Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial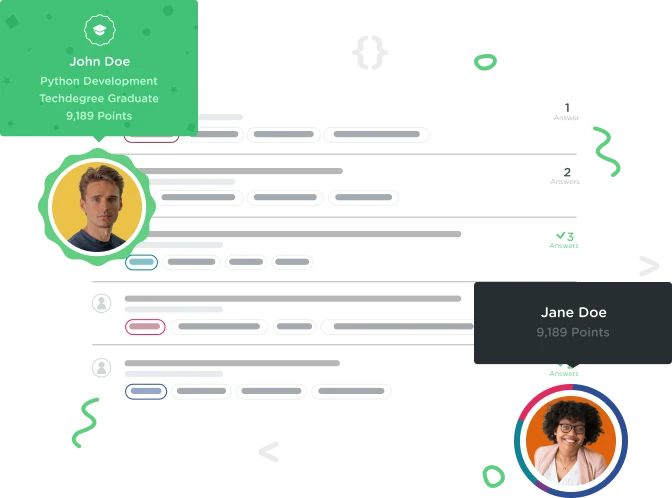

Seif Eldein Sayed
3,508 PointsPhp sorting problem
I want to sort a list of products by size, brand or price but I don't know how to achieve this in php. <?php
function get_list_view_html($product_id, $product) {
$output = "";
$output = $output . "<li>";
$output = $output . '<a href="shirt.php?id=' . $product_id . '">';
$output = $output . '<img src="' . $product["img"] . '" alt="' . $product["name"] . '">';
$output = $output . "<p>View Details</p>";
$output = $output . "</a>";
$output = $output . "</li>";
return $output;
} ?>
<?php
function get_table_data()
{
include("inc/connectionfile.php");
$result = mysql_query("SELECT * FROM product");
while($row = mysql_fetch_array($result))
{
echo get_list_view_html($row['id'],$row);
}
}
?>
I call get_table_data on the page
2 Answers

Randy Hoyt
Treehouse Guest TeacherIf you want to sort in PHP with code like this, you'll need to get the data out of MySQL first, sort the resulting array, and then loop through them and display them. Here's the simplest change:
<?php
function get_table_data()
{
include("inc/connectionfile.php");
$result = mysql_query("SELECT * FROM product");
$products = array();
while($row = mysql_fetch_array($result))
{
$products[] = $row;
}
// re-order the array elements
foreach ($products as $product) {
echo get_list_view_html($product['id'],$product);
}
}
?>
There are a LOT of other changes I would make to this: I would use PDO instead of these mysql_
functions, I would order the results directly in the SQL query, etc. We'll be looking at moving the products to a database table and covering these items in future videos.
Does that help?

Seif Eldein Sayed
3,508 PointsYes, this is what I did, I have the products now in database, but now I don't want to only show them. I want to sort them according to price for example. and another issue that I'm facing is that I will make a "back-end " for shirts4mike where designers can Add shirts that they created but I don't know how can I dynamically add them to Paypal cart.
Future videos about this method would be awesome ofcourse. Thanks for helping me anyway. Can't believe how stupid I am not thinking in putting them in an array xD