Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial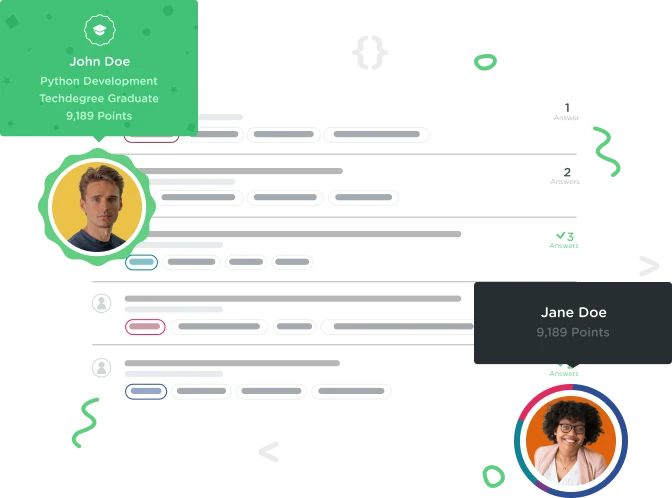
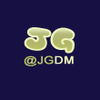
Jonathan Grieve
Treehouse Moderator 91,254 PointsPHP: Ping Pong Simulation Game
Just thought I'd share this version of the Ping Pong Game. Code is Alena's with 1 or 2 little tweaks of my own. What do you think? :)
http://www.jonniegrieve.co.uk/jg-lab.co.uk/phpbasics-2016/pingpong.php
<!doctype html>
<html>
<head>
<title>PHP: Ping Pong Simulation Game!</title>
<link href="https://fonts.googleapis.com/css?family=Roboto+Slab" rel="stylesheet">
</head>
<body>
<style>
/* Styles */
* {
margin: 0;
padding: 0;
}
body {
background: pink;
font-family: "Roboto Slab";
}
header {
width: 100%;
height: 150px;
background: blue;
color: white;
}
.pp_head {
text-align: center;
}
.content {
line-height: 1.5;
position: relative;
width: 400px;
margin: 20px auto;
background: yellow;
/*padding: 15px;*/
box-sizing: content-box;
}
div.rallies:nth-child(2n) {
background: lightgreen;
color: orangered;
}
.rallies {
padding-left: 20px;
}
footer {
text-align: center;
margin-top: 20px;
}
.logo {
position: relative;
top: 20px;
margin: 0 auto;
display: block;
width: 100px;
height: 100px;
border-radius: 100%;
}
h2.winner {
margin-top: 10px;
padding: 10px;
text-align: center;
font-size: 17pt;
text-transform: uppercase;
color: red;
}
p span {
font-weight: bold;
}
</style>
<?php
//initialise and declare variables for player and round scores.
$player1 = 0;
$player2 = 0;
$round = 0;
?>
<header>
<img class="logo" src = "logo.png" />
</header>
<div class="pp_head">
<h1>PHP Ping Pong Simulation Game</h1>
<p> Let's play!</p>
</div>
<div class = "content">
<div class="round">
<?php
//WIN
//player must reach a score of 11
//player must be a minimum of 2 point higher than opponent
//continue the game while the absolute value is less than ;
while (abs($player1 - $player2) < 2 || $player1 < 11 && $player2 < 11) {
$round++;
echo "<div class =\"rallies\">";
echo "<h2>Round $round</h2>\n";
//random scores between 1 (max) and 0 (min)
if(rand(0,1)) {
$player1++;
} else {
$player2++;
}
echo "Player1 = $player1<br />\n";
echo "Player2 = $player2<br />\n";
echo "</div>";
}
?>
</div>
<div class = "winner">
<?php
if($player1 > $player2) {
echo "<h2 class=\"winner\">Player 1";
} else {
echo "<h2 class=\"winner\">Player 2";
}
echo " is the winner after $round rounds</h2>";
?>
</div>
</div>
<footer>
<p>PHP Ping Pong Simulation Game. From Teehouse by Jonnie Grieve Digital Media</a></p>
</footer>
</body>
</html>
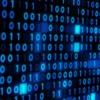
Alexander Davison
65,469 PointsGood!
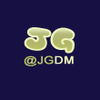
Jonathan Grieve
Treehouse Moderator 91,254 PointsThanks for your feedback

Amarria WordPress Design
9,750 PointsThanks for this.
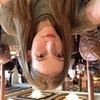
Kat Stacy
41,658 Points//continue the game while the absolute value is less than ; while (abs($player1 - $player2) < 2 || $player1 < 11 && $player2 < 11) Why do you use the logical operators where you do. In Alena's game, the score starts out with an absolute value of 5. So wouldn't the game be over if you use the || or logical operator where you do? If the absolute value needs to be greater than 2 for the loop to stop or the players must have a score of greater than 11 to win then I think the game should have ended before it began. Shouldn't the first logical operator be &&? And why is the second logical operator && shouldn't it be || since we only want one of the players to have a score greater than 11 to win? I am so confused by this lesson.
1 Answer
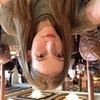
Kat Stacy
41,658 PointsI think I know the answer to my question of why the certain logical operators where used.
while (abs($player1 - $player2) < 2 || $player1 < 11 && $player2 < 11)
While either of the conditions are true then the loop will continue to run. Even thought the first condition is false (bc the abs starting value is more than 2) the second condition is true so the while loop will continue to run until both conditions are false.
casthrademosthene
6,275 Pointscasthrademosthene
6,275 PointsWow you took the game from a 1 to a 9... I love it.. You did a great job