Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial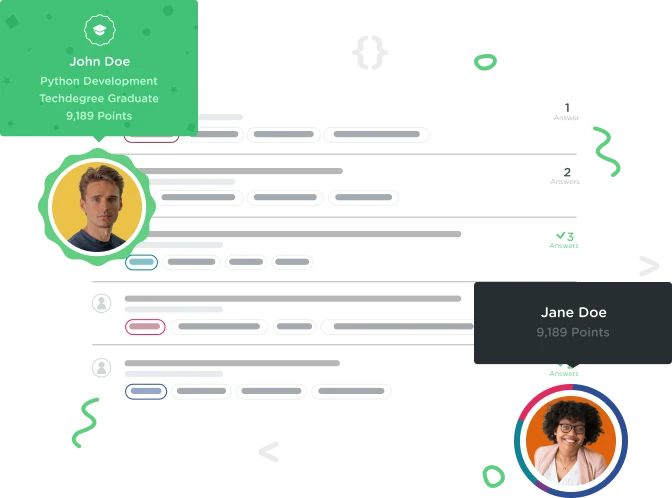

SHANTANU RANA
Courses Plus Student 524 PointsPHP Problem
Question: With this piece of code I want the user to type either name, year or state and the following array from pms.php will be displayed. I want that there is only one box in index.php and it is compatible searching all three fields however I am not sure where am i actually making a mistake.
Thanks in advance for your help.
index.html -----------------
<!DOCTYPE html>
<!-- Home page of PM database search example. -->
<html>
<head>
<title>Associative array search example</title>
<meta charset="utf-8">
<link rel="stylesheet" type="text/css" href="styles/wp.css">
</head>
<body>
<h2>Australian Prime Ministers</h2>
<h3>Query</h3>
<form method="get" action="results.php">
<table>
<tr><td>Name: </td><td><input type="text" name="query"></td></tr>
<!--
<tr><td>Year: </td><td><input type="text" name="year"></td></tr>
<tr><td>State: </td><td><input type="text" name="state"></td></tr>
-->
<tr><td colspan=1><input type="submit" value="Search">
<input type="reset" value="Reset"></td></tr>
<table>
</form>
<hr>
<p>
Source:
<a href="show.php?file=index.html">index.html</a>
</p>
</body>
</html>
results.php
<?php
/*
* Simple example to illustrate associative arrays and queries.
* DANGEROUS: Does not sanitise user input.
* BAD STYLE: Does not use templates. Interleaves PHP and HTML.
*/
include "includes/defs.php";
/* Get form data. */
$query = $_GET['query'];
/*
$year = $_GET['year'];
$state = $_GET['state'];
*/
/* Get array of pms that match query in form data. */
$pms = search($query);
?>
<!-- display results -->
<!DOCTYPE html>
<!-- Results page of associative array search example. -->
<html>
<head>
<title>Associative array search results page</title>
<meta charset="utf-8">
<link rel="stylesheet" type="text/css" href="styles/wp.css">
</head>
<body>
<h2>Australian Prime Ministers</h2>
<h3>Results</h3>
<?php
if (count($pms) == 0) {
?>
<p>No results found.</p>
<?php
} else {
?>
<table class="bordered">
<thead>
<tr><th>No.</th><th>Name</th><th>From</th><th>To</th><th>Duration</th><th>Party</th><th>State</th></tr>
</thead>
<tbody>
<?php
foreach($pms as $pm) {
print
"<tr><td>{$pm['index']}</td><td>{$pm['name']}</td><td>{$pm['from']}</td><td>{$pm['to']}</td><td>{$pm['duration']}</td><td>{$pm['party']}</td><td>{$pm['state']}</td></tr>\n";
}
?>
</tbody>
</table>
<?php
}
?>
<p><a href="index.html">New search</a></p>
<hr>
<p>
Sources:
<a href="show.php?file=results.php">results.php</a>
<a href="show.php?file=includes/defs.php">includes/defs.php</a>
<a href="show.php?file=includes/pms.php">includes/pms.php</a>
</p>
</body>
</html>
defs.php
<?php
/* Functions for PM database example. */
/* Load sample data, an array of associative arrays. */
include "pms.php";
/* Search sample data for $name or $year or $state from form. */
function search($query) {
global $pms;
// Filter $pms by $name
if (!empty($query)) {
$results = array();
foreach ($pms as $pm) {
if (stripos($pm['query'], $query) !== FALSE ||
/*
stripos($pm['from'], $query) !== FALSE ||
stripos($pm['to'], $query) !== FALSE || */
stripos($pm['state'], $query) !== FALSE)
{
$results[] = $pm;
}
}
$pms = $results;
}
/*
// Filter $pms by $year
if (!empty($year)) {
$results = array();
foreach ($pms as $pm) {
