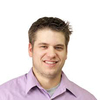
Kevin Korte
28,148 PointsBecause you can't put HTML inside of php tags unless it's being printed to the browser as a string. That's fine for simple html bits, for example maybe your're generating list items from a PHP loop, than you can just echo "<ul class='mylist'>";
and continue on your looping.
But if you're doing bigger blocks of html code, it's more readable to open and close the tags when needed. Keep in mind the server will only look at what's inside the PHP tags before it sends it to the browser.

Dustin Matlock
33,856 PointsSome say it's for readability and good practice, but on a slightly similar note <?php
doesn't always need ?>
at the end unless it's within an HTML file. Pure PHP files (server-side scripts) do not need opening and closing tags, since in HTML, the tags are needed show where PHP starts and stops. However, there are more possible explanations which some might argue for.
Closing Brackets and PHP Tags

andi mitre
Treehouse Guest TeacherI didn't watch the video but it seems that he is incorporating php with html. For example you can have an index.php file with many lines of html but you also need to include some php for data retrieval from the database (there are many more reasons why).In that case you need to open and close php tags to write your php script in which can be done numerous time on the same file. Here is a more concrete example.
<li>
<label for="Message">Message</label>
<textarea type="text" name="message" placeholder="Enter message" autocomplete="off" required="on"></textarea>
<?php include ("account.php");
$sql = "select * from registered_table where user = '$username' and pass = '$password'";
$result = mysql_query($sql) or die(mysql_error());
if (mysql_num_rows($result) != 0)
{
while ($row = mysql_fetch_array($result))
{
$chat_content = $row['chat_content'];
print "<textarea value=$chat_content>$chat_content";
print "</textarea>";
}
}
?>
</li>

Chris Nelson
4,978 PointsAre you saying it's because html is inside the php that the bracket is closed before the html and reopened after the html?
I thought it might have been because of the curly braces, but then that wasn't consistent with the first part of the file I'm working with.
Still a little unclear.

andi mitre
Treehouse Guest TeacherNo. So anytime you want to write PHP inside an html file you need to declare where your php script will start with the opening tag which is <?php and when your script ends you provide the closing tag ?> In between these tags you write the php code. the tags are there to say in this html file here's where the php starts and ends. hope that helps
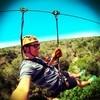
thomascawthorn
22,986 PointsHey Chris!
So I think there's a few things to mention which might help answer your question:
Everything inside php tags <?php ?> has to be php code.
so your example of:
<?php if (isset($_GET["status"]) AND $_GET["status"] == "thanks") { Thanks for the email! I’ll be in touch shortly! } else { .... } ?>
won't work because the conditional in the if statement ( Thanks for the email! I’ll be in touch shortly!) is not defined as a string (you'll need to surround with ""), nor is it echo'd to the page.
If you wanted to avoid opening and closing php tags and create the same statement in php, you could try
<?php
if (isset($_GET["status"]) AND $_GET["status"] == "thanks") {
echo " <p>Thanks for the email! I’ll be in touch shortly!</p>";
} else {
echo "<p>Something else</p>";
}
?>
I haven't tested this so it might not work straight away!
By opening and closing the php tags, you can write plain old HTML in conditional statements, functions, etc...
I think this is an advantage if you have lot's of html compared to php or maybe it runs better with your code indentation..
Maybe you're creating a string which involves concatenating (joining together) a large number of different variables and strings, which would be a lot cleaner in php:
<p>Hello <?php echo $name; ?> You are <?php echo $age; ?> years old and live at <?php echo $address; ?>.</p>
//vs
<?php
echo "<p>Hello" . $name . " You are " . $age . " years old and live at " . $address .".</p>" ;
?>
Both have the same result, it's whatever you prefer/looks cleaner I guess!

Chris Nelson
4,978 PointsThank you to all who have submitted an answer. I seem to have found the answer to my question in the video following the one I was looking at, which is:
Basically, it DOES have to do with the fact that HTML was included inside the PHP, and they need to be maintained separate.
Thanks again.

Brandon Stewart
3,434 PointsAn easy way to do if statements while in HTML around it is by doing it this way.
<?php if ($blah = '1') : ?>
<h2>BLAH</h2>
<?php else : ?>
<h1>Not BLAH</h1>
<?php endif; ?>