Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial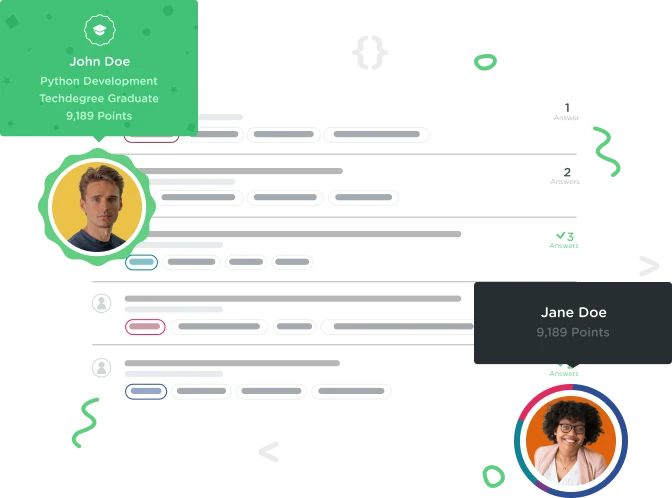

Daniel Silva
5,353 PointsPHP Redirect
I'm trying to redirect to a new page once the user logs in with the proper credentials. However, when I click login it doesn't do anything.
This page is where the username and password are checked through the database.
<?php
session_start();
require_once '../DatabaseConnection/DatabaseConnection.php';//check conn file
$date = date("format", $timestamp);
$_SESSION['LoginDate']= $date;
$myusername = $_POST['username'];
$mypassword = $_POST['password'];
$myusername = stripslashes($myusername);
$mypassword = stripslashes($mypassword);
$myusername = mysqli_real_escape_string($conn, $myusername);
$mypassword = mysqli_real_escape_string($conn, $mypassword);
$Hashed = hash("ripemd128", $mypassword);
$sql = "SELECT username, password FROM user_table WHERE username ='".$myusername."' and password ='".$Hashed."'";
$loginresult = $conn->query($sql);
if(!$loginresult){
$message = "Whole query". $sql;
echo $message;
die('Invalid query' . mysqli_error());
}
$count = $loginresult->num_rows;
if($count ==1){
header("Location: stats.php");
$_SESSION['username']=$myusername;
$_SESSION['password']=$mypassword;
}else{
header('Location:login.php');
$_SESSION['badPass']=1;
echo "Error";
}
mysqli_close($conn);
This page is where the user fills logs in.
<?php
session_start();
require_once('../DatabaseConnection/DatabaseConnection.php');
if(isset($_POST) & !empty($_POST)){
$username = mysqli_real_escape_string($conn, $_POST['username']);
$password = md5($_POST['password']);
$sql = "SELECT * FROM `user_table` WHERE username='$username' AND password='$password'";
$result = mysqli_query($conn, $sql);
$count = mysqli_num_rows($result);
if($count == 1){
$_SESSION['username'] = $username;
}else{
$fmsg = "Invalid Username/Password";
}
}
if(isset($_SESSION['username'])){
$smsg = "User already logged in";
}
?>
<!DOCTYPE html>
<html>
<head>
<title>User Login in PHP & MySQL</title>
<!-- Latest compiled and minified CSS -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" >
<!-- Latest compiled and minified JavaScript -->
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js" ></script>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<div class="container">
<?php if(isset($smsg)){ ?><div class="alert alert-success" role="alert"> <?php echo $smsg; ?> </div><?php } ?>
<?php if(isset($fmsg)){ ?><div class="alert alert-danger" role="alert"> <?php echo $fmsg; ?> </div><?php } ?>
<form class="form-signin" method="POST" action="loginVerify.php">
<h2 class="form-signin-heading">Please Register</h2>
<div class="input-group">
<span class="input-group-addon" id="basic-addon1">@</span>
<input type="text" name="username" class="form-control" placeholder="Username" required>
</div>
<label for="inputPassword" class="sr-only">Password</label>
<input type="password" name="password" id="inputPassword" class="form-control" placeholder="Password" required>
<button class="btn btn-lg btn-primary btn-block" type="submit">Login</button>
<a class="btn btn-lg btn-primary btn-block" href="newUser.php">Register</a>
</form>
</div>
</body>
</html>
Any help would be appreciated. I know the path to the new file it's supposed to direct to is good so it's not a file path issue.
Daniel Bell
17,178 PointsDaniel Bell
17,178 PointsYou have this line in the second snippet:
if(isset($_POST) & !empty($_POST)){
You want && there, not &.