if (strpos($pm['from'], $year) !== FALSE ||
strpos($pm['to'], $year) !== FALSE) {
$results[] = $pm;
}
}
$pms = $results;
}
// Filter $pms by $state
if (!empty($state)) {
$results = array();
foreach ($pms as $pm) {
if (stripos($pm['state'], $state) !== FALSE) {
$results[] = $pm;
}
}
*/
$pms = $results;
}
return $pms;
?>
pms.php
<?php
/* Australian Prime Ministers. Data as of 5 March 2010. */
$pms = array(
array('index' => '1', 'name' => 'Edmund Barton', 'from' => '1 January 1901', 'to' => '24 September 1903', 'party' => 'Protectionist', 'duration' => '2 years, 8 months, 24 days', 'state' => 'New South Wales'),
array('index' => '2', 'name' => 'Alfred Deakin', 'from' => '24 September 1903', 'to' => '27 April 1904', 'party' => 'Protectionist', 'duration' => '0 years, 7 months, 4 days', 'state' => 'Victoria'),
array('index' => '3', 'name' => 'Chris Watson', 'from' => '27 April 1904', 'to' => '18 August 1904', 'party' => 'Labour', 'duration' => '0 years, 3 months, 21 days', 'state' => 'New South Wales'),
array('index' => '4', 'name' => 'George Reid', 'from' => '18 August 1904', 'to' => '5 July 1905', 'party' => 'Free Trade', 'duration' => '0 years, 10 months, 18 days', 'state' => 'New South Wales'),
array('index' => '-', 'name' => 'Alfred Deakin', 'from' => '5 July 1905', 'to' => '13 November 1908', 'party' => 'Protectionist', 'duration' => '3 years, 4 months, 9 days', 'state' => 'Victoria'),
array('index' => '5', 'name' => 'Andrew Fisher', 'from' => '13 November 1908', 'to' => '2 June 1909', 'party' => 'Labour', 'duration' => '0 years, 6 months, 21 days', 'state' => 'Queensland'),
array('index' => '-', 'name' => 'Alfred Deakin', 'from' => '2 June 1909', 'to' => '29 April 1910', 'party' => 'Commonwealth Liberal', 'duration' => '0 years, 10 months, 28 days', 'state' => 'Victoria'),
array('index' => '-', 'name' => 'Andrew Fisher', 'from' => '29 April 1910', 'to' => '24 June 1913', 'party' => 'Labor', 'duration' => '3 years, 1 month, 26 days', 'state' => 'Queensland'),
array('index' => '6', 'name' => 'Joseph Cook', 'from' => '24 June 1913', 'to' => '17 September 1914', 'party' => 'Commonwealth Liberal', 'duration' => '1 year, 2 months, 25 days', 'state' => 'New South Wales'),
array('index' => '-', 'name' => 'Andrew Fisher', 'from' => '17 September 1914', 'to' => '27 October 1915', 'party' => 'Labor', 'duration' => '1 year, 1 month, 11 days', 'state' => 'Queensland'),
array('index' => '7', 'name' => 'Billy Hughes', 'from' => '27 October 1915', 'to' => '9 February 1923', 'party' => 'Labor/Nationalist', 'duration' => '7 years, 3 months, 14 days', 'state' => 'New South Wales, Victoria'),
array('index' => '8', 'name' => 'Stanley Bruce', 'from' => '9 February 1923', 'to' => '22 October 1929', 'party' => 'Nationalist', 'duration' => '6 years, 8 months, 14 days', 'state' => 'Victoria'),
array('index' => '9', 'name' => 'James Scullin', 'from' => '22 October 1929', 'to' => '6 January 1932', 'party' => 'Labor', 'duration' => '2 years, 2 months, 16 days', 'state' => 'Victoria'),
array('index' => '10', 'name' => 'Joseph Lyons', 'from' => '6 January 1932', 'to' => '7 April 1939', 'party' => 'United Australia', 'duration' => '7 years, 3 months, 2 days', 'state' => 'Tasmania'),
array('index' => '11', 'name' => 'Earle Page', 'from' => '7 April 1939', 'to' => '26 April 1939', 'party' => 'Country', 'duration' => '0 years, 0 months, 20 days', 'state' => 'New South Wales'),
