Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial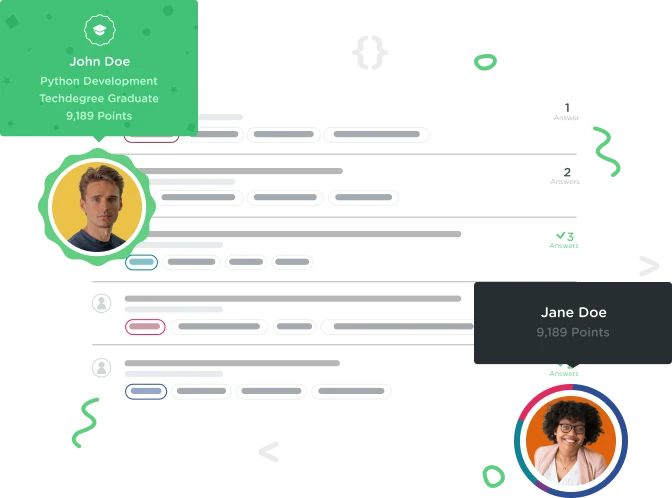
Trevor Wood
17,828 PointsPHP Signing in a user help
The form says that it signs in the user, however it is not actually signing the user in. Anybody know what I'm doing wrong here?
<?php
$pageTitle = "Sign In";
$pageCategory = "Sign In";
$pageCategoryurl = "/signin.php";
//signup.php
include($_SERVER["DOCUMENT_ROOT"] . "/inc/header.php");
include($_SERVER["DOCUMENT_ROOT"] . "/inc/search.php");
?>
<div class="content">
<div id="signinheader"><h2>Sign in</h2></div><div style="clear:both"></div>
<?php
if(isset($_SESSION['signed_in']) && $_SESSION['signed_in'] == true)
{
echo 'You are already signed in, you can <a href="signout.php">sign out</a> if you want.';
}
else
{
if($_SERVER['REQUEST_METHOD'] != 'POST')
{
/*the form hasn't been posted yet, display it
note that the action="" will cause the form to post to the same page it is on */
echo '<form method="post" action="">
<table>
<tr>
<th><label for="username" class="signinlabel">Username:</label></th>
<td><input type="text" name="username" class="signininput"></td>
</tr>
<tr>
<th><label for="userpass" class="signinlabel">Password:</label></th>
<td><input type="password" name="userpass" class="signininput"></td>
</tr>
</table>
<input type="submit" value="Sign In" class="signinbutton">
</form>';
}
else
{
/* so, the form has been posted, we'll process the data in three steps:
1. Check the data
2. Let the user refill the wrong fields (if necessary)
3. Save the data
*/
$errors = array(); /* declare the array for later use */
if(!isset($_POST['username']) OR empty($_POST['username']))
{
$errors[] = 'The username field must not be empty.';
}
if(!isset($_POST['userpass']) OR empty($_POST['userpass']))
{
$errors[] = 'The password field must not be empty.';
}
if(!empty($errors)) /*check for an empty array, if there are errors, they're in this array (note the ! operator)*/
{
echo '<div id="signinerror"><h3>Uh-oh.. a couple of fields are not filled in correctly..</h3>';
echo '<ul>';
foreach($errors as $key => $value) /* walk through the array so all the errors get displayed */
{
echo '<li class="signinerrorli">' . $value . '</li>'; /* this generates a nice error list */
}
echo '</ul></div><div style="clear:both"></div>';
}
else
{
//the form has been posted without, so save it
//notice the use of mysqli_real_escape_string, keep everything safe!
//also notice the sha1 function which hashes the password
$username = $_POST['username'];
$userpass = sha1($_POST['userpass']);
$result = mysqli_query($con,"SELECT * FROM users
WHERE username = '$username' AND userpass = '$userpass'");
if(!$result)
{
//something went wrong, display the error
echo 'Something went wrong while signing in. Please try again later.';
//echo mysqli_error(); //debugging purposes, uncomment when needed
}
else
{
//the query was successfully executed, there are 2 possibilities
//1. the query returned data, the user can be signed in
//2. the query returned an empty result set, the credentials were wrong
if(mysqli_num_rows($result) == 0)
{
echo 'You have supplied a wrong user/password combination. Please try again.';
}
else
{
$_SESSION['signed_in'] = true;
//we also put the user_id and user_name values in the $_SESSION, so we can use it at various pages
while($row = mysqli_fetch_assoc($result))
{
$_SESSION['user_id'] = $row['user_id'];
$_SESSION['username'] = $row['username'];
$_SESSION['useremail'] = $row['useremail'];
}
echo 'Welcome, ' . $_SESSION['username'] . '. <a href="/home.php">Proceed to the homepage</a>.';
}
}
}
}
}
?>
</div>
<?php
include($_SERVER["DOCUMENT_ROOT"] . "/inc/footer.php");
?>
1 Answer

dhillonsh
4,745 Points<?php
session_start();
$pageTitle = "Sign In";
You need to initiate the start of the session at the top of the page.