array('index' => '12', 'name' => 'Robert Menzies', 'from' => '26 April 1939', 'to' => '28 August 1941', 'party' => 'United Australia', 'duration' => '2 years, 4 months, 4 days', 'state' => 'Victoria'),
array('index' => '13', 'name' => 'Arthur Fadden', 'from' => '28 August 1941', 'to' => '7 October 1941', 'party' => 'Country', 'duration' => '0 years, 1 month, 9 days', 'state' => 'Queensland'),
array('index' => '14', 'name' => 'John Curtin', 'from' => '7 October 1941', 'to' => '5 July 1945', 'party' => 'Labor', 'duration' => '3 years, 8 months, 29 days', 'state' => 'Western Australia'),
array('index' => '15', 'name' => 'Frank Forde', 'from' => '6 July 1945', 'to' => '13 July 1945', 'party' => 'Labor', 'duration' => '0 years, 0 months, 8 days', 'state' => 'Queensland'),
array('index' => '16', 'name' => 'Ben Chifley', 'from' => '13 July 1945', 'to' => '19 December 1949', 'party' => 'Labor', 'duration' => '4 years, 5 months, 7 days', 'state' => 'New South Wales'),
array('index' => '-', 'name' => 'Robert Menzies', 'from' => '19 December 1949', 'to' => '26 January 1966', 'party' => 'Liberal', 'duration' => '16 years, 1 month, 8 days', 'state' => 'Victoria'),
array('index' => '17', 'name' => 'Harold Holt', 'from' => '26 January 1966', 'to' => '19 December 1967[5]', 'party' => 'Liberal', 'duration' => '1 year, 10 months, 23 days', 'state' => 'Victoria'),
array('index' => '18', 'name' => 'John McEwen', 'from' => '19 December 1967', 'to' => '10 January 1968', 'party' => 'Country', 'duration' => '0 years, 0 months, 23 days', 'state' => 'Victoria'),
array('index' => '19', 'name' => 'John Gorton', 'from' => '10 January 1968', 'to' => '10 March 1971', 'party' => 'Liberal', 'duration' => '3 years, 2 months, 0 days', 'state' => 'Victoria'),
array('index' => '20', 'name' => 'William McMahon', 'from' => '10 March 1971', 'to' => '5 December 1972', 'party' => 'Liberal', 'duration' => '1 year, 8 months, 25 days', 'state' => 'New South Wales'),
array('index' => '21', 'name' => 'Gough Whitlam', 'from' => '5 December 1972', 'to' => '11 November 1975', 'party' => 'Labor', 'duration' => '2 years, 11 months, 7 days', 'state' => 'New South Wales'),
array('index' => '22', 'name' => 'Malcolm Fraser', 'from' => '11 November 1975', 'to' => '11 March 1983', 'party' => 'Liberal', 'duration' => '7 years, 4 months, 0 days', 'state' => 'Victoria'),
array('index' => '23', 'name' => 'Bob Hawke', 'from' => '11 March 1983', 'to' => '20 December 1991', 'party' => 'Labor', 'duration' => '8 years, 9 months, 10 days', 'state' => 'Victoria'),
array('index' => '24', 'name' => 'Paul Keating', 'from' => '20 December 1991', 'to' => '11 March 1996', 'party' => 'Labor', 'duration' => '4 years, 2 months, 20 days', 'state' => 'New South Wales'),
array('index' => '25', 'name' => 'John Howard', 'from' => '11 March 1996', 'to' => '3 December 2007', 'party' => 'Liberal', 'duration' => '11 years, 8 months, 23 days', 'state' => 'New South Wales'),
array('index' => '26', 'name' => 'Kevin Rudd', 'from' => '3 December 2007', 'to' => '24 June 2010', 'party' => 'Labor', 'duration' => '2 years, 6 months, 21 days', 'state' => ' Queensland'),
array('index' => '27', 'name' => 'Julia Gillard', 'from' => '24 June 2010', 'to' => 'Incumbent', 'party' => 'Labor', 'duration' => '2 years, 8 months, 6 days', 'state' => ' Victoria')
);
